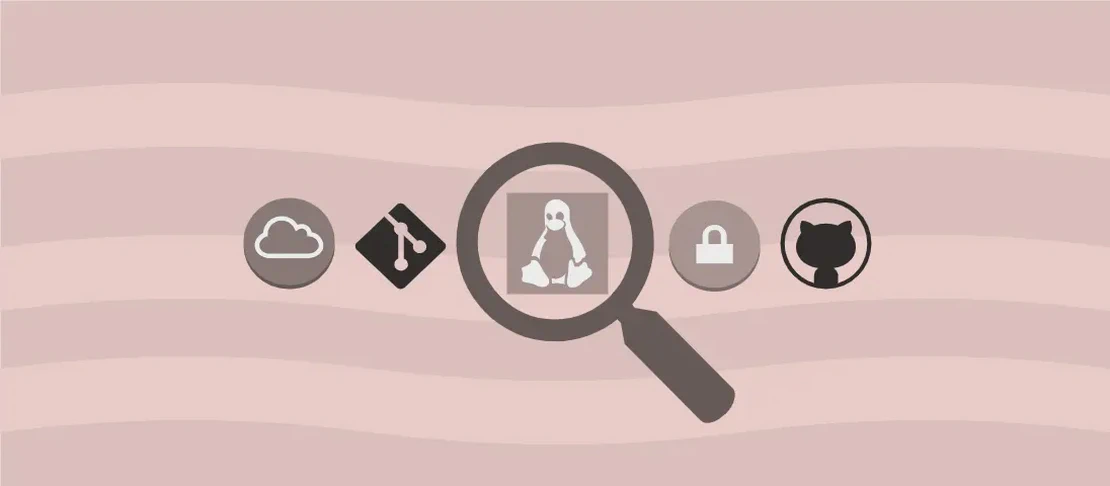
Exploring Caddy Web Server Functions (with examples)
Caddy is an enterprise-ready, open-source web server known for its ability to automatically handle HTTPS, making it a preferred choice among developers who prioritize security and ease of use. Written in the Go programming language, Caddy offers a range of functionalities that simplify web server management and deployment. Whether you’re running a simple file server or a reverse proxy, Caddy ensures smooth operations.
Use case 1: Start Caddy in the foreground
Code:
caddy run
Motivation: Running Caddy in the foreground is particularly useful during the development phase when immediate feedback on server operations is necessary. It allows developers to see real-time outputs and logs directly in the console, facilitating easier debugging and testing of web applications.
Explanation:
caddy
: The command initiates Caddy.run
: This sub-command starts the Caddy server in the foreground, meaning it will continue to run actively in the current terminal session until manually stopped.
Example Output: When executed, the terminal will display the Caddy server logs and real-time operations, including HTTPS setup logs and any requests being processed.
Use case 2: Start Caddy with the specified Caddyfile
Code:
caddy run --config path/to/Caddyfile
Motivation: Using a Caddyfile allows developers to configure Caddy with specific custom settings, such as defining different site addresses, paths to files, or directives for the web server. This is ideal for those needing a tailored web server configuration beyond the default settings.
Explanation:
--config
: This flag specifies the path to the configuration file (Caddyfile) that Caddy should use.path/to/Caddyfile
: Represents the file path to the Caddyfile containing the desired server configuration.
Example Output: The server will start using the specific configurations detailed in the Caddyfile, with logs reflecting the custom parameters in action.
Use case 3: Start Caddy in the background
Code:
caddy start
Motivation: Running Caddy in the background allows the server to continue functioning independently from the current terminal session. This is beneficial for deploying applications in a production environment where it is undesirable for a server crash or terminal closure to disrupt service availability.
Explanation:
start
: Instructs Caddy to begin its operations running in the background, making it possible to close the terminal without stopping the server.
Example Output: Upon successful execution, a message will confirm that Caddy is running as a background process, and it will log the process ID for further management actions.
Use case 4: Stop a background Caddy process
Code:
caddy stop
Motivation: This command is essential for managing server resources and operations, especially during maintenance or when updates are required. It allows administrators to halt the background server safely.
Explanation:
stop
: Sends a stop signal to the Caddy process that was initiated withcaddy start
, thereby safely terminating the server.
Example Output: The command will generate a confirmation message indicating that the Caddy server process has been stopped, ensuring no further requests are processed until it is restarted.
Use case 5: Run a simple file server on the specified port with a browsable interface
Code:
caddy file-server --listen :8000 --browse
Motivation: Setting up a simple file server with a browsable interface is incredibly useful for quick and easy sharing of files within a local network. It provides a web-based interface for users to navigate and download available files, ideal for collaborative environments where file distribution is a frequent task.
Explanation:
file-server
: Activates Caddy’s ability to serve files directly over the web.--listen :8000
: Specifies the network port (8000 in this case) to listen for incoming requests.--browse
: Enables a directory listing on the web interface, allowing users to browse the directory’s contents.
Example Output: When accessed in a web browser, the interface displays the files and folders available in the root directory, allowing users to browse and download as needed.
Use case 6: Run a reverse proxy server
Code:
caddy reverse-proxy --from :80 --to localhost:8000
Motivation: Running a reverse proxy server is critical for managing requests to backend services, enhancing security, and improving load distribution. This is particularly useful in microservices architectures where redirecting traffic from external requests to multiple internal services is required.
Explanation:
reverse-proxy
: This sub-command sets up Caddy to function as a reverse proxy server.--from :80
: Specifies the port Caddy listens to for incoming requests (port 80 is standard for HTTP traffic).--to localhost:8000
: Directs these incoming requests to be forwarded to a local service running on port 8000.
Example Output: Upon executing the command, Caddy will handle incoming requests on port 80 and forward them to the specified destination, allowing seamless service communication.
Conclusion:
Caddy offers a versatile and straightforward suite of functionalities that make it an effective choice for various web server applications. Whether for development, file serving, reverse proxying, or running a web server in production, Caddy simplifies setup and execution with its robust and automated features.