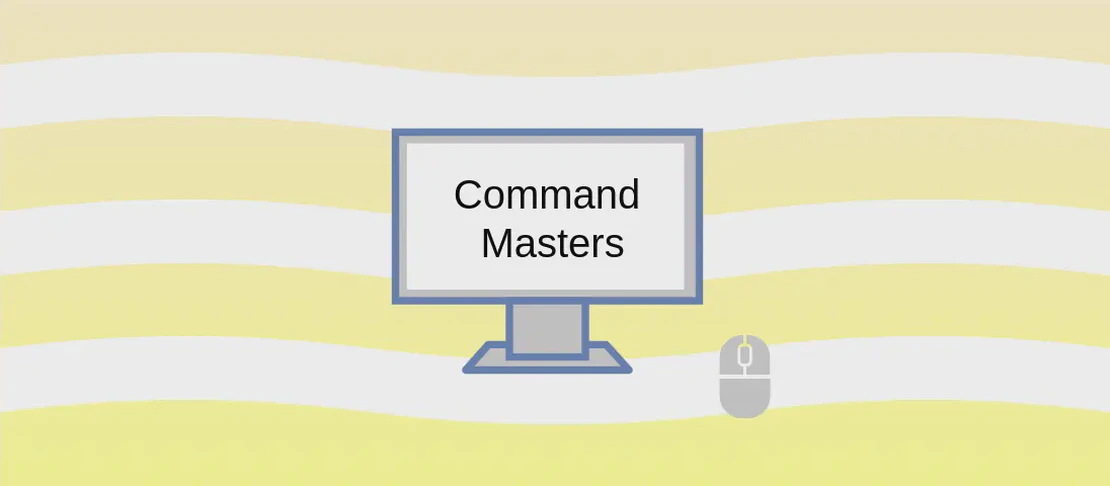
How to Use the Command 'bfs' (with Examples)
‘bfs’ is a versatile command-line tool designed to efficiently perform a breadth-first search to find files and directories based on specific criteria. It’s particularly useful for directory traversal and file management tasks, allowing users to search by file extension, name patterns, size, modification date, and more. Notably, ‘bfs’ can execute commands on the files it locates, making it a powerful utility for system administrators and developers who need to organize or process large numbers of files.
Use case 1: Find Files by Extension
Code:
bfs root_path -name '*.ext'
Motivation:
Finding files by their extension is a common task for anyone managing a large database of documents, images, or code files. Whether you’re seeking all JPEGs in your photo archive or every Python script in a project directory, this use case simplifies locating files quickly based on their file type.
Explanation:
bfs root_path
: Initiates a breadth-first search starting at the ‘root_path’ directory.-name '*.ext'
: Searches for files with an extension ‘.ext’, where ‘*’ matches any sequence of characters. This utilities searches for files ending in the specified extension.
Example Output:
root_path/subdirectory/file1.ext
root_path/another_dir/file2.ext
root_path/yet_another_directory/file3.ext
Use case 2: Find Files Matching Multiple Path/Name Patterns
Code:
bfs root_path -path '**/path/**/*.ext' -or -name '*pattern*'
Motivation:
Sometimes, you might need to identify files located in specific folder paths or those with certain naming patterns. This can be essential for organizing files or preparing for batch processing when dealing with complex directory structures.
Explanation:
bfs root_path
: Starts the search from ‘root_path’.-path '**/path/**/*.ext'
: Uses double asterisks ‘**’ to match zero or more directories within ‘path’ and finds files with the ‘.ext’ extension.-or
: Logical OR operation allowing the search to match any of the specified patterns.-name '*pattern*'
: Matches files containing ‘pattern’ in their name.
Example Output:
root_path/path/to/files/document.ext
root_path/another_directory/useful_pattern_file.txt
Use case 3: Find Directories Matching a Given Name, Case-Insensitive
Code:
bfs root_path -type d -iname '*lib*'
Motivation:
This use case helps find directories when the exact capitalization in names is unknown or varies. This can be particularly helpful in environments where naming conventions aren’t strictly followed or when migrating between different filesystems with case sensitivities.
Explanation:
bfs root_path
: Initiates the search under ‘root_path’.-type d
: Filters search results to include only directories.-iname '*lib*'
: Searches for directory names containing ’lib’, ignoring case differences.
Example Output:
root_path/Library
root_path/project_dir/libFiles
Use case 4: Find Files Matching a Given Pattern, Excluding Specific Paths
Code:
bfs root_path -name '*.py' -not -path '*/site-packages/*'
Motivation:
For Python developers or professionals working with similar frameworks, it might be necessary to identify Python files while excluding third-party libraries or dependencies located in directories such as ‘site-packages’. This use case is crucial for differentiating between local source code and external libraries.
Explanation:
bfs root_path
: Begins the search within ‘root_path’.-name '*.py'
: Looks for files ending with ‘.py’, indicating Python files.-not
: Negates the condition that follows.-path '*/site-packages/*'
: Excludes files within paths containing ‘site-packages’.
Example Output:
root_path/src/file_utilities.py
root_path/tests/test_script.py
Use case 5: Find Files Matching a Given Size Range, Limiting the Recursive Depth to “1”
Code:
bfs root_path -maxdepth 1 -size +500k -size -10M
Motivation:
Identifying files by size is useful for optimizing storage or investigating large files that may unnecessarily consume disk space. By restricting search depth, it’s possible to focus on files directly within the specified directory without traversing into subdirectories.
Explanation:
bfs root_path
: Starts the search from ‘root_path’.-maxdepth 1
: Limits the search to the immediate contents of ‘root_path’, not exploring further down the directory hierarchy.-size +500k
: Searches for files larger than 500 kilobytes.-size -10M
: Limits the search to files smaller than 10 megabytes.
Example Output:
root_path/large_document.txt
root_path/project_archive.zip
Use case 6: Run a Command for Each File
Code:
bfs root_path -name '*.ext' -exec wc -l {} \;
Motivation:
Executing a command on each file is a powerful feature that can automate tasks, such as counting lines in source code files or processing a batch of documents with a script. This use case exemplifies automating repetitive tasks to save time and improve efficiency.
Explanation:
bfs root_path
: Starts searching under ‘root_path’.-name '*.ext'
: Targets files with the specified ‘.ext’ extension.-exec wc -l {} \;
: Executes the ‘wc -l’ command (which counts lines) on each file found. The curly braces ‘{}’ serve as placeholders for each located file, and the semicolon ‘;’ marks the end of the command.
Example Output:
50 root_path/document1.ext
72 root_path/document2.ext
Use case 7: Find All Files Modified Today and Pass the Results to a Single Command as Arguments
Code:
bfs root_path -daystart -mtime -1 -exec tar -cvf archive.tar {} \+
Motivation:
Archiving or backing up files modified within a certain timeframe, such as today, is critical for data management tasks. This use case effectively automates consolidation of daily work into a structured archive.
Explanation:
bfs root_path
: Initiates a search from ‘root_path’.-daystart
: Resets the reference time for relative calculations to the beginning of the current day.-mtime -1
: Searches for files modified in the last day.-exec tar -cvf archive.tar {} \+
: Collects files found into a single ’tar’ archive named ‘archive.tar’. The plus sign ‘+’ concatenates the results into a single command execution.
Example Output:
added file 'root_path/project/note.txt'
added file 'root_path/project/update.log'
Use case 8: Find Empty Files (0 Byte) or Directories and Delete Them Verbosely
Code:
bfs root_path -type f|d -empty -delete -print
Motivation:
Over time, file systems can accumulate empty files or unused directories, leading to clutter and potential confusion. By identifying and removing these empty entities, disk space is optimized, and directory structures are kept tidy and manageable.
Explanation:
bfs root_path
: Begins searching from ‘root_path’.-type f|d
: Targets files (‘f’) or directories (’d’).-empty
: Searches for empty files or directories.-delete
: Removes entities found.-print
: Outputs the names of deleted files or directories for confirmation.
Example Output:
removed 'root_path/empty_document.txt'
removed 'root_path/empty_directory'
Conclusion:
The ‘bfs’ command is a highly efficient tool for file and directory management, allowing users to define detailed search criteria and automate repetitive tasks. Whether you need to organize files, execute operations on multiple documents, or clean up your file system, ‘bfs’ provides accessible and powerful solutions to a variety of common file handling challenges.