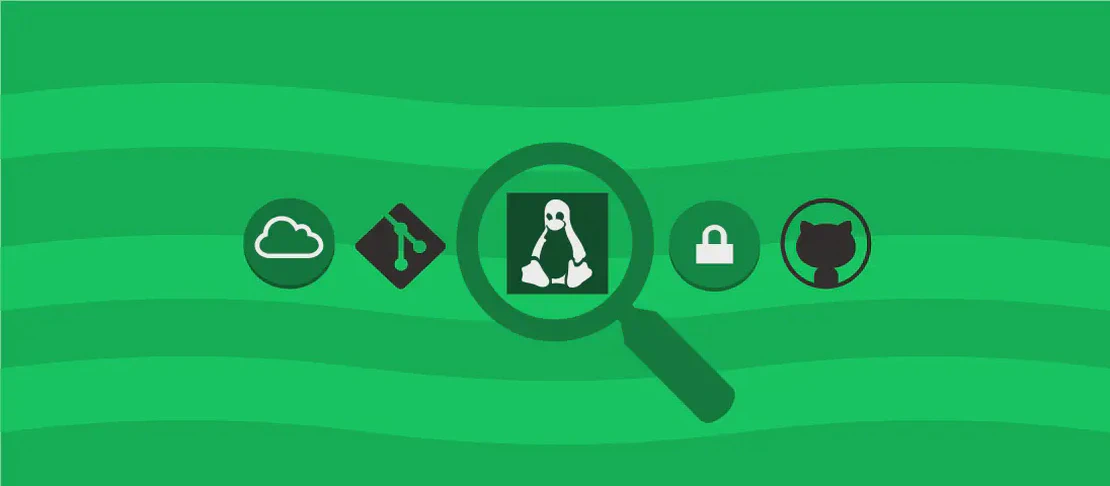
How to use the command 'cargo fix' (with examples)
Cargo is the package manager for Rust. The ‘cargo fix’ command is used to automatically fix lint warnings reported by ‘rustc’, the Rust compiler. This command analyzes the code and applies the necessary changes to fix the reported issues. It is a convenient tool to maintain code quality and ensure adherence to best practices.
Use case 1: Fix code even if it already has compiler errors
Code:
cargo fix --broken-code
Motivation: The ‘–broken-code’ flag allows ‘cargo fix’ to attempt fixing the code even if it has compiler errors. This can be useful when working with a large codebase that has multiple errors. By applying fixes to the code, it may help to reveal additional issues that are not apparent due to the existing errors.
Explanation: The ‘–broken-code’ flag tells ‘cargo fix’ to fix the code even if there are existing compiler errors. This flag overrides the default behavior of ‘cargo fix’ which skips files with errors. It allows the command to make improvements to the codebase despite the presence of errors.
Example output: If there are existing compiler errors, ‘cargo fix’ will display the warnings and errors reported by ‘rustc’ while attempting to apply the necessary fixes. The output will show a summary of the fixes made and any remaining issues.
Use case 2: Fix code even if the working directory has changes
Code:
cargo fix --allow-dirty
Motivation: The ‘–allow-dirty’ flag enables ‘cargo fix’ to fix the code even if the working directory has uncommitted changes. This is beneficial when a developer wants to apply fixes to their code without the need to commit changes immediately.
Explanation: The ‘–allow-dirty’ flag instructs ‘cargo fix’ to proceed fixing the code even if the working directory has modifications. By default, ‘cargo fix’ requires a clean working directory to avoid accidentally losing changes. The flag relaxes this requirement and allows fixing the code with pending changes.
Example output: If there are uncommitted changes in the working directory, ‘cargo fix’ will display a warning about the dirty state. It will provide a summary of the changes made and any remaining issues that require manual intervention.
Use case 3: Migrate a package to the next Rust edition
Code:
cargo fix --edition
Motivation: The ‘–edition’ flag is used to migrate a package to the next Rust edition. Rust introduces new language features and improvements in each edition. By migrating to a new edition, developers can take advantage of these new features and maintain compatibility with the latest Rust language version.
Explanation: The ‘–edition’ flag tells ‘cargo fix’ to apply the necessary changes to migrate the package to the next Rust edition. The command analyzes the codebase and updates code in accordance with the changes made in the new edition. This includes adjusting syntax, incorporating new APIs, and handling deprecations.
Example output: When the ‘–edition’ flag is used, ‘cargo fix’ will display the changes made to migrate the codebase to the next Rust edition. It will provide a summary of the modifications made and any remaining issues that require manual attention.
Use case 4: Fix the package’s library
Code:
cargo fix --lib
Motivation: The ‘–lib’ flag allows ‘cargo fix’ to specifically target the package’s library for fixing. This can be useful when there are multiple target components in the package, and the focus is solely on the library code.
Explanation: The ‘–lib’ flag tells ‘cargo fix’ to fix the code in only the package’s library component. By default, ‘cargo fix’ analyzes and applies changes to the entire codebase, including tests and other target components. The flag narrows the scope to only the library code.
Example output: When the ‘–lib’ flag is used, ‘cargo fix’ will display the changes made to fix the package’s library. It will provide a summary of the modifications applied and any remaining issues that require manual intervention.
Use case 5: Fix the specified integration test
Code:
cargo fix --test name
Motivation: The ‘–test’ flag enables ‘cargo fix’ to fix a specific integration test file. This is useful when there are multiple integration tests, and the goal is to apply fixes to a specific test case.
Explanation: The ‘–test name’ flag instructs ‘cargo fix’ to fix the specified integration test file identified by ’name’. By default, ‘cargo fix’ applies changes to all integration tests in the codebase. But with this flag, the command will only target the specified test file, making it convenient for targeted fixes.
Example output: When the ‘–test name’ flag is used, ‘cargo fix’ will display the changes made to fix the specified integration test file. It will provide a summary of the modifications applied and any remaining issues that require manual attention.
Use case 6: Fix all members in the workspace
Code:
cargo fix --workspace
Motivation: The ‘–workspace’ flag allows ‘cargo fix’ to fix all members in a workspace. A workspace is a set of packages managed by a single ‘Cargo.toml’ file. By fixing all members in the workspace, developers can ensure consistent code quality and apply fixes to multiple packages simultaneously.
Explanation: The ‘–workspace’ flag tells ‘cargo fix’ to fix all members in the workspace. By default, ‘cargo fix’ only fixes the package where the command is executed. With this flag, ‘cargo fix’ traverses all members of the workspace and applies the necessary fixes to each package.
Example output: When the ‘–workspace’ flag is used, ‘cargo fix’ will display the changes made to fix each member of the workspace. It will provide a summary of the modifications applied to each package and any remaining issues that require manual intervention.
Conclusion:
The ‘cargo fix’ command is a powerful tool for automatically fixing code issues reported by ‘rustc’. It provides a convenient way to improve code quality, apply changes to migrate to new Rust editions, and fix specific target components. Understanding the various use cases with their respective flags enables developers to make the most of this command and maintain a high-quality Rust codebase.