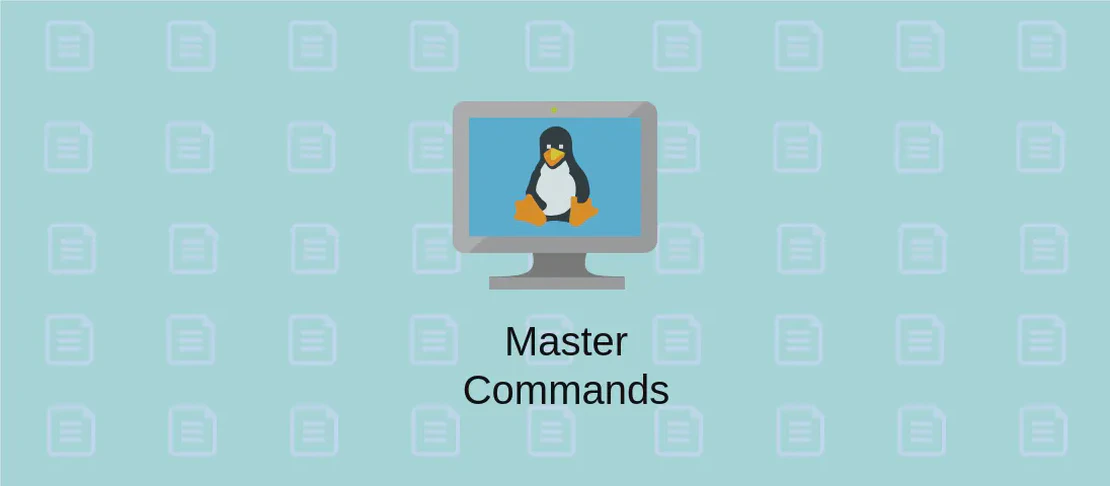
Utilizing the Command 'bundle' (with examples)
Bundler is an essential utility in the Ruby ecosystem, simplifying the management of an application’s dependencies. It ensures that the gems (libraries) required for your application are installed in the versions specified so that your code runs consistently across different environments. By utilizing a Gemfile
, Bundler helps keep all required gems organized and up to date, preventing potential compatibility issues and streamlining development.
Use case 1: Install all gems defined in the Gemfile
Code:
bundle install
Motivation:
When you embark on working with a Ruby application, one of the first steps is ensuring all necessary gems are available in your environment. Running bundle install
ensures that every dependency specified in your Gemfile
is fetched and installed onto your system. This command is particularly crucial when setting up a fresh copy of a project as it guarantees that your environment mirrors that of the original development setup.
Explanation:
bundle install
: This command reads the dependencies listed in yourGemfile
and installs them, thereby creating a consistent state for your application’s development environment. It also locks the exact versions of the gems in theGemfile.lock
to prevent any future conflicts.
Example output:
Fetching gem metadata from https://rubygems.org/...
Resolving dependencies...
Using rake 12.3.1
Using bundler 2.2.0
Using rails 5.2.3
...
Bundle complete! 18 Gemfile dependencies, 84 gems now installed.
Use `bundle info [gemname]` to see where a bundled gem is installed.
Use case 2: Execute a command in the context of the current bundle
Code:
bundle exec command arguments
Motivation:
Frequently, you need to run scripts or commands that require specific gems your application depends on. However, simply executing them might lead to version conflicts or other unexpected issues if the default system gems differ from those specified in your Gemfile
. Therefore, using bundle exec
ensures you are utilizing the gem versions that are defined for your application, promoting reliability and consistency across environments.
Explanation:
bundle exec
: Ensures the command that follows is executed in the context of the gems in your Gemfile. This mitigates issues arising from incompatible or unintended versions of libraries.command arguments
: This is the specific command, possibly with arguments, you want to execute using the environment set by Bundler.
Example output (for an example command bundle exec rails server
):
=> Booting Puma
=> Rails 5.2.3 application starting in development
=> Run `rails server -h` for more startup options
Listening on tcp://localhost:3000
Use case 3: Update all gems by the rules defined in the Gemfile
Code:
bundle update
Motivation:
Applications can quickly fall behind by using older versions of software libraries. Keeping dependencies up to date is paramount for leveraging the latest features, performance improvements, and security patches. bundle update
comes handy in aligning your application with the latest versions of gems allowed by your Gemfile
.
Explanation:
bundle update
: This command updates all the gems to the newest versions as restricted by yourGemfile
. It also regenerates theGemfile.lock
to lock the versions of the now updated gems.
Example output:
Resolving dependencies...
Fetching rake 13.0.6 (was 12.3.1)
Fetching rails 6.0.3 (was 5.2.3)
...
Bundle updated! 18 Gemfile dependencies, 84 gems now installed.
Use case 4: Update one or more specific gem(s) defined in the Gemfile
Code:
bundle update gem_name1 gem_name2
Motivation:
At times, you may need to update specific gems due to newly released features, patches, or for testing purposes, without wanting to disturb the rest of your dependency set. The bundle update
command enables selective updating of gems as per your requirements, thus minimizing the likelihood of introducing unintended side effects to your application.
Explanation:
bundle update
: Specifies that gem(s) need to be updated.gem_name1 gem_name2
: List of specific gem names you want Bundler to update according to the constraints defined in theGemfile
.
Example output:
Resolving dependencies...
Fetching gem_name1 2.1 (was 2.0)
Fetching gem_name2 4.2 (was 4.0)
Using rack 2.2.3
...
Use case 5: Update one or more specific gems(s) defined in the Gemfile
but only to the next patch version
Code:
bundle update --patch gem_name1 gem_name2
Motivation:
Restrictive updates are sometimes necessary to ensure maximum stability while benefiting from minor improvements or bug fixes. Running the bundle update --patch
limits the updates to minor patches, avoiding potentially unstable changes that could break functionalities or required interfaces of your global setup.
Explanation:
bundle update --patch
: Indicates Bundler should limit updates to the next patch release.gem_name1 gem_name2
: Specifies the exact gems for restricted updates, only applying available patch versions for these.
Example output:
Resolving dependencies...
Fetching gem_name1 2.0.1 (was 2.0)
Fetching gem_name2 4.0.1 (was 4.0)
Use case 6: Update all gems within the given group in the Gemfile
Code:
bundle update --group development
Motivation:
Projects often include segmented dependencies for different environments, such as development, testing, or production. To augment development productivity and ensure compatibility with recent tools and practices, using bundle update --group
focuses updates within specific dependency groups without disturbing other gem categories.
Explanation:
bundle update --group
: Limits updating to the specified group of gems.development
: The group parameter that determines which gems from theGemfile
should be updated.
Example output:
Resolving dependencies...
Fetching pry 0.14.1 (was 0.14.0)
Fetching rubocop 1.23 (was 1.22)
...
Updated development group gems successfully.
Use case 7: List installed gems in the Gemfile
with newer versions available
Code:
bundle outdated
Motivation:
Assessing which dependencies are out-of-date allows developers to maintain an optimal versioning of dependencies. Running bundle outdated
equips developers not only with the awareness of available updates but also helps in strategizing version upgrades to align with company policies or project needs, fortifying application stability and security.
Explanation:
bundle outdated
: Scans the installed gems specified in your Gemfile and reports any that have newer versions available.
Example output:
Outdated gems included in the bundle:
* rails (newest 6.0.3, installed 5.2.3)
* rack (newest 2.2.3, installed 2.1.1)
Use case 8: Create a new gem skeleton
Code:
bundle gem gem_name
Motivation:
When starting the development of a new Ruby gem, establishing a coherent structure and following best practice setups is crucial. bundle gem
assists in jumpstarting this task by automatically generating a scaffold with directories and files adhering to conventions. Such preparation ensures uniformity across multiple projects, promoting maintainable and easily navigable codebases.
Explanation:
bundle gem
: Initiates the process of creating a gem scaffold.gem_name
: Designates the desired name for the new gem, which then generates corresponding directory and file names.
Example output:
Creating gem 'gem_name'...
create gem_name/Gemfile
create gem_name/lib/gem_name.rb
create gem_name/lib/gem_name/version.rb
create gem_name/Rakefile
create gem_name/gem_name.gemspec
create gem_name/README.md
...
Gem 'gem_name' has been created!
Conclusion:
Bundler simplifies Ruby project management, ensuring consistent environments, managing dependencies, and accelerating development with structured tools and methodologies. From executing commands with the right dependencies to maintaining bleeding-edge or stable setups, these bundled utilities deliver reliable and scalable options for any Ruby developer exploring Gemfile potentials.