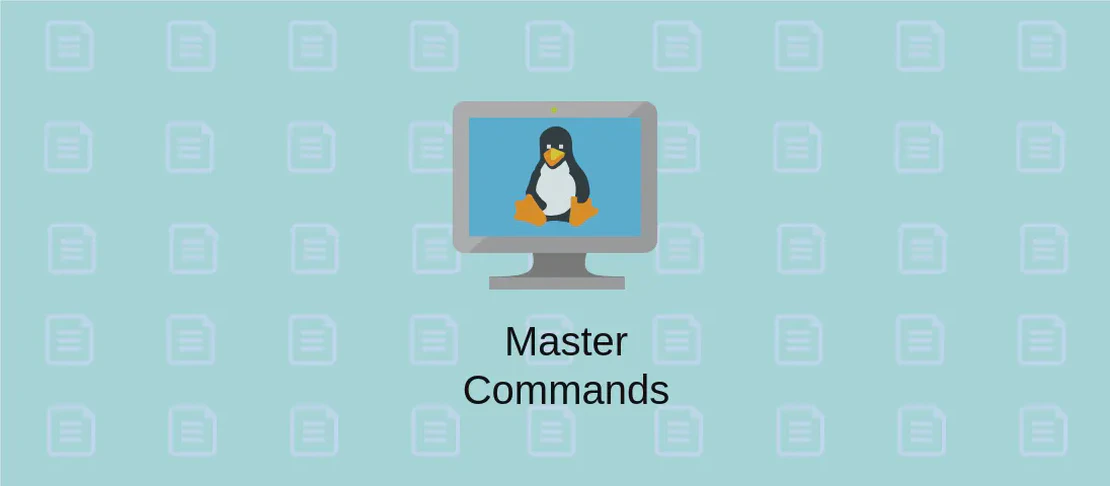
How to Use the Command 'bundletool dump' (with Examples)
- Linux , Macos , Windows , Android , Bundletool
- December 17, 2024
The bundletool dump
command is a versatile tool designed to manipulate Android Application Bundles (AAB files). Android App Bundles are a type of format for publishing and distributing Android applications on the Google Play Store. These bundles help optimize the app delivery process by enabling the generation and usage of smaller, optimized APKs based on the device’s requirements. bundletool
provides insights into the contents and structure of these bundles, allowing developers to understand and modify them more effectively. Below, we explore various use cases of the bundletool dump
command with practical examples and detailed explanations.
Use Case 1: Display the AndroidManifest.xml
of the Base Module
Code:
bundletool dump manifest --bundle path/to/bundle.aab
Motivation:
In the Android app development process, the AndroidManifest.xml
is a vital file that defines essential information about the application, such as permissions, components, and activities. Viewing the manifest of the base module is crucial for debugging and verifying the app’s initial setup.
Explanation:
bundletool
: The command-line tool used to interact with Android App Bundles.dump
: A command to extract specific data from the bundle.manifest
: Specifies that the tool should show theAndroidManifest.xml
.--bundle
: Argument to specify the path to the Android App Bundle (.aab) file we are examining.path/to/bundle.aab
: Placeholder for the actual path to the bundle file.
Example Output:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.app"
android:versionCode="1"
android:versionName="1.0">
...
</manifest>
Use Case 2: Display a Specific Value from the AndroidManifest.xml
Using XPath
Code:
bundletool dump manifest --bundle path/to/bundle.aab --xpath /manifest/@android:versionCode
Motivation:
When maintaining or updating an application, developers often need to check specific attributes within the manifest, such as the app version code, without parsing the entire XML file.
Explanation:
--xpath
: Allows querying specific parts of the manifest using XPath expressions./manifest/@android:versionCode
: XPath query to extract theversionCode
attribute from the manifest node.
Example Output:
1
Use Case 3: Display the AndroidManifest.xml
of a Specific Module
Code:
bundletool dump manifest --bundle path/to/bundle.aab --module name
Motivation:
Complex applications may consist of multiple modules, each with its manifest file. Viewing the manifest of a specific module helps verify modular attributes and dependencies, aiding in efficient debugging or module-specific modifications.
Explanation:
--module
: Specifies which module’s manifest to display.name
: The actual name of the module whose manifest is requested.
Example Output:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.module" ...>
...
</manifest>
Use Case 4: Display All the Resources in the Application Bundle
Code:
bundletool dump resources --bundle path/to/bundle.aab
Motivation:
Understanding the resources packaged within a bundle is essential for developers aiming to optimize resource usage or troubleshoot issues related to resource access.
Explanation:
resources
: Instructs the tool to list resources contained in the bundle.
Example Output:
drawable/icon.png
layout/activity_main.xml
values/strings.xml
...
Use Case 5: Display the Configuration for a Specific Resource
Code:
bundletool dump resources --bundle path/to/bundle.aab --resource type/name
Motivation:
Dealing with resource configurations is crucial in scenarios such as supporting multiple device specifications or localizations. This use case allows developers to inspect how resources are configured.
Explanation:
--resource
: A specific resource type and name which configuration should be queried.type/name
: The complete definition of the resource, likedrawable/icon
.
Example Output:
density: nodpi
locale: en
Use Case 6: Display the Configuration and Values for a Specific Resource Using the ID
Code:
bundletool dump resources --bundle path/to/bundle.aab --resource 0x7f0e013a --values
Motivation:
Resource IDs are unique identifiers used to refer to resources programmatically. By using the resource ID, developers can extract both configuration and actual values, aiding in a more in-depth analysis of resource implementation.
Explanation:
0x7f0e013a
: The hexadecimal identifier of the resource.--values
: Option to also extract associated values along with configurations.
Example Output:
Resource: 0x7f0e013a
Configuration: land-xxhdpi
Value: Hello World!
Use Case 7: Display the Contents of the Bundle Configuration File
Code:
bundletool dump config --bundle path/to/bundle.aab
Motivation:
Examining the bundle configuration is essential for developers working on optimizing app delivery by understanding the structure and features that bundle encapsulates.
Explanation:
config
: Specifies the extraction of the bundle’s configuration aspect.
Example Output:
version: 1.0
compression: true
modules:
- base
- feature1
Conclusion:
Using bundletool dump
, developers can effectively access and inspect various elements of an Android Application Bundle, thereby aiding in debugging, optimizing, and understanding their applications. With the detailed use cases described above, you can tailor the command’s capabilities to suit your specific project needs.