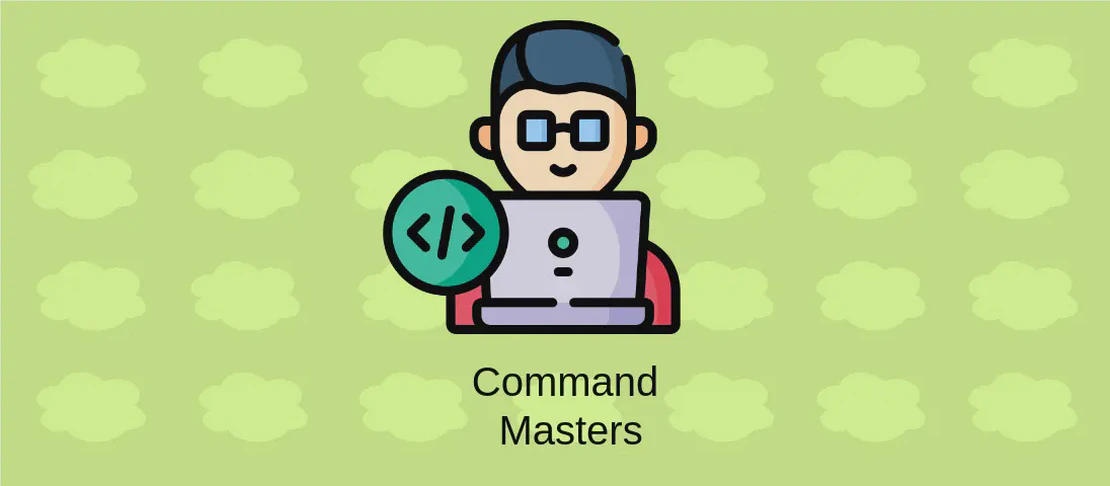
How to Use the Command 'cargo help' (with examples)
Cargo is the Rust package manager and build system. The cargo
command provides various functionalities to manage and create Rust projects, resolve dependencies, compile packages, and build documentation, among others. The command cargo help
is a tool that assists users by providing detailed information on how to use Cargo and its multitude of subcommands, which can range from building projects to running tests and more. This command is particularly useful for both new and experienced Rust developers as it serves as an on-demand reference guide.
Use Case 1: Display General Help
Code:
cargo help
Motivation:
In any comprehensive command-line tool like Cargo, users often need an overview of all the functionalities that the tool offers. Running cargo help
is the quickest way to get a general understanding of what commands are available and what each command’s purpose is. This is particularly important for new users who might not be familiar with all the capabilities of Cargo or for experienced users who want to refresh their memory about available options.
Explanation:
cargo
: This is the main command for interacting with Rust’s package manager and build system.help
: This subcommand is used to display documentation that outlines the overall functionality of Cargo and its various subcommands. No additional arguments or flags are needed to execute this command.
Example Output:
When running cargo help
, users are typically presented with a structured and categorized list of available Cargo subcommands. It provides a brief description of what each command does, such as:
Cargo is a package manager and build system for Rust.
Usage:
cargo [OPTIONS] [SUBCOMMAND]
Options:
-V, --version Print version info and exit
--list List installed commands
-h, --help Print this message and exit
Commonly used cargo commands:
build Compile the current package
check Analyze the current package and report errors, but don't build object files
clean Remove the target directory
doc Build this package's and its dependencies' documentation
new Create a new cargo package
run Run a binary or example of the local package
See 'cargo help <command>' for more information on a specific command.
Use Case 2: Display Help for a Subcommand
Code:
cargo help build
Motivation:
As a developer becomes more acquainted with Cargo, they might need to delve deeper into specific subcommands to make full use of their features. For example, understanding all the options available for the cargo build
subcommand can optimize the way you compile your projects. By using cargo help build
, users can get detailed information about the build
subcommand, which might include options for specifying target directories, release modes, and various flags that could influence the output of their build process. This targeted help is crucial for optimizing workflows and ensuring that the user utilizes the command to its fullest potential.
Explanation:
cargo
: The primary command for Rust’s package management and build system.help
: A subcommand to access detailed documentation and usage examples associated with Cargo.build
: A specific subcommand of Cargo for which help is being requested. Thebuild
command is used to compile the current project, and by appending it tocargo help
, you ask for detailed guidance on its various options and functionalities.
Example Output:
When executing cargo help build
, the output will provide a detailed breakdown of the build
subcommand, including its purpose and how to customize its behavior:
Compile the current project.
Usage:
cargo build [OPTIONS]
Options:
-p, --package <SPEC>... Package(s) to build
--release Build artifacts in release mode, with optimizations
--target <TRIPLE> Build for the target triple
--all Build all packages in the workspace
--exclude <SPEC>... Exclude packages from the build
Some additional options and flags might be displayed based on the user's Cargo version and any custom subcommands they may have installed. The detailed explanation of these flags aids in efficiently configuring the build process for specific needs, such as targeting a different architecture or opting for a release build over a debug build.
## Conclusion:
The `cargo help` command and its variations are invaluable tools for developers working with Rust. Whether you're looking for an overview of what Cargo can do or need detailed instructions on a specific subcommand, using `cargo help` equips you with the necessary knowledge to navigate and leverage the full spectrum of Cargo's capabilities effectively. It is a quintessential part of the Rust developer's toolkit, ensuring that help is always just a command away.