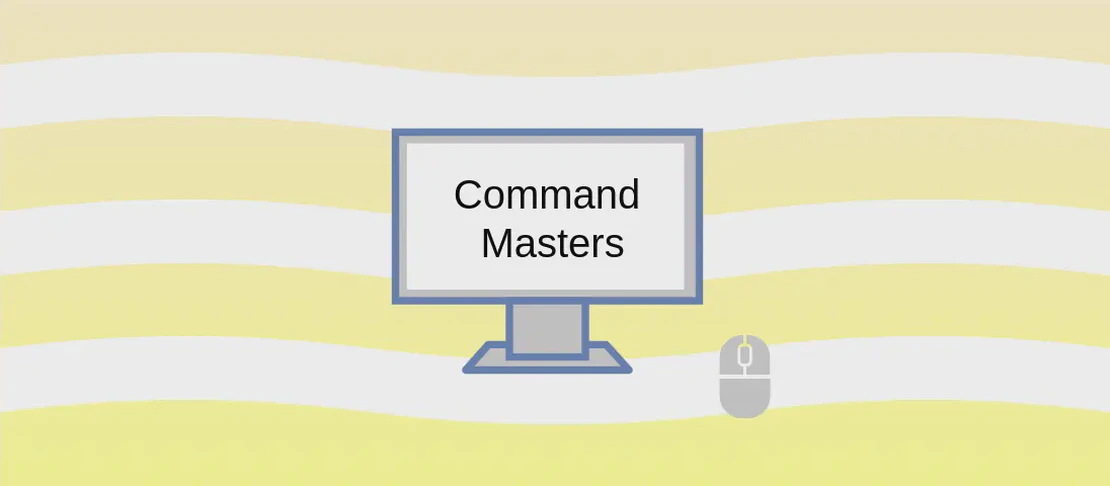
How to Use the Command 'cargo fmt' (with Examples)
The cargo fmt
command is a powerful tool in the Rust programming language ecosystem, dedicated to formatting your Rust code. With the help of rustfmt
, the command ensures that your code adheres to a consistent style, making it more readable and maintainable. This tool is particularly valuable as it automates the formatting process, reducing the cognitive load of developers and helping teams maintain a uniform code style across large projects.
Use Case 1: Format All Source Files
Code:
cargo fmt
Motivation:
When working on collaborative projects, it is crucial to maintain a clean and consistent codebase. Using cargo fmt
helps achieve this by automatically formatting all source files in your Rust project according to the community’s idiomatic/official style guidelines. This reduces unnecessary formatting changes in code reviews, allowing developers to focus on the functionality and logic rather than style issues. It is an efficient practice, especially in large projects with multiple contributors, to execute this command regularly before committing changes.
Explanation:
cargo fmt
: This command, without any additional arguments, appliesrustfmt
to all Rust source files in the current project.rustfmt
itself is a tool specifically designed to format Rust code according to predefined style guidelines. By executingcargo fmt
, every source file (.rs) in the current project will be scanned, and the code within these files will be reformatted to adhere to the recognized style standards. This promotes code cleanliness and uniformity across the project.
Example Output:
Execution of cargo fmt
typically does not produce console output unless there is an error or a significant change logged. In most cases, it will silently reformat the code files.
Use Case 2: Check for Formatting Errors Without Writing to Files
Code:
cargo fmt --check
Motivation:
In some cases, you might want to verify that your project’s code is formatted according to the standard guidelines without applying any changes. This is especially useful in continuous integration (CI) pipelines, where you want to ensure code style compliance before allowing changes into main branches. The --check
flag allows developers to identify formatting deviations as part of automated testing, preventing inconsistent styling from being merged into the version control system. This safeguard supports an organized codebase and reduces the manual effort needed during code reviews.
Explanation:
--check
: This flag instructscargo fmt
to runrustfmt
in a “check” mode. Instead of modifying the files, it only checks if they are already formatted according to the defined style. If any files are not properly formatted,cargo fmt --check
will exit with a non-zero status code, providing feedback about which files need reformatting without altering them.
Example Output:
Executing cargo fmt --check
may produce output similar to the following if there are unformatted files:
Diff in path/to/file.rs at line ...
Diff in another/path/file.rs at line ...
If all files are formatted correctly, there will be no output or a simple message indicating compliance.
Use Case 3: Pass Arguments to Each rustfmt
Call
Code:
cargo fmt -- --edition 2021
Motivation:
rustfmt
offers a variety of configuration options that allow you to tailor its behavior to specific project needs. You might want to pass custom arguments to rustfmt
for specialized formatting based on different project requirements, or updating the code to a newer edition of Rust. For instance, the --edition
argument can be used to apply formatting rules relevant to a specific edition of Rust. This use case is critical when transitioning projects between Rust editions or when integrally adapting new formatting standards specific to particular code structures or syntax variations introduced over different Rust releases.
Explanation:
--
: This separator indicates tocargo fmt
that any subsequent arguments are meant forrustfmt
itself. It signals the end of arguments intended forcargo
and the beginning of arguments forrustfmt
.--edition 2021
: This argument tellsrustfmt
to format the source code according to the rules of the 2021 edition of Rust. Each edition of Rust may introduce changes to idiomatic styling, so this can ensure that your codebase conforms to the appropriate standards of the chosen edition.
Example Output:
Running cargo fmt
with additional rustfmt
arguments should typically not yield any output unless specific issues arise. A successful run will mean your code is updated according to the specified rustfmt
options.
Conclusion
The cargo fmt
command is an essential tool for Rust developers seeking a streamlined and efficient code formatting process. By automating the adherence to style guidelines, it promotes readability, maintainability, and consistency across projects. Whether you are enforcing formatting standards in a team environment, ensuring compliance through CI tools, or customizing your formatting for specific scenarios, cargo fmt
offers valuable functionalities to suit your needs.