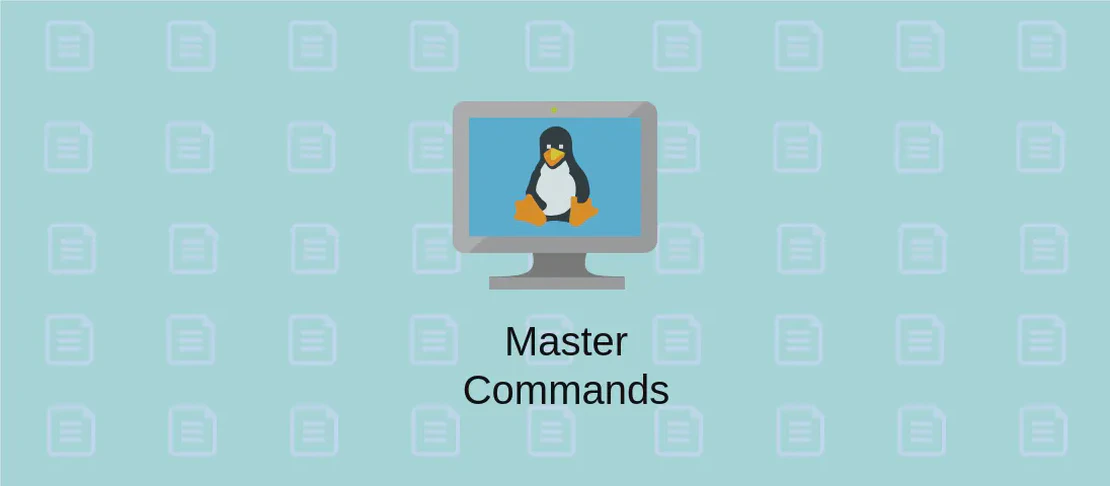
How to use the command 'cake' (with examples)
CakePHP is a rapid development framework for PHP which uses commonly known design patterns like Active Record, Association Data Mapping, Front Controller, and MVC. With built-in tools, CakePHP allows you to logically separate elements of your application and its focused view-controller for your data. The CakePHP framework provides a command-line interface (CLI) tool called cake
, which aids in various development tasks ranging from generating boilerplates to debugging your application effectively. Below are examples illustrating its versatile use cases along with explanations to better understand its utility.
Use case 1: Display basic information about the current app and available commands
Code:
cake
Motivation for using this example:
This command serves as an entry point to interact with CakePHP’s CLI tool. Whether you’re a new developer starting with CakePHP or an experienced one looking to refresh your memory about available commands, using cake
provides a quick overview of the application’s environment and what you can do with CakePHP’s command-line tools.
Explanation:
The command cake
without any arguments displays general information about the current application, like the version in use, the application path, and a list of available commands, which often serve as a handy reminder of what’s possible with CakePHP.
Example output:
Welcome to CakePHP v4.0.0 Console commands:
- cache
- routes
- schema_cache
- server
...
Use case 2: List available routes
Code:
cake routes
Motivation for using this example:
It’s essential to know what routes are available to ensure your application handles user requests properly. Especially useful during debugging or development, listing available routes can help verify that URL routing is implemented correctly within your application.
Explanation:
The routes
command lists all the defined paths and their associated controllers and actions in your application. This allows you to understand and verify the paths users can take through your website.
Example output:
Route collection:
/ (method: GET, POST)
=> Home::index()
/articles/* (method: GET)
=> Articles::view($id)
...
Use case 3: Clear configuration caches
Code:
cake cache clear_all
Motivation for using this example:
Clearing cache is often necessary when configuration changes occur, as old cached values may still be used otherwise. Routine clearing of cache ensures that new configurations are applied correctly, which is crucial for debugging and performance optimization.
Explanation:
The cache clear_all
command clears all the configuration caches, ensuring that your application can reflect new changes immediately without the interference of old, cached data.
Example output:
Cache 'default' cleared.
Cache 'persistent' cleared.
...
Use case 4: Build the metadata cache
Code:
cake schema_cache build --connection connection
Motivation for using this example:
Building the metadata cache is important for enhancing the performance of accessing the database schema information. It helps in reducing repeated SQL queries by storing the schema meta-information in the cache. This process is highly beneficial when the database schema remains relatively static and requires minimal querying.
Explanation:
The schema_cache build --connection connection
command constructs meta-information necessary for your database schema caching. The --connection
option specifies which database connection’s schema cache should be built, allowing precise control over which parts of the application benefit from optimized access.
Example output:
Schema cache build successful for connection 'default'.
...
Use case 5: Clear the metadata cache
Code:
cake schema_cache clear
Motivation for using this example:
At times, the database schema may change, necessitating an updated metadata cache. Clearing old information ensures accurate schema representation and prevents potential errors during application runtime or development.
Explanation:
The schema_cache clear
command removes all the cached database schema meta-information, forcing the system to refresh and possibly rebuild the schema cache when accessed again.
Example output:
Cache 'schema_cache' cleared.
Use case 6: Clear a single cache table
Code:
cake schema_cache clear table_name
Motivation for using this example:
Sometimes you only need to clear the metadata cache for a specific table, especially when incremental schema updates occur or when a specific table’s cache becomes corrupt or outdated.
Explanation:
The schema_cache clear table_name
allows for precise clearing of cache for a specified table, thus focusing on specific database components for faster cache management without affecting other database tables.
Example output:
Schema cache for table 'table_name' cleared.
Use case 7: Start a development web server
Code:
cake server
Motivation for using this example:
Running a development server directly through the command-line interface quickly sets up an environment for you to test changes to your application without a complex local setup. This convenience ensures that applications are always verifiable without dependency concerns.
Explanation:
The server
command initiates a built-in PHP server on the default port (8765) for testing purposes. This command is especially useful for quick testing and local development without needing to configure a full-fledged web server.
Example output:
Built-in server is running in http://localhost:8765/ ...
[Sun Oct 10 10:10:10 2023] PHP 7.4.3 Development Server (http://localhost:8765) started
Use case 8: Start a REPL (interactive shell)
Code:
cake console
Motivation for using this example:
Engaging with PHP interactively allows for executing PHP code snippets directly in a Read-Eval-Print Loop (REPL) environment for testing out commands, debugging, or performing exploratory programming.
Explanation:
The console
command opens an interactive shell to evaluate PHP code on the fly. This is an excellent tool for developers who wish to interact directly with parts of their application or execute database queries live.
Example output:
Welcome to CakePHP Console v4.0.0
>>>
Conclusion:
In conclusion, the command-line interface provided by CakePHP, through the cake
command, offers a powerful set of tools aimed at facilitating various development tasks. These examples illustrate the utility of cake
in managing server operations, caches, routes, and much more, reaffirming its position as an essential tool for CakePHP developers. Leveraging these commands enhances productivity and streamlines the application development process.