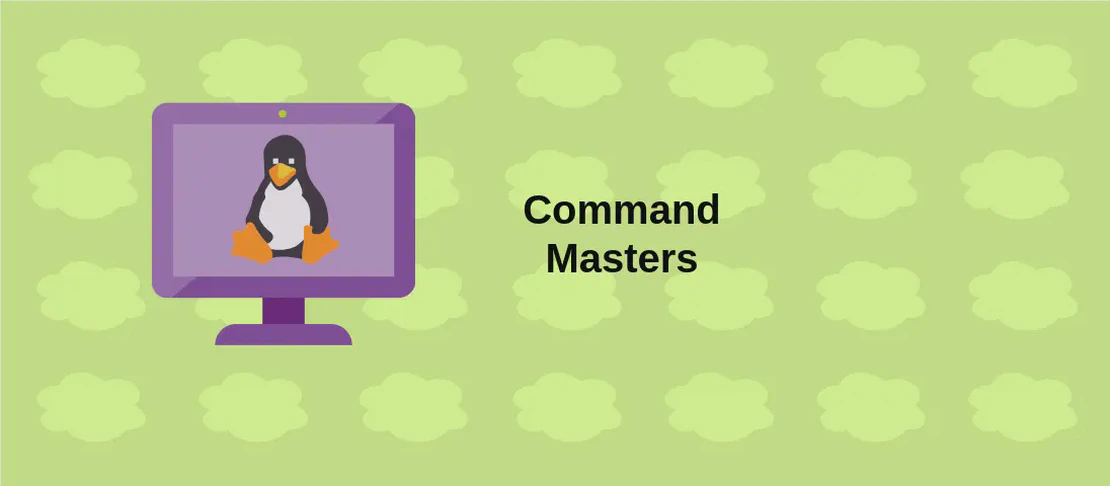
Mastering the Boot Command for Clojure Development (with examples)
Boot is a sophisticated and versatile build tooling system for the Clojure programming language. It provides a dynamic environment for developers to manage dependencies, automate tasks, and streamline the development process. With Boot, you get the ability to work interactively and incrementally, making Clojure development a more efficient and enjoyable experience. Below, we explore several practical use cases of the Boot command to demonstrate its capabilities.
Use case 1: Starting a REPL Session
Code:
boot repl
Motivation:
Running a Read-Eval-Print Loop (REPL) session is a fundamental part of Clojure development. It serves as an interactive environment where developers can test snippets of code, experiment with language features, and debug applications in real-time. The boot repl
command initiates this session, allowing you to work directly with your project’s code or explore Clojure standalone.
Explanation:
boot
: The command-line tool that invokes Boot’s functionality.repl
: A task in Boot that starts the REPL environment. It reads Clojure expressions, evaluates them, and prints the results.
Example Output:
nREPL server started on port 61234 on host 127.0.0.1 - nrepl://127.0.0.1:61234
REPL-y 0.4.4, nREPL 0.6.0
Clojure 1.10.0
Java HotSpot(TM) 64-Bit Server VM 11.0.5+10-LTS
Type :repl/help at the prompt for online help.
Use case 2: Building a Single uberjar
Code:
boot jar
Motivation:
An uberjar
is a JAR file that contains not only your compiled code but also all of its dependencies. This single file can then be easily distributed and run within any Java environment. Creating an uberjar
is essential for deploying applications, as it encapsulates everything needed to execute the program on different machines without worrying about local library dependencies.
Explanation:
boot
: Initiates the Boot tooling system.jar
: This task compiles the project and packages the compiled classes and resources into a JAR file, specifically anuberjar
.
Example Output:
Writing: target/project-name-standalone.jar
Use case 3: Generating Scaffolding for a New Project
Code:
boot --dependencies boot/new new --template template_name --name project_name
Motivation:
When starting a new project, it’s beneficial to have a basic structure already in place. This includes directories, configuration files, and basic code templates that follow best practices. By using Boot to generate scaffolding, developers can jumpstart their projects, ensuring a consistent and sound foundation from which to build.
Explanation:
boot
: Initiates the Boot environment.--dependencies boot/new
: Specifies that we use theboot/new
dependency, which provides the scaffolding functionality.new
: Indicates that we are utilizing the new project task.--template template_name
: The template to be used for the new project’s structure. It can be customized or specified according to user needs.--name project_name
: Sets the name of the new project.
Example Output:
Creating project directory: project_name
Configuring project: Using template template_name
Use case 4: Building for Development
Code:
boot dev
Motivation:
During the development phase, it’s important to have configurations that optimize for speed and flexibility. This typically includes features like hot-reloading code changes, displaying verbose logging, and using mock services. The boot dev
command facilitates creating a development environment tailored to making the coding process as seamless and efficient as possible.
Explanation:
boot
: Launches Boot’s command environment.dev
: Refers to a custom task that developers generally define when using theboot/new
template. It configures the project for development settings.
Example Output:
Starting development server on http://localhost:3000
Use case 5: Building for Production
Code:
boot prod
Motivation:
Before releasing an application to users or deploying to a production environment, it needs to be optimized for stability and performance. The boot prod
command typically uses configuration settings that minify code, optimize assets, and enable only the necessary services. This command ensures that the build is robust and production-ready.
Explanation:
boot
: The command-line tool for the Boot system.prod
: A task that boot/new developers commonly define to compile the application with production-ready settings.
Example Output:
Building project-name with production settings
Use case 6: Displaying Help for a Specific Task
Code:
boot task --help
Motivation:
Boot can handle a myriad of tasks, which can often be specifically tailored or might include non-standard options. The boot task --help
command provides detailed information about any given task, helping developers utilize Boot’s capabilities fully and understand the options and configurations that affect their projects.
Explanation:
boot
: Invokes the Boot system’s tool.task
: The specific task for which you need help information.--help
: A flag that requests the documentation or help file for the specified task.
Example Output:
Task: my-task
Usage: boot my-task options...
Description: This task does something very useful.
Options:
--option-a Description for option a
--option-b Description for option b
Conclusion:
Boot is a powerful tool for Clojure developers, providing robust support for a wide range of building and development tasks. Whether you’re starting a new project, developing, testing, or deploying your application, Boot helps streamline these processes, allowing you to focus on writing effective and efficient Clojure code. By understanding and applying the use cases above, developers can make the most of what Boot offers and optimize their Clojure workflows.