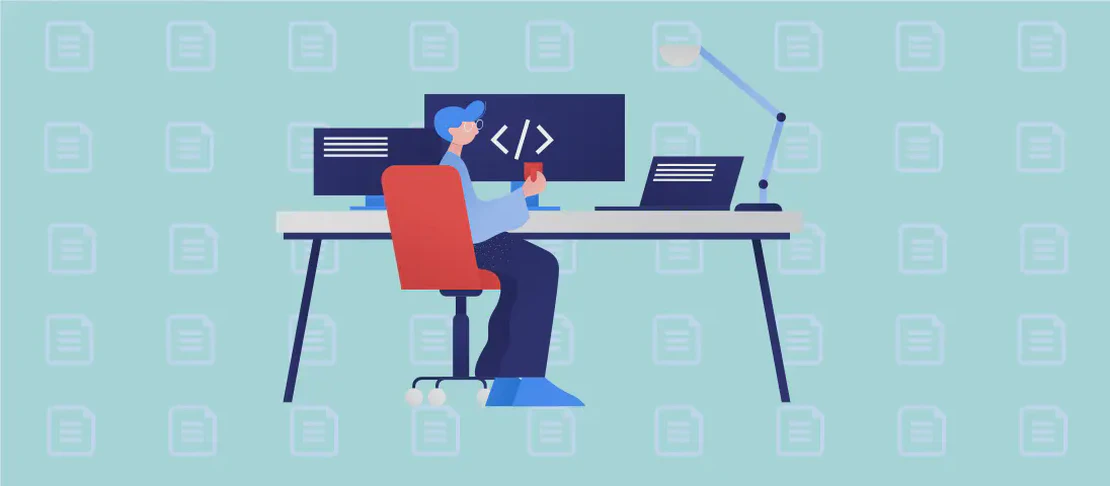
How to use the command 'bitcoin-cli' (with examples)
The bitcoin-cli
is a command-line interface for interacting with the Bitcoin Core daemon, allowing users to perform a variety of tasks ranging from transaction execution to obtaining blockchain and network information. This tool operates through RPC (Remote Procedure Call) commands with configurations set in a file named bitcoin.conf
. It serves as a powerful interface for developers and power users to engage with the Bitcoin network programmatically, enhancing both access and efficiency.
Send a transaction to a given address (with examples)
Code:
bitcoin-cli sendtoaddress "address" amount
Motivation for using the example:
Sending transactions is a fundamental aspect of engaging with the Bitcoin network. Whether purchasing goods, transferring funds to another user, or migrating assets between wallets, executing a transaction command with bitcoin-cli
ensures fast and accurate processing directly from the command line without needing a graphical interface.
Explanation for every argument:
"address"
: This specifies the destination Bitcoin address to which you intend to send funds. It must be a valid Bitcoin address.amount
: Here, you assign the amount of Bitcoin you want to send. It should be expressed in BTC and must be a non-negative number.
Example output:
TRANSACTION_ID
The output will return a transaction ID string, which is a unique identifier for the transaction, confirming that the transaction has been successfully broadcast to the network.
Generate one or more blocks (with examples)
Code:
bitcoin-cli generate num_blocks
Motivation for using the example:
Generating blocks is especially useful in a test network environment where new blocks can be created by users to verify transactions remove stale blocks, or to observe behavior at different block heights. This command is key in isolating and testing various features or protocols before they go live on the main Bitcoin network.
Explanation for every argument:
num_blocks
: This stands for the number of blocks you wish to generate or mine. Typically used in a test network scenario where new blocks are generated at will.
Example output:
[
"blockhash1",
"blockhash2",
...
]
This output lists the unique block hashes of the blocks that were successfully generated and added to the blockchain.
Print high-level information about the wallet (with examples)
Code:
bitcoin-cli getwalletinfo
Motivation for using the example:
Accessing wallet information is crucial for users to keep track of their Bitcoin balance, understand transaction counts, and inspect wallet states. It helps in monitoring security and planning financial strategies accordingly.
Explanation for every argument:
- This command doesn’t take any arguments. It simply fetches and provides an overview of the current state and balance of your wallet.
Example output:
{
"walletname": "Wallet",
"walletversion": 169900,
"balance": 0.12345678,
"unconfirmed_balance": 0.00000000,
"immature_balance": 0.00000000,
"txcount": 14,
"keypoololdest": 1504650493,
"keypoolsize": 1000,
...
}
The output provides a dictionary with comprehensive details about the wallet, including balance, unconfirmed balance, transaction count, and the age and size of the key pool.
List all outputs from previous transactions available to fund outgoing transactions (with examples)
Code:
bitcoin-cli listunspent
Motivation for using the example:
Listing unspent transaction outputs, or UTXOs, aids in identifying funds accessible for future transactions. This function is pertinent to transactions requiring specific coin inputs, such as those needing particular denominations or manual input selection for privacy purposes.
Explanation for every argument:
- This command is used without any required arguments. It pulls a list of all unspent transactions available for spending with the current wallet.
Example output:
[
{
"txid": "transaction_id1",
"vout": 0,
"address": "bitcoin_address",
"account": "",
"scriptPubKey": "script",
"amount": 0.12345678,
"confirmations": 10,
"spendable": true
},
...
]
This output is an array of unspent transactions, detailing identifiers, amounts, addresses, and additional transaction-specific data.
Export the wallet information to a text file (with examples)
Code:
bitcoin-cli dumpwallet "path/to/file"
Motivation for using the example:
Creating backups of your wallet is critical for security and disaster recovery purposes. This command allows users to secure their wallet information in a text file, ensuring re-deployability across platforms and safeguarding against data loss.
Explanation for every argument:
"path/to/file"
: This is the destination path where the wallet information file will be stored. It should include the filename and desired directory location.
Example output:
"filename"
The file will include all the private keys for addresses and corresponding metadata such as labels. The output will confirm successful export by showing the filename.
Get blockchain information (with examples)
Code:
bitcoin-cli getblockchaininfo
Motivation for using the example:
Retrieving blockchain information helps users understand the current status and statistical data about the blockchain. This is useful for developers, miners, or anyone needing in-depth blockchain data for analytics or decision-making.
Explanation for every argument:
- This command takes no arguments. It provides high-level information about the current state of the blockchain.
Example output:
{
"chain": "main",
"blocks": 650000,
"headers": 650000,
"bestblockhash": "hash_value",
"difficulty": 1484528458337.449,
"mediantime": 1613152207,
"verificationprogress": 0.99999925,
...
}
The output delivers an array of data points representing the current blockchain status, including the number of blocks, verification progress, and more.
Get network information (with examples)
Code:
bitcoin-cli getnetworkinfo
Motivation for using the example:
Gathering network statistics offers insight into the P2P (peer-to-peer) connections within the Bitcoin network. It’s especially significant for developers and node operators interested in connectivity, protocol analysis, and network health diagnostics.
Explanation for every argument:
- This command doesn’t require any arguments. It serves to offer comprehensive insights regarding the current network statuses.
Example output:
{
"version": 210000,
"subversion": "/Satoshi:0.21.0/",
"protocolversion": 70015,
"localservices": "0000000000000009",
"localrelay": true,
"timeoffset": 0,
"connections": 8,
...
}
The output is a detailed JSON that includes the protocol version, connection count, local relay, and more crucial network-related data.
Stop the Bitcoin Core daemon (with examples)
Code:
bitcoin-cli stop
Motivation for using the example:
Stopping the Bitcoin Core daemon is essential for maintenance, configuration updates, or general shut down procedures. Gracefully terminating the daemon ensures data integrity and system stability upon re-launch.
Explanation for every argument:
- This command does not require additional arguments; it simply sends a command to stop the currently running Bitcoin Core daemon.
Example output:
"Bitcoin server stopping"
The output confirms that a shutdown command was successfully sent and that the server will cease operations shortly.
Conclusion:
The bitcoin-cli
command-line tool is a powerful utility for administering, managing, and interacting with the Bitcoin network. From sending transactions and generating blocks to gathering comprehensive wallet and blockchain data, bitcoin-cli
encapsulates virtually all operations needed by developers, miners, and Bitcoin enthusiasts to proficiently engage with Bitcoin in a more controlled environment through the command line.