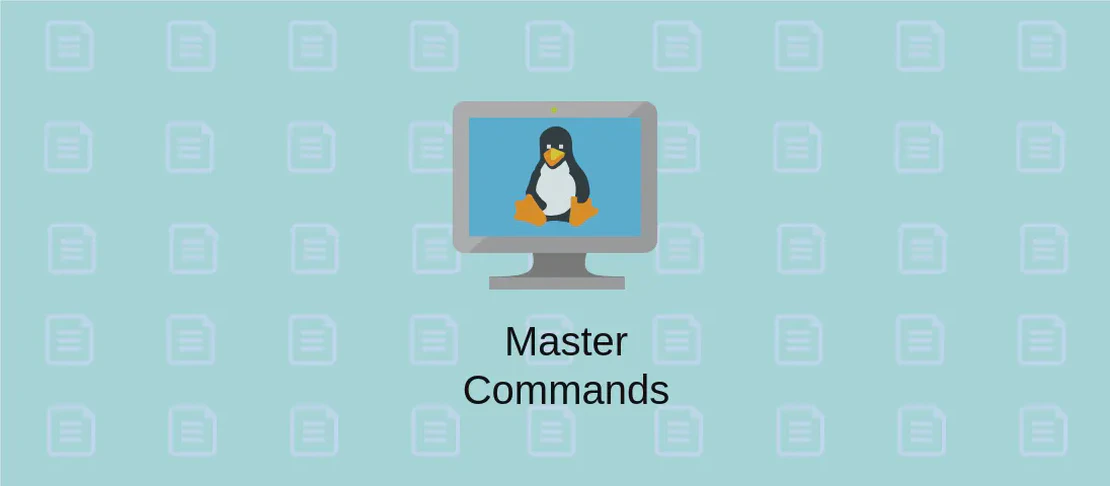
Understanding the 'cargo build' Command (with examples)
In the Rust programming language ecosystem, Cargo is the package manager and build system that developers use to manage, compile, and package their Rust projects. The cargo build
command is a fundamental tool within Cargo, utilized to compile a Rust package and all of its dependencies. This command can significantly streamline the development process by automating the compilation of Rust code based on the configurations set in the Cargo.toml
manifest file. Using cargo build
, developers can easily produce build artifacts necessary for testing, releasing, or running their applications.
Let’s delve into various use cases of the cargo build
command, exploring its different flags and options to understand its versatility and power.
Use Case 1: Build the Package(s) Defined by the Cargo.toml
Manifest File
Code:
cargo build
Motivation:
This is the most fundamental and straightforward way to compile a Rust package. When you run cargo build
without any additional flags or options, it compiles the package(s) specified in the local Cargo.toml
manifest file. This is incredibly useful during the initial stages of development when you want to quickly compile your project to identify any syntax or logical errors.
Explanation:
- The
cargo build
command reads the localCargo.toml
file to determine the package’s configuration and dependencies. - It compiles the specified package along with any dependencies into a binary, which is usually stored in the
target/debug
directory.
Example Output:
Compiling my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 2.34s
Use Case 2: Build Artifacts in Release Mode, with Optimizations
Code:
cargo build --release
Motivation:
During development, you might not care about optimizations and just want your code to compile quickly, which is what the default mode (--debug
) emphasizes. However, when you are preparing your software for production, performance becomes critical. Building in release mode applies optimizations to the compiled code, producing faster and usually smaller binaries. This use case is crucial when you’re ready to showcase your application or deploy it to the end-users.
Explanation:
- The
--release
flag is an option that tells Cargo to compile the package with optimizations, targeting high-performance release builds. - As a result, the artifacts are located in the
target/release
directory rather thantarget/debug
.
Example Output:
Compiling my_project v0.1.0 (/path/to/my_project)
Finished release [optimized] target(s) in 8.56s
Use Case 3: Require that Cargo.lock
is Up to Date
Code:
cargo build --locked
Motivation:
In collaborative projects or continuous integration scenarios, ensuring reproducibility of builds is often necessary. The Cargo.lock
file is used to keep track of the exact versions of dependencies. Sometimes, you want to guarantee that no changes have been made to this file to prevent accidental upgrade of dependencies that could potentially break your build. This command is most beneficial in environments where consistency across builds is paramount.
Explanation:
- The
--locked
flag enforces that theCargo.lock
file must be up to date. - If the
Cargo.lock
file is out of date or missing for dependencies, the build will fail, alerting developers to update it first.
Example Output:
error: the lock file needs to be updated but --locked was passed to prevent this
Use Case 4: Build All Packages in the Workspace
Code:
cargo build --workspace
Motivation:
A workspace in Rust is a collection of one or more packages that share a common Cargo.toml file. This setup makes it easier to manage large projects split into multiple packages. Using the --workspace
flag, you can compile all packages in a workspace together, which is advantageous when testing and validating integrated systems made up of multiple components.
Explanation:
- The
--workspace
flag tells Cargo to compile all the packages and their dependencies listed in the workspace instead of just the default package. - This ensures that every component in the workspace is correctly compiled and ready for testing or deployment.
Example Output:
Compiling package_one v0.1.0 (workspace_path/package_one)
Compiling package_two v0.1.0 (workspace_path/package_two)
Finished dev [unoptimized + debuginfo] target(s) in 4.21s
Use Case 5: Build a Specific Package
Code:
cargo build --package package
Motivation:
In larger projects containing multiple packages, there may be times when you need to focus on a specific part of the project. Compiling a specific package can save time and resources by only building what you are actively working on. This use case is particularly helpful during development and testing of individual components within a larger system.
Explanation:
- The
--package
flag followed by the package name specifies which package to compile in a workspace. - This command directs Cargo to only build the given package and its dependencies, skipping other packages.
Example Output:
Compiling specific_package v0.1.0 (/path/to/specific_package)
Finished dev [unoptimized + debuginfo] target(s) in 1.87s
Use Case 6: Build Only the Specified Binary
Code:
cargo build --bin name
Motivation:
When your project contains multiple binaries, it might be inefficient to build every single one each time. Instead, if you are working on a specific binary, you can compile just that one. This is beneficial for targeting specific parts of your project, which can improve build times and focus on the current development task.
Explanation:
- The
--bin
flag specifies the binary that you want to compile by its name. - This command assumes that you have set up binaries within the
[bin]
section in yourCargo.toml
.
Example Output:
Compiling my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.97s
Use Case 7: Build Only the Specified Test Target
Code:
cargo build --test testname
Motivation:
Automated testing is a cornerstone of modern software development. If you have a suite of tests and need to focus on developing and debugging a particular test, you can compile just that test target. This functionality allows developers to quickly iterate, debug, and improve specific components of their software without the overhead of building unrelated tests.
Explanation:
- The
--test
flag specifies which test target to compile. - The command leverages the test targets defined in the
[[test]]
sections inCargo.toml
.
Example Output:
Compiling my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.57s
Conclusion
The cargo build
command is an essential part of a Rust developer’s toolkit, enabling efficient compilation of Rust projects with ease and precision. By understanding and effectively using its various flags, developers can optimize their build processes to suit diverse project requirements, whether it be development, testing, or deployment. Each use case provided above illustrates the flexibility and adaptability of cargo build
to different stages and aspects of Rust software development.