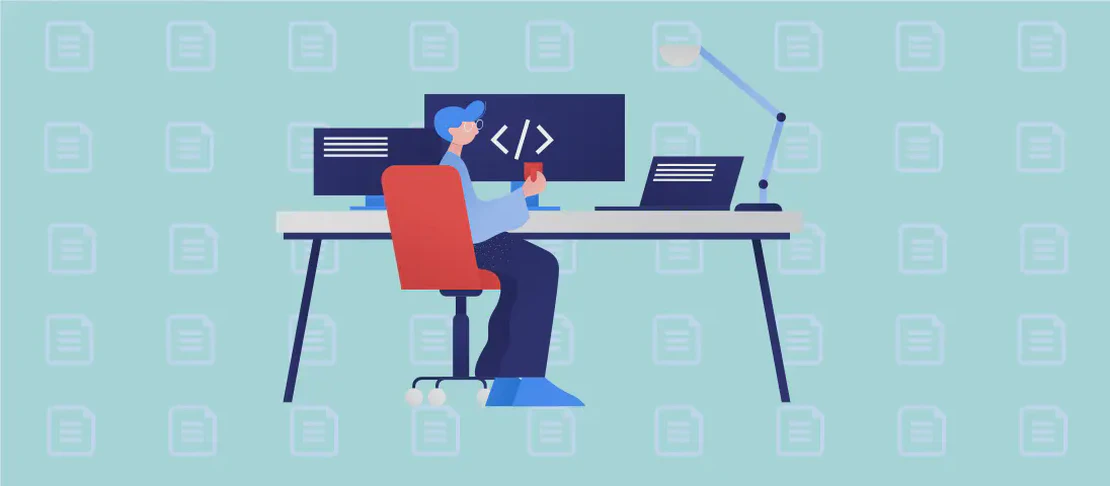
How to use the command 'bundletool' (with examples)
Bundletool is an essential command-line tool designed by Google to work with Android Application Bundles (AAB). An Android App Bundle is a new distribution format that Google Play uses to deliver optimized APKs for each device configuration, which means smaller app downloads and updates for end-users. Bundletool lets developers generate APK sets from an AAB, validate these bundles, and install them on devices, among other functions. This article explores various use cases of the bundletool
command, offering insightful examples and explanations for each scenario.
Use case 1: Display help for a subcommand
Code:
bundletool help subcommand
Motivation:
Developers often require detailed instructions on how to efficiently use a specific command or subcommand. The help
feature in bundletool
provides essential guidance on the usage and options available for a specific subcommand, making it invaluable for both learning and troubleshooting purposes.
Explanation:
help
: This argument instructsbundletool
to display help documentation.subcommand
: This placeholder represents any subcommand (likebuild-apks
,install-apks
, etc.) for which help is needed.
Example output:
Usage: bundletool build-apks [OPTIONS]
Options:
--bundle <BUNDLE> Path to the App Bundle.
--output <OUTPUT> Path to the output APK Set.
–-ks <KEYSTORE> Path to the keystore.
...
Use case 2: Generate APKs from an application bundle (prompts for keystore password)
Code:
bundletool build-apks --bundle path/to/bundle.aab --ks path/to/key.keystore --ks-key-alias key_alias --output path/to/file.apks
Motivation:
When distributing an Android application, developers need to convert an AAB into a set of APKs tailored for different device configurations. This conversion process is crucial for app testing and distribution beyond the Play Store, such as for internal testing across various devices.
Explanation:
build-apks
: This subcommand tellsbundletool
to generate APKs from the specified AAB.--bundle path/to/bundle.aab
: Indicates the path to the application bundle file that needs to be converted.--ks path/to/key.keystore
: Specifies the path to the keystore used for signing the APKs.--ks-key-alias key_alias
: The alias of the key within the keystore used for signing APKs.--output path/to/file.apks
: Specifies the path to the output file where the generated APKs will be saved.
Example output:
INFO: Starting APK generation.
Enter keystore password:
INFO: APK Set file generated at 'path/to/file.apks'
Use case 3: Generate APKs from an application bundle giving the keystore password
Code:
bundletool build-apks --bundle path/to/bundle.aab --ks path/to/key.keystore --ks-key-alias key_alias –ks-pass pass:the_password --output path/to/file.apks
Motivation:
Automating the APK generation process, especially in continuous integration/continuous deployment (CI/CD) environments, can be highly beneficial. This command shows how to supply the keystore password directly within the command, thus allowing script-based executions without manual password entry.
Explanation:
build-apks
: Initiates APK generation from the specified AAB.--bundle path/to/bundle.aab
: Provides the path to the Android application bundle.--ks path/to/key.keystore
: Indicates the path to the signing keystore.--ks-key-alias key_alias
: The alias of the keystore key for signing.–ks-pass pass:the_password
: Supplies the password for the keystore directly.--output path/to/file.apks
: Specifies where the resulting APKs should be saved.
Example output:
INFO: Starting APK generation.
INFO: APK Set file generated at 'path/to/file.apks'
Use case 4: Generate APKs including only one single APK for universal usage
Code:
bundletool build-apks --bundle path/to/bundle.aab --mode universal --ks path/to/key.keystore --ks-key-alias key_alias --output path/to/file.apks
Motivation:
Sometimes, developers need a universal APK, which is a single APK that contains resources for all device configurations. This is particularly useful for internal usage or alternative distribution channels where size optimization for different devices isn’t necessary.
Explanation:
build-apks
: Instructsbundletool
to generate APK files from an AAB.--bundle path/to/bundle.aab
: Indicates the path to the app bundle.--mode universal
: Tellsbundletool
to generate a universal APK, which contains all configurations.--ks path/to/key.keystore
: Points to the keystore for signing the APK.--ks-key-alias key_alias
: The alias in the keystore used for signing.--output path/to/file.apks
: Path where the output APK will be saved.
Example output:
INFO: Starting APK generation for universal mode.
INFO: Universal APK generated at 'path/to/file.apks'
Use case 5: Install the right combination of APKs to an emulator or device
Code:
bundletool install-apks --apks path/to/file.apks
Motivation:
Testing applications on actual devices or emulators is a vital part of the Android development process. This command helps developers install the right combination of APKs optimized for a particular device directly, saving time and ensuring the application performs as expected on target devices.
Explanation:
install-apks
: A subcommand for installing APKs on a connected device or emulator.--apks path/to/file.apks
: Specifies the path to the APK set file that contains the necessary APKs for installation.
Example output:
INFO: APK Set deployed successfully to device/emulator.
Use case 6: Estimate the download size of an application
Code:
bundletool get-size total --apks path/to/file.apks
Motivation:
Understanding the download size of an application ahead of release can help developers make informed decisions about content optimization and user bandwidth considerations. This is especially important as consumers in different locations may face network constraints that affect their willingness to download large apps.
Explanation:
get-size
: A subcommand used to estimate the download size of the application.total
: Specifies that the command should calculate the total size of the APK.--apks path/to/file.apks
: The path to the APK set file used for size estimation.
Example output:
INFO: Estimated total download size: 15MB
Use case 7: Generate a device specification JSON file for an emulator or device
Code:
bundletool get-device-spec --output path/to/file.json
Motivation:
Generating a device specification JSON file is essential for developers who need a precise understanding of the device’s configuration. This information is crucial to tailor APKs specific to the device’s characteristics, ensuring optimal performance.
Explanation:
get-device-spec
: A subcommand to retrieve the device specification.--output path/to/file.json
: Specifies where to save the generated JSON file containing the device’s specifications.
Example output:
INFO: Device specification file created at 'path/to/file.json'
Use case 8: Verify a bundle and display detailed information about it
Code:
bundletool validate --bundle path/to/bundle.aab
Motivation:
Before distribution, validating an application bundle ensures that it complies with Android standards and is free of errors. This step helps prevent potential issues during the app release process, allowing developers to rectify problems early.
Explanation:
validate
: A subcommand to verify the integrity and correctness of an AAB file.--bundle path/to/bundle.aab
: Provides the path to the specific Application Bundle to be validated.
Example output:
INFO: Bundle 'bundle.aab' is valid.
Conclusion:
Bundletool offers Android developers robust versatility in managing Application Bundles. From generating APK sets and installing them to estimating sizes and validating bundles, this command-line tool facilitates different crucial processes in developing and distributing Android applications efficiently. Understanding and utilizing the use cases described above ensures a more streamlined development workflow and a smoother user experience.