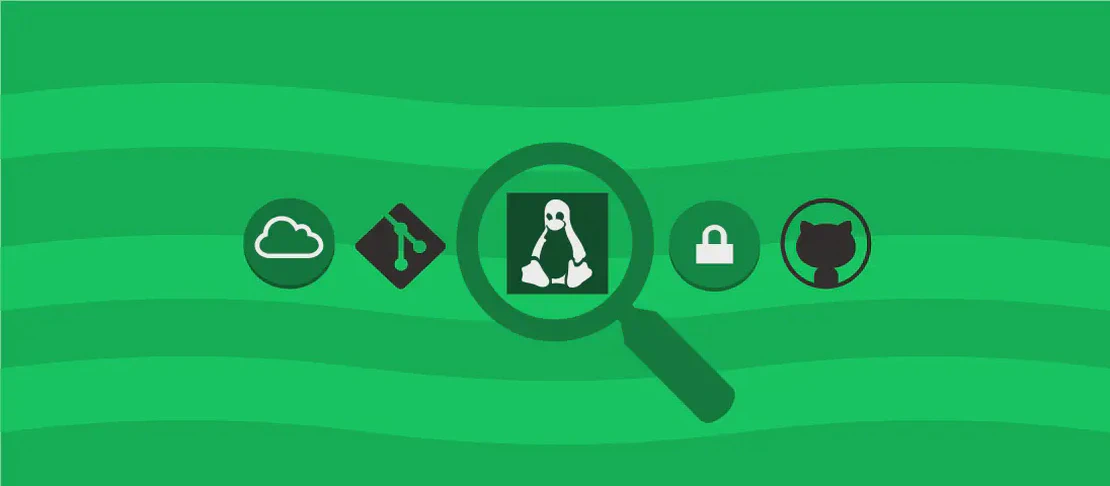
How to Use the Command 'cargo init' (with examples)
The cargo init
command is an essential tool for developers working with the Rust programming language. It is used to initialize a new Cargo package, which is the standard unit of software distribution in Rust. This command allows you to quickly set up a new Rust project with options to define the project’s type as either a binary or a library, integrate with version control systems, and customize the package name. Whether you are starting a new project from scratch or organizing code into a library, cargo init
provides the foundational setup to commence Rust development efficiently. Below are various use cases to illustrate the functionality and versatility of the cargo init
command.
Use case 1: Initialize a Rust project with a binary target in the current directory
Code:
cargo init
Motivation:
Initializing a Rust project with a binary target is a common starting point for many developers. A binary target is typically used when the goal is to create an executable program. By running cargo init
in the current directory, you can instantly set up the necessary structure and configuration files to start building your application. This saves time and ensures that you are using the standard directory layout and conventions recommended by the Rust community.
Explanation:
The command cargo init
without any additional arguments initializes a new Cargo package in the current directory with the default configuration. The package is set up as a binary crate, meaning it includes an entry point (main.rs
) from which your application can be executed. It also creates a Cargo.toml
file that acts as the manifest for project metadata and dependencies management.
Example Output:
Created binary (application) package
Use case 2: Initialize a Rust project with a binary target in the specified directory
Code:
cargo init path/to/directory
Motivation:
You might want to set up a new Rust project in a specific directory rather than the current one. This could be motivated by organizational preferences or because your project structure requires different segments saved separately. Running cargo init
with a path allows you to specify exactly where you want the project initialized, enforcing consistency and organization in your file system.
Explanation:
By providing a path (path/to/directory
), this command initializes a new binary Rust package in the specified directory. Like the default behavior, it creates a Cargo.toml
and a main.rs
file necessary for running and managing the package. If the directory does not exist, it will automatically be created.
Example Output:
Created binary (application) package at path/to/directory
Use case 3: Initialize a Rust project with a library target in the current directory
Code:
cargo init --lib
Motivation:
When the intent is to develop a library rather than a standalone executable, initializing a project as a library package is essential. Libraries do not have a main
function; instead, they expose functions and modules for other applications to use. Using cargo init --lib
helps form the skeleton of a reusable Rust library quickly, staying true to idiomatic Rust programming practices.
Explanation:
The --lib
flag modifies the behavior of cargo init
to initialize the package as a library crate. Instead of creating a main.rs
file, it creates a lib.rs
file, which serves as the main point of entry for the library’s functionality.
Example Output:
Created library package
Use case 4: Initialize a version control system repository in the project directory (default: git
)
Code:
cargo init --vcs git|hg|pijul|fossil|none
Motivation:
Leveraging version control is crucial for software development as it tracks changes, facilitates collaboration, and archives the development history. By default, cargo init
sets up a git repository, but you can specify other systems like Mercurial (hg
), Pijul, Fossil, or none at all. This ensures that your project seamlessly integrates with your preferred version control system right from the start.
Explanation:
The --vcs
argument specifies which version control system to initialize in the project directory. The option git
initializes a Git repository (default behavior), hg
for Mercurial, pijul
, fossil
, or none
if no version control system is desired.
Example Output (for git):
Initialized `git` repository
Use case 5: Set the package name (default: directory name)
Code:
cargo init --name name
Motivation:
Setting a specific package name is vital when the directory name does not accurately represent the package’s purpose or when a descriptive, unique name is preferred for publication to crates.io, the Rust community’s package registry. This can be crucial for branding, recognition, and avoiding naming conflicts with existing packages.
Explanation:
The --name
argument allows you to define a custom name for your package, different from the directory name. This customization impacts the Cargo.toml
file, where the name specified is included as the package
name.
Example Output:
Created binary (application) package with name `name`
Conclusion:
The cargo init
command is a versatile and powerful tool within the Rust ecosystem that simplifies starting new projects, ensuring consistency, and integrating early with version control systems. By understanding and utilizing its various options, developers can tailor their project initialization to specific needs, paving the way for structured and efficient Rust development. Whether you’re initiating a binary application or a library, with or without version control, cargo init
emerges as an indispensable CPU-saver for your coding endeavors.