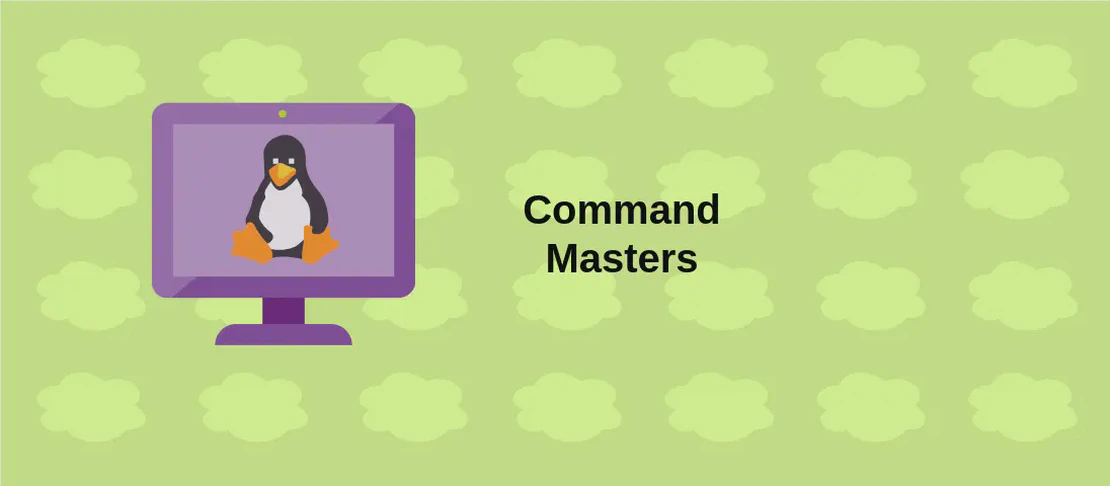
How to use the command 'bun' (with examples)
Bun is a modern JavaScript runtime and toolkit that stands out due to its powerful built-in features, including a JavaScript runtime, a bundler, a test runner, and a package manager. It strives to be a comprehensive solution for JavaScript developers by simplifying tasks commonly handled by Node.js, Webpack, Jest, and npm. The command provides a range of functionalities, from running scripts to managing dependencies, making it particularly versatile and valuable in the development process.
Use case 1: Run a JavaScript file or a package.json
script
Code:
bun run path/to/file|script_name
Motivation:
Running JavaScript files or scripts defined in package.json
is a fundamental part of any development workflow. Whether you’re executing a script to build, start a server, or a custom task, the bun run
command streamlines the process by offering a quick and efficient way to get your code up and running.
Explanation:
bun run
: This invocation of Bun is used to execute JavaScript files or scripts defined in thepackage.json
.path/to/file|script_name
: Specifying either a file path or the name of a script frompackage.json
. If it’s a file, Bun will execute it; if it’s a script, Bun will locate and run it.
Example output:
Suppose you run a script named “start” in package.json
:
$ bun run start
Server is running at http://localhost:3000
Use case 2: Run unit tests
Code:
bun test
Motivation:
Automated testing is critical for maintaining code quality and ensuring new features or bug fixes do not break existing functionality. The bun test
command integrates testing within your workflow, making it easier to run and manage tests.
Explanation:
bun test
: This command identifies and runs all the test files in your directory that match common test file conventions (e.g., files ending in.test.js
or placed in atests
folder).
Example output:
$ bun test
PASS ./sum.test.js
PASS ./product.test.js
Test Suites: 2 passed, 2 total
Tests: 5 passed, 5 total
Snapshots: 0 total
Use case 3: Download and install all the packages listed as dependencies in package.json
Code:
bun install
Motivation:
Managing project dependencies is crucial for any software project. The bun install
command simplifies this process by automating the download and setup of all necessary packages, ensuring that your development environment matches the project’s requirements.
Explanation:
bun install
: Directs Bun to read thepackage.json
file and install all listed dependencies, including devDependencies.
Example output:
$ bun install
Downloading dependencies...
Finished installing 120 packages
Use case 4: Add a dependency to package.json
Code:
bun add module_name
Motivation:
When developing, you’ll often need to add new dependencies to your project. The bun add
command facilitates this by automatically updating your package.json
and installing the package without needing to manually edit your package.json
.
Explanation:
bun add
: Initiates the process of adding a new dependency.module_name
: The name of the package you wish to add. This directs Bun to fetch and include the specified package as a dependency.
Example output:
$ bun add lodash
Adding lodash...
Installed lodash@4.17.21
Use case 5: Remove a dependency from package.json
Code:
bun remove module_name
Motivation:
As projects evolve, certain dependencies may become redundant or be replaced. The bun remove
command offers a streamlined way to cleanse your project of unused or obsolete packages by updating the package.json and removing the dependency files.
Explanation:
bun remove
: Commands Bun to initiate the removal of a dependency.module_name
: Specifies which dependency to eliminate from your project.
Example output:
$ bun remove lodash
Removing lodash...
Removed lodash@4.17.21
Use case 6: Create a new Bun project in the current directory
Code:
bun init
Motivation:
Starting a new project requires setting up basic project structure and configurations. The bun init
command simplifies the initial setup process by automatically generating a package.json
file and other essential files, providing a convenient starting point for your project.
Explanation:
bun init
: Executes Bun’s project initialization process, crafting a newpackage.json
alongside other configuration essentials, tailored to kickstart new projects.
Example output:
$ bun init
Created package.json
Initialized empty Git repository
Use case 7: Start a REPL (interactive shell)
Code:
bun repl
Motivation:
Having an interactive shell allows for immediate experimentation and debugging of JavaScript code. With the bun repl
command, you can quickly test out JavaScript code snippets without needing to create or run a separate file.
Explanation:
bun repl
: Opens an interactive session where you can type and execute JavaScript expressions live.
Example output:
$ bun repl
Bun REPL:
> console.log('Hello, Bun!')
Hello, Bun!
Use case 8: Upgrade Bun to the latest version
Code:
bun upgrade
Motivation:
Staying up-to-date with the latest features and security patches is critical for any software tool. The bun upgrade
command ensures you always have the latest version of Bun, allowing you to benefit from ongoing performance improvements and new capabilities.
Explanation:
bun upgrade
: Instructs the Bun package manager to check for and install the latest available version.
Example output:
$ bun upgrade
Checking for updates...
Upgraded Bun to version 0.3.0
Conclusion:
Bun represents a robust solution for modern JavaScript development, packing various essential tools into a single JavaScript runtime. Whether you are managing dependencies, running tests, or initializing new projects, Bun’s simple yet powerful commands allow developers to cleanly handle a wide range of tasks with ease. By mastering these use cases, you can streamline your development workflow, making Bun a handy tool in any JavaScript developer’s toolkit.