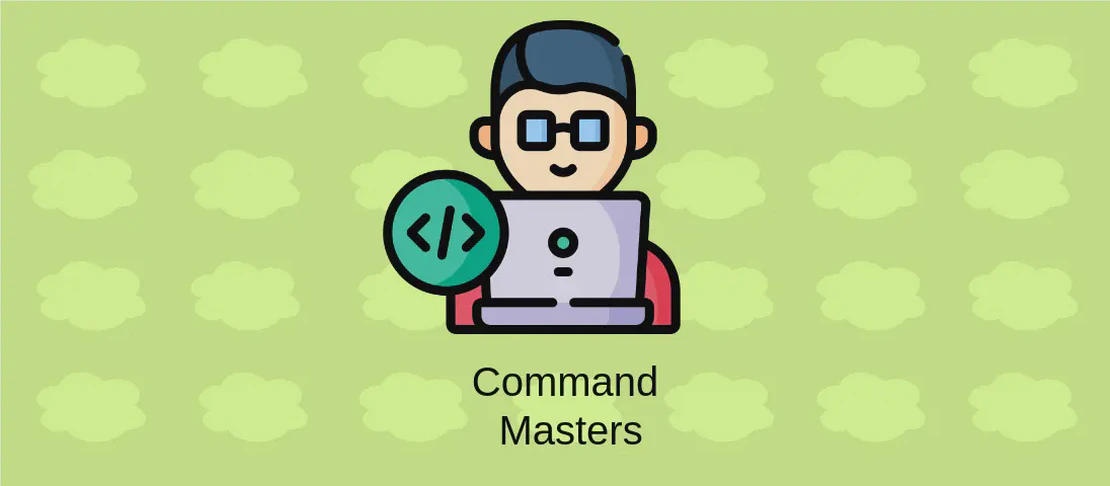
How to Use the Command 'bower' (with examples)
Bower is a package manager specifically designed for front-end web development. It assists developers by simplifying the management of libraries, frameworks, and other packages required for building web applications. Bower allows developers to easily install, update, and manage the dependencies that a project needs to run. It facilitates the retrieval of these dependencies from various sources such as GitHub or a specific URL, making the development process more streamlined and efficient.
Use case 1: Install a project’s dependencies, listed in its bower.json
Code:
bower install
Motivation: When you work on a project that uses Bower, its necessary dependencies are typically listed in a file named bower.json
. Running bower install
simplifies the process of setting up the project environment by automatically downloading and installing all required packages. This is incredibly useful when you clone a new project repository and need to get it up and running quickly.
Explanation:
bower
: This is the command to invoke the Bower package manager.install
: This argument tells Bower to look at thebower.json
file in the current directory, identify the dependencies listed there, and install them into thebower_components
directory.
Example output:
bower cached git://example.com/repo.git#1.0.0
bower validate 1.0.0 against git://example.com/repo.git#*
bower install repo#1.0.0
repo#1.0.0 bower_components/repo
Use case 2: Install one or more packages to the bower_components directory
Code:
bower install package1 package2
Motivation: Sometimes a developer might want to add specific new packages to an existing project. By specifying the package names and using bower install
, you ensure that Bower fetches and installs those packages into the bower_components
directory. This manual selection allows for more control over the project’s dependencies.
Explanation:
bower
: Initiates the Bower command-line tool.install
: This instructs Bower to proceed with the installation.package1 package2
: These are placeholders for the package names you want to install. You can specify one or more package names to download them concurrently.
Example output:
bower not-cached git://github.com/example/package1.git
bower resolve git://github.com/example/package1.git#*
bower checkout package1#master
...
bower install package1#x.y.z
package1#x.y.z bower_components/package1
Use case 3: Uninstall packages locally from the bower_components directory
Code:
bower uninstall package1 package2
Motivation: Over time, some packages may become unnecessary, or a developer might wish to remove specific libraries due to conflicts or redundancy. The bower uninstall
command serves to clean up the bower_components
directory by removing unwanted packages, thus maintaining a more organized project structure.
Explanation:
bower
: This calls upon the Bower utility.uninstall
: Signals Bower to remove specific packages.package1 package2
: These are the names of the packages you intend to uninstall.
Example output:
bower uninstall package1
bower uninstall package2
removed bower_components/package1
removed bower_components/package2
Use case 4: List local packages and possible updates
Code:
bower list
Motivation: Knowing the current state of installed packages is crucial for maintaining software projects. With the bower list
command, developers can view all locally installed packages and identify any available updates. This serves to ensure that all dependencies are aligned and up-to-date, potentially reducing bugs and performance issues.
Explanation:
bower
: Activates the Bower package manager.list
: Directs Bower to display a list of all currently installed packages and their statuses, including any that might need updates.
Example output:
bower check-new Checking for updates of all packages...
bower outdated package1#1.0.0 -> x.y.z
Installed packages:
package1#1.0.0 (latest is x.y.z)
package2#0.9.0
Use case 5: Create a bower.json
file for your package
Code:
bower init
Motivation: A bower.json
file is essential for any project using Bower as it explicitly outlines all required dependencies. Using the bower init
command, developers can quickly generate this file by providing the necessary information interactively. This facilitates easy sharing and setup of the project’s environment by other developers.
Explanation:
bower
: Utilizes the Bower tool.init
: Commands Bower to initiate an interactive process to gather information and generate abower.json
file.
Example output:
? name
? description
? main file
? what types of modules does this package expose
? keywords
? authors
? license
...
Use case 6: Install a specific dependency version, and add it to bower.json
Code:
bower install local_name=package#version --save
Motivation: In some cases, a specific version of a package is required to maintain compatibility or to benefit from new features and improvements. By specifying the version, bower install
ensures that the correct package version is integrated into the project. The --save
flag automatically updates bower.json
so that all contributors remain aligned on dependencies.
Explanation:
bower
: Accesses the Bower command interface.install
: Initiates the installation procedure.local_name=package#version
: Installs the specified version of the package under an optionally local alias.--save
: Updates thebower.json
file to reflect this dependency, ensuring it will be recognized in subsequent installations.
Example output:
bower not-cached git://github.com/example/package.git#v2.0.0
bower resolve git://github.com/example/package.git#v2.0.0
...
bower install package#v2.0.0
package#v2.0.0 bower_components/package
Use case 7: Display help for a specific command
Code:
bower help command
Motivation: Understanding how to use Bower commands effectively is vital, particularly for new developers. The bower help
command provides detailed explanations and uses for specific commands, making it an indispensable resource for clarifying doubts and expanding knowledge on Bower functionality.
Explanation:
bower
: Calls upon the Bower tool.help
: Triggers Bower’s help documentation.command
: Represents the specific Bower command you need help with, offering insights into its options and uses.
Example output:
Usage:
bower install <packages> <options>
Options:
-f, --force-latest Force latest version on conflict
...
Conclusion:
Bower provides a set of powerful commands designed to simplify the management of front-end web development dependencies. From setting up initial project environments to managing existing installations and seeking help, Bower streamlines many aspects of dependency management, making it a beneficial tool for web developers who need an efficient means to handle multiple packages.