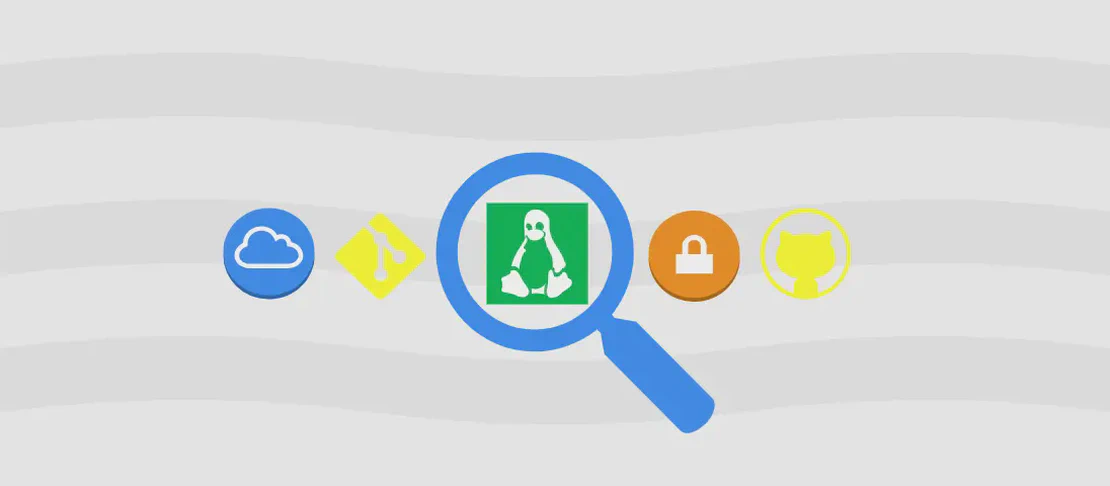
How to use the command 'black' (with examples)
Black is a powerful Python code formatter that enforces consistent coding styles by automatically reformulating code to adhere to PEP 8 standards. Its primary function is to simplify the formatting process and reduce the neeed for code style discussions in code reviews. With just a few commands, it can manage individual files, entire projects, or inline Python code, all while providing insights into what changes it makes.
Use case 1: Auto-format a file or entire directory
Code:
black path/to/file_or_directory
Motivation:
When working on a large Python project, keeping code consistently formatted is crucial for readability and maintenance. Automatic formatting with Black saves time and effort that would otherwise be spent manually adjusting code style.
Explanation:
black
: The command initiates the Black formatter.path/to/file_or_directory
: This specifies the target file or directory that you want to be automatically formatted. By specifying a directory, Black will recursively format all Python files contained within.
Example output:
After running the command, Black processes the files and returns a summary like:
reformatted path/to/file/foo.py
reformatted path/to/file/bar.py
All done! ✨ 🍰 ✨
2 files reformatted.
This output tells you which files were reformatted and confirms completion.
Use case 2: Format the [c]ode passed in as a string
Code:
black -c "code"
Motivation:
When quickly testing snippets of code or when the Python code is embedded within another application, formatting inline strings of code becomes necessary. This can ensure that even temporary or embedded code remains clean and readable.
Explanation:
-c
: This argument signifies that the following input is a string of code rather than a file."code"
: A string containing the Python code that you wish to format.
Example output:
An example output might look like this:
- "a=1\nb=2\nprint(a+b)"
+ "a = 1\nb = 2\nprint(a + b)"
This indicates the changes Black would apply to the string, aligning it with standard Python formatting.
Use case 3: Show whether a file or a directory would have changes made to them if they were to be formatted
Code:
black --check path/to/file_or_directory
Motivation:
Before committing changes or integrating a large project, you often want to assess which files need reformatting without immediately making changes. The --check
option is also useful when setting up Continuous Integration checks.
Explanation:
--check
: A flag that informs you if the files are not formatted according to Black’s standards, without making any changes.path/to/file_or_directory
: Directs Black to check the formatting for the specified file or directory.
Example output:
If there are files that require formatting, you might see:
would reformat path/to/file/foo.py
Oh no! 🎯 💥 🎻
1 file would be reformatted.
This indicates which files are not properly formatted.
Use case 4: Show changes that would be made to a file or a directory without performing them (dry-run)
Code:
black --diff path/to/file_or_directory
Motivation:
When reorganizing existing code, understanding potential changes before they are applied is critical. The --diff
option is essentially a preview mode that many developers find invaluable when incrementally adopting a new formatter.
Explanation:
--diff
: This flag produces a diff output showing the changes that Black would make but does not apply them.path/to/file_or_directory
: Indicates the target file or directory for which you wish to see potential changes.
Example output:
The output will show a diff of what changes would be applied, similar to a Git diff, highlighting modifications, such as:
--- original path/to/file/foo.py
+++ reformatted path/to/file/foo.py
@@ -1,3 +1,3 @@
-def say_hello():
- print("Hello, World!")
+def say_hello():
+ print("Hello, World!")
This showcases proposed formatting changes.
Use case 5: Auto-format a file or directory, emitting exclusively error messages to stderr
Code:
black --quiet path/to/file_or_directory
Motivation:
When you are focusing on error-free integration in larger build systems or scripts, minimizing output to show only critical errors can be beneficial. It keeps logs clean and focuses only on failures.
Explanation:
--quiet
: Suppresses normal output and reports only error messages.path/to/file_or_directory
: Defines the target file or directory for reformatting.
Example output:
If there are no errors, there would be no output. Error messages, if any, will appear in stderr.
Use case 6: Auto-format a file or directory without replacing single quotes with double quotes (adoption helper)
Code:
black --skip-string-normalization path/to/file_or_directory
Motivation:
In legacy codebases or when maintaining third-party code, changing quotation marks might not be desirable. The --skip-string-normalization
feature allows Black to format code while preserving the existing quote style.
Explanation:
--skip-string-normalization
: Directs Black to leave the string quotes unchanged, aiding in incremental adoption of Black.path/to/file_or_directory
: Indicates the file or directory to be formatted with this restriction.
Example output:
Black processes the files and reformats according to all rules except changing quote characters. If no other changes are needed, you might see:
All done! ✨ 🍰 ✨
0 files reformatted, 1 file left unchanged.
This confirms that only essential formatting changes have been applied, without altering quote marks.
Conclusion:
The Black formatter is an invaluable tool for Python developers aiming for consistency and readability in code. By automating code style enforcement, it reduces errors and the overhead of manual formatting, freeing developers to focus on problem-solving and feature development. Black provides flexible options to tailor its behavior to fit projects of varying needs, ensuring both ease of use and comprehensive coverage.