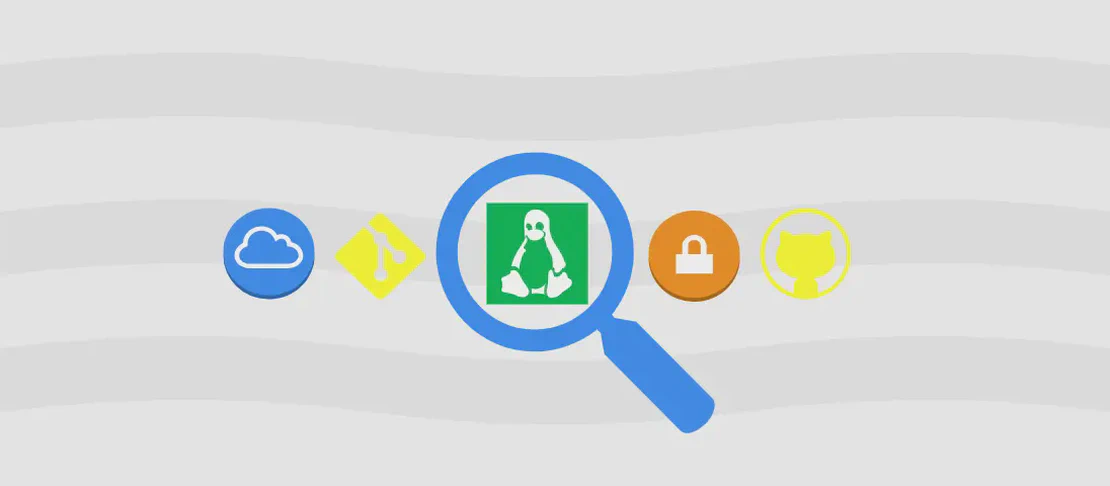
How to Use the Command 'cargo add' (with Examples)
Cargo is the Rust package manager, and it helps manage Rust projects by handling dependencies, building the project, and much more. The cargo add
command is particularly useful within this ecosystem as it enables you to add dependencies to your Rust project’s Cargo.toml
file. This command simplifies dependency management by automatically updating the manifest file with the required dependency details. Let’s explore various use cases and examples of using the cargo add
command.
Add the Latest Version of a Dependency to the Current Project
Code:
cargo add dependency
Motivation: When developing a Rust project, adding third-party crates (libraries) is a common task. Developers may want to integrate multiple external libraries that can speed up development and add rich functionality to their projects. Using the latest version ensures that you benefit from the most recent features and improvements offered by those crates.
Explanation:
When you use cargo add dependency
, the command consults the central crate repository available at crates.io to find and download the latest stable version of the specified dependency. It then inserts this information into your Cargo.toml
manifest file, which serves as the blueprint for your project’s dependencies.
Example Output:
After executing the command, Cargo.toml
will be updated with:
[dependencies]
dependency = "1.2.3" # assuming 1.2.3 is the latest version
Add a Specific Version of a Dependency
Code:
cargo add dependency@version
Motivation: Sometimes new versions of a crate might introduce breaking changes or bugs. Developers may prefer to stick with a version that they have tested and is known to be stable for their use case. In such situations, specifying the version number ensures that the project stays reliable and predictable.
Explanation:
By appending @version
to the command, you instruct Cargo to fetch the particular version of the crate that you trust or require. This is especially useful in larger projects where stability is critical.
Example Output:
If you run the command with cargo add serde@1.0.130
, your Cargo.toml
will be updated to:
[dependencies]
serde = "1.0.130"
Add a Dependency and Enable One or More Specific Features
Code:
cargo add dependency --features feature_1,feature_2
Motivation: Many Rust crates offer optional features which can be included to extend the functionality of the library without affecting the core functionalities. Developers might want to enable only certain aspects of a library to reduce bloat and improve compilation times by explicitly opting into certain features.
Explanation:
The --features
flag allows you to specify which optional features you want to include. By listing each feature separated by commas, you direct Cargo to customize the crate’s integration based on your project’s specific needs.
Example Output:
For cargo add foo --features bar,baz
, Cargo.toml
will show:
[dependencies]
foo = { version = "2.1.0", features = ["bar", "baz"] }
Add an Optional Dependency
Code:
cargo add dependency --optional
Motivation: In some cases, you may want to offer additional features in your library or application that are not mandatory. By marking a dependency as optional, you can conditionally enable its features based on user or build-time configurations.
Explanation:
The --optional
flag marks a dependency as optional in your Cargo.toml
. This converts the dependency into a feature, allowing users to enable it only when needed. It makes your project more flexible and less resource-intensive.
Example Output:
By running this command, Cargo.toml
looks like:
[dependencies]
dependency = { version = "0.1.0", optional = true }
Add a Local Crate as a Dependency
Code:
cargo add --path path/to/crate_directory
Motivation: Using local crates is important when you are developing multiple interdependent projects or working on code that isn’t published to crates.io. It allows you to work on the dependency and the project simultaneously without needing to publish changes to the internet.
Explanation:
The --path
option specifies a local directory path to a crate you want to add as a dependency. Cargo updates the manifest to point to this path instead of fetching the crate from the registry.
Example Output:
If you run cargo add --path ../my_local_crate
, Cargo.toml
will reflect:
[dependencies]
my_local_crate = { path = "../my_local_crate" }
Add a Development or Build Dependency
Code:
cargo add dependency --dev|build
Motivation: Projects often require tools and libraries for testing, debugging, or building that are not needed for the final production code. By categorizing dependencies as development (–dev) or build (–build), developers ensure that they’re only included during the development phase and not bundled into the final package.
Explanation:
The --dev
flag specifies that a dependency should only be used during development (e.g., testing frameworks), while --build
indicates a dependency needed during the build process (e.g., procedural macros).
Example Output:
For cargo add criterion --dev
, Cargo.toml
gets updated with:
[dev-dependencies]
criterion = "0.3.4"
Add a Dependency with All Default Features Disabled
Code:
cargo add dependency --no-default-features
Motivation: Default features of a crate can sometimes include functionalities that are not necessary for your project, leading to larger binaries or unwanted dependencies. Disabling them can result in a lighter and more efficient build, where only the needed features are enabled manually.
Explanation:
The --no-default-features
flag ensures that when you add a dependency, Cargo does not automatically include its default features. You can then manually enable necessary features via the --features
option.
Example Output:
Running cargo add baz --no-default-features
updates Cargo.toml
to:
[dependencies]
baz = { version = "0.8.7", default-features = false }
Conclusion
Mastering the cargo add
command can significantly enhance your Rust project’s efficiency and manageability by simplifying dependency handling. By tailoring each dependency’s integration to your project’s unique needs and constraints, you ensure a more maintainable and resource-efficient codebase. Whether integrating crates from the public domain or local libraries, cargo add
provides a robust toolset for any Rust developer.