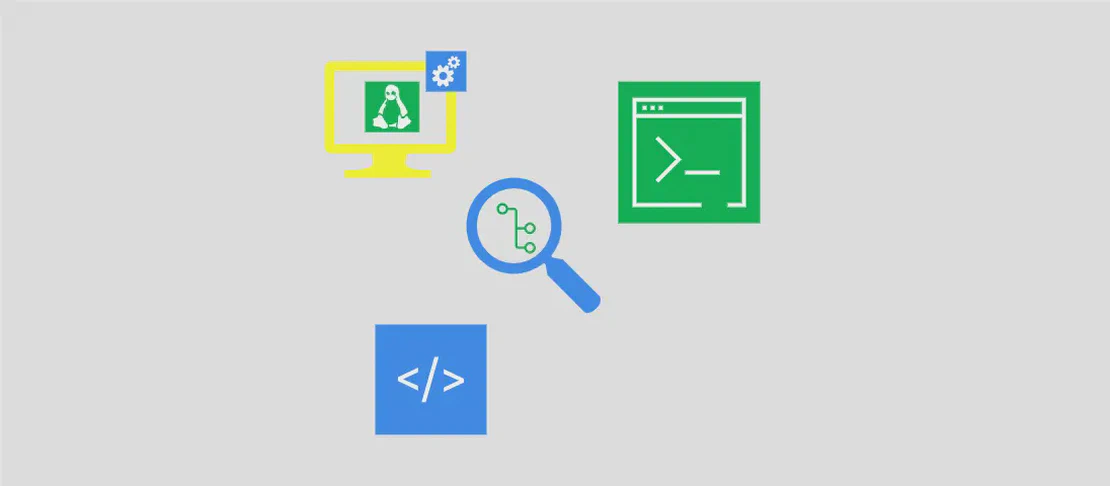
How to use the command cargo (with examples)
Cargo is a command-line tool for managing Rust projects and their dependencies. It provides several subcommands that perform different tasks related to Rust projects. This article will illustrate different use cases of the cargo
command with examples.
Use case 1: Search for crates
Code:
cargo search search_string
Motivation: The cargo search
command allows users to search for crates (Rust libraries) available on the official crates.io registry. This helps developers find and discover existing libraries that they can use in their Rust projects.
Explanation:
search_string
is the text to be searched. It can be a crate name or any keyword related to the desired crate.
Example output:
criterion (0.3.0) - Statistical tracking and benchmarking
libc (0.2.88) - A low-level library to use libc functionality in Rust
Use case 2: Install a binary crate
Code:
cargo install crate_name
Motivation: The cargo install
command is used to install binary crates. Installing a binary crate makes the corresponding executable available for use on the system.
Explanation:
crate_name
is the name of the crate to be installed.
Example output:
Updating crates.io index
Installing diesel_cli v1.4.4 Running `...`
Compiling ...
...
Installing ...
Use case 3: List installed binary crates
Code:
cargo install --list
Motivation: The cargo install --list
command provides a list of all binary crates that have been installed using cargo install
. This is useful for managing installed crates and verifying their presence on the system.
Explanation: The --list
flag is used to signal that we want to list the installed binary crates.
Example output:
diesel_cli v1.4.4
sqlx-cli v0.5.7
Use case 4: Create a new binary or library Rust project
Code:
cargo init --bin|lib path/to/directory
Motivation: The cargo init
command is used to create a new Rust project. This command generates the required files and directories to start a new project, saving developers from the hassle of setting things up manually.
Explanation:
--bin|lib
specifies whether the project should be a binary or library project.path/to/directory
specifies the directory in which the project should be created. If not provided, the command will use the current working directory.
Example output:
Created binary (application) `my_app` package
Use case 5: Add a dependency to Cargo.toml
Code:
cargo add dependency
Motivation: The cargo add
command allows users to easily add a dependency to their Rust projects. This command updates the Cargo.toml
file and automatically fetches and includes the specified dependency in the project.
Explanation:
dependency
is the name of the crate to be added as a dependency.
Example output:
Updating crates.io index
Adding reqwest v0.11.4 to dependencies in Cargo.toml
Use case 6: Build the Rust project using the release profile
Code:
cargo build --release
Motivation: The cargo build
command compiles a Rust project and produces the corresponding binary. Building with the --release
flag enables optimizations for performance, producing a more optimized and faster binary.
Explanation:
--release
flag instructs the build process to use the release profile.
Example output:
Compiling my_app v0.1.0 (/path/to/my_app)
Finished release [optimized] target(s) in 3.41s
Use case 7: Build the Rust project using the nightly compiler
Code:
cargo +nightly build
Motivation: The cargo +nightly build
command is used to build a Rust project using the nightly version of the Rust compiler. This allows developers to use features and enhancements that are not yet available in the stable Rust release.
Explanation:
+nightly
specifies that the nightly version of the Rust compiler should be used for building the project.
Example output:
Compiling my_app v0.1.0 (/path/to/my_app)
Finished dev [unoptimized + debuginfo] target(s) in 2.88s
Use case 8: Build using a specific number of threads
Code:
cargo build --jobs number_of_threads
Motivation: The cargo build
command utilizes multiple threads by default to speed up the build process. However, there may be cases where the number of threads can be customized to optimize resource usage or manage build times.
Explanation:
--jobs number_of_threads
allows users to specify the number of threads to be used for building the project.
Example output:
Compiling my_app v0.1.0 (/path/to/my_app)
Finished dev [unoptimized + debuginfo] target(s) in 5.88s
Conclusion:
The cargo
command is an essential tool for managing Rust projects. It provides convenient features for searching crates, installing binary crates, creating new projects, adding dependencies, and building projects with various configurations. Utilizing these features can greatly enhance the development experience with Rust.