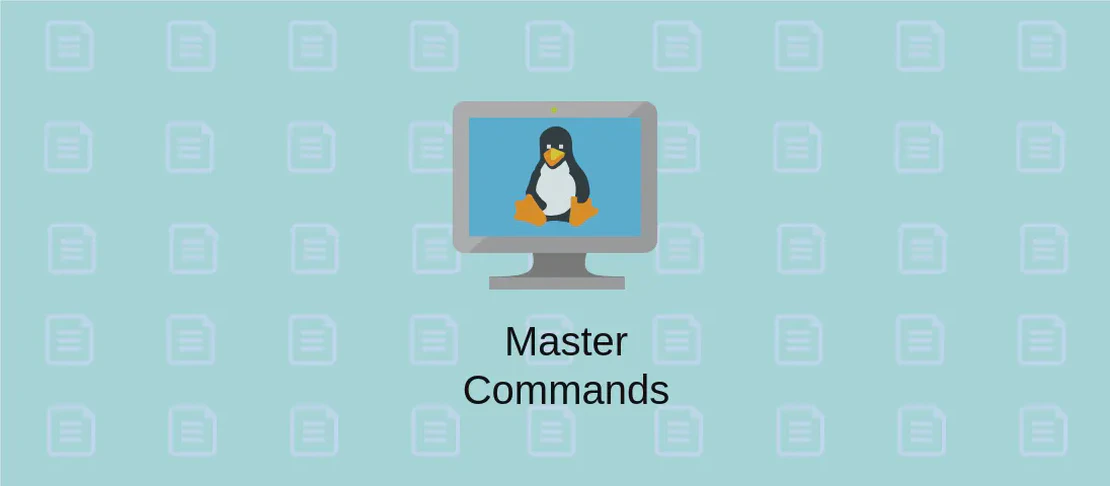
Understanding 'cargo check' in Rust (with examples)
The cargo check
command is a valuable tool in the Rust programming environment. It enables developers to quickly analyze their code for errors without compiling a fully executable binary, which can be a time-consuming process. Essentially, cargo check
can give you feedback on your code’s correctness by examining your package and its dependencies, ensuring that everything is in order before you proceed to the actual compilation. This can significantly speed up development as it provides rapid iteration cycles.
Use case 1: Check the current package
Code:
cargo check
Motivation: Developers often make changes to their code that they want to quickly verify without the overhead of full compilation. Using cargo check
allows a developer to validate that current modifications have not introduced new syntax or type checking errors, thus providing confidence before running a more time-intensive cargo build
.
Explanation:
cargo check
: This is the base command that checks for errors in the current Rust package you are working on. It inspects your code without actually producing compiled artifacts.
Example output:
Checking my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.27s
This output indicates that all files in the current package have been checked successfully, and no errors were found.
Use case 2: Check all tests
Code:
cargo check --tests
Motivation: Tests are an essential part of software development, ensuring the code is functioning as expected. Running cargo check --tests
allows a developer to confirm that all the test code in their project is syntactically and semantically correct before executing them, ensuring that they won’t fail due to basic compilation issues.
Explanation:
cargo check
: The base command for checking code.--tests
: This flag includes all test code in the checking process, ensuring that all components of your codebase are free from errors.
Example output:
Checking my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.34s
This means the check ran through all the test code, and no errors were found.
Use case 3: Check the integration tests in tests/integration_test1.rs
Code:
cargo check --test integration_test1
Motivation: Often, integration tests are kept in separate files to verify that different components of a project work together as expected. By running cargo check --test integration_test1
, a developer can focus on a specific integration test file, ensuring it’s ready to be executed without syntax or type errors.
Explanation:
cargo check
: The base command for checking code.--test integration_test1
: This specifies that only the filetests/integration_test1.rs
should be checked for errors, allowing a more targeted approach, especially useful in large projects with many test files.
Example output:
Checking my_project v0.1.0 (/path/to/my_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.15s
This feedback suggests the specific integration test file integration_test1.rs
is free from errors.
Use case 4: Check the current package with the features feature1
and feature2
Code:
cargo check --features feature1,feature2
Motivation: Features in Rust allow for conditional compilation, where different parts of the code might be compiled and checked based on the chosen features. Using cargo check --features
, a developer can validate the code’s correctness under these specific feature conditions, ensuring that all feature-dependent paths are error-free.
Explanation:
cargo check
: The standard checking procedure.--features feature1,feature2
: This flag tells Cargo to considerfeature1
andfeature2
as active, thus including the code and dependencies associated with those features in the checking process.
Example output:
Checking my_project v0.1.0 (/path/to/my_project) with features ["feature1", "feature2"]
Finished dev [unoptimized + debuginfo] target(s) in 0.24s
This shows that the project, with feature1
and feature2
active, has no errors.
Use case 5: Check the current package with default features disabled
Code:
cargo check --no-default-features
Motivation: By default, Rust projects often come with a set of enabled features. However, there might be scenarios where a developer needs to ensure that the code works with those default features turned off, perhaps when deploying a minimalistic version of the package.
Explanation:
cargo check
: The starting point for error checking.--no-default-features
: This flag effectively disables any default features that might otherwise automatically come into play, checking the codebase under the assumption that none of those default features are active.
Example output:
Checking my_project v0.1.0 (/path/to/my_project) without default features
Finished dev [unoptimized + debuginfo] target(s) in 0.22s
This confirms that the project checks out correctly without the aid of any default features.
Conclusion:
Using cargo check
is a powerful way to ensure code correctness throughout various stages of development in Rust. By understanding and leveraging its different options — such as testing, feature-specific checks, and default feature exclusions — a developer can streamline the process of error-checking and focus on writing effective, bug-free code.