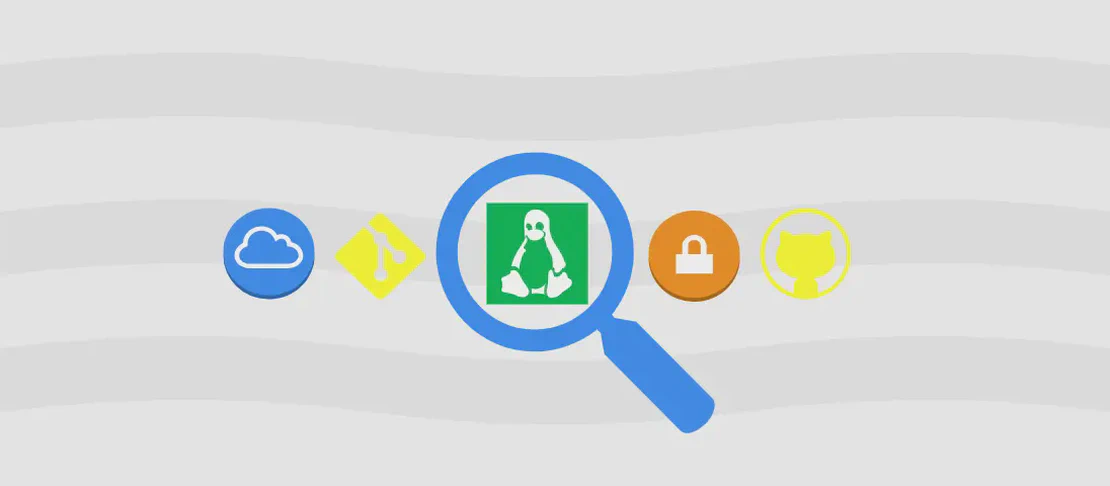
Mastering 'cargo clippy' (with examples)
cargo clippy
is a tool used in the Rust programming language ecosystem to catch common mistakes and improve code quality. It offers a wide range of lints, each helping detect different common errors or areas for improvement in your Rust code. Whether you are a beginner or an experienced Rust developer, cargo clippy
can help ensure that your code adheres to best practices.
Use case 1: Run checks over the code in the current directory
Code:
cargo clippy
Motivation:
Using cargo clippy
without any additional arguments is the most straightforward way to check the current project for potential issues. It helps ensure that your code is robust and adheres to commonly accepted standards and practices in Rust programming. This basic usage is particularly helpful for quickly identifying improvements in your ongoing work within the current directory.
Explanation:
cargo clippy
: The command itself operates on the current directory by default, without further need for specification. It analyzes the Rust code present and highlights any problems it identifies.
Example output:
warning: needless borrow
--> src/main.rs:15:14
|
15| let x = &y;
| ^^
|
= note: `#[warn(clippy::needless_borrow)]` on by default
warning: 1 warning emitted
Use case 2: Require that Cargo.lock
is up to date
Code:
cargo clippy --locked
Motivation:
This command checks that the Cargo.lock
file, which records the exact versions of dependencies used, is up to date. Ensuring that Cargo.lock
is current boosts project reproducibility by locking dependencies to specific versions, reducing discrepancies between different environments or team members.
Explanation:
--locked
: This flag signals thatcargo clippy
should only proceed if theCargo.lock
file is up to date. It prevents the command from updating the lock file automatically, thus ensuring consistency in dependency management.
Example output:
error: the lock file /path/to/your/project/Cargo.lock needs to be updated but --locked was passed to prevent this
Use case 3: Run checks on all packages in the workspace
Code:
cargo clippy --workspace
Motivation:
Running checks across all packages in a workspace is crucial for large projects where multiple interdependent packages are developed in tandem. This ensures that each package within the workspace adheres to coding standards and best practices, maintaining the overall quality and integrity of the entire codebase.
Explanation:
--workspace
: This instructscargo clippy
to analyze every package defined within the workspace, rather than just a singular package or project.
Example output:
warning: 3 warnings emitted in 'package1'
warning: 2 warnings emitted in 'package2'
Use case 4: Run checks for a package
Code:
cargo clippy --package package_name
Motivation:
Running cargo clippy
for a specific package is vital when your workspace contains multiple packages, but you need to focus quality checks on just one package. This helps target specific areas of your project that require improvements or adherence checks without overhead from other packages.
Explanation:
--package package_name
: This flag precisely specifies which package within your workspace you want to check. Replacepackage_name
with the actual name of your package.
Example output:
warning: redundant clone
--> src/lib.rs:34:10
|
34| let z = foo.clone();
| ^^^^^^^^^^^^ help: remove this
Use case 5: Run checks for a lint group
Code:
cargo clippy -- --warn clippy::complexity
Motivation:
Applying checks for a specific lint group allows developers to focus on particular areas of code quality, such as reducing complexity, improving performance, or enhancing safety. By specifying a lint group, you can tailor the feedback to support your current objectives in code optimization or compliance with coding standards.
Explanation:
--
: This marks the end of options for thecargo clippy
command itself, followed by options that are passed directly to the underlying Rust compiler.--warn clippy::complexity
: This specifies that thecomplexity
lint checks should be highlighted as warnings.
Example output:
warning: function can be written in a simpler way
--> src/main.rs:42:5
|
42| let result = if condition { true } else { false };
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
|
= note: `#[warn(clippy::if_not_else)]` on by default
Use case 6: Treat warnings as errors
Code:
cargo clippy -- --deny warnings
Motivation:
Treating warnings as errors is particularly helpful for enforcing quality control measures. It pushes developers to fix every warning produced, ensuring their code doesn’t just run without errors but also without warnings, thus being even more polished and adhering strictly to best practices.
Explanation:
--
: Ends thecargo clippy
options, allowing the next options to be applicable to the internal Rust compiler processes.--deny warnings
: Converts warnings generated byclippy
into errors, making them mandatory to fix before proceeding.
Example output:
error: unused variable: `x`
--> src/main.rs:9:9
|
9 | let x = 5;
| ^ help: consider using the variable
Use case 7: Run checks and ignore warnings
Code:
cargo clippy -- --allow warnings
Motivation:
In some contexts, you might prioritize rapid iteration or feature development over strict adherence to every warning issued by cargo clippy
. Ignoring warnings can facilitate prototyping and experimentation by reducing disruptions from relatively minor issues that don’t impede functionality.
Explanation:
--
: Passes through options forcargo clippy
to the Rust compiler itself.--allow warnings
: Instructsclippy
to allow warnings without treating them as errors, thereby enabling more leniency during development cycles.
Example output:
The code compiles successfully with existing warnings.
Use case 8: Apply Clippy suggestions automatically
Code:
cargo clippy --fix
Motivation:
Automatically applying clippy
suggestions can significantly streamline the process of code improvement and maintenance. This use case is especially beneficial during mass refactoring tasks or when onboarding codebases with a large stack of warnings and suggestions.
Explanation:
--fix
: This flag allowsclippy
to not only analyze the code but also apply any feasible suggestions it sees fit, automating the task of making small but meaningful improvements.
Example output:
Applied 10 suggestions.
Conclusion:
The cargo clippy
command is a versatile tool in the Rust ecosystem, aiding developers at every level in writing more efficient, cleaner, and error-free code. Each use case described above provides unique benefits depending on the context in which it is applied, from individual package checks to workspace-wide analyses, ensuring that Rust developers can maintain high coding standards. Whether choosing to enforce strict compliance by treating warnings as errors or taking a more flexible approach by ignoring them, cargo clippy
caters to diverse development needs.