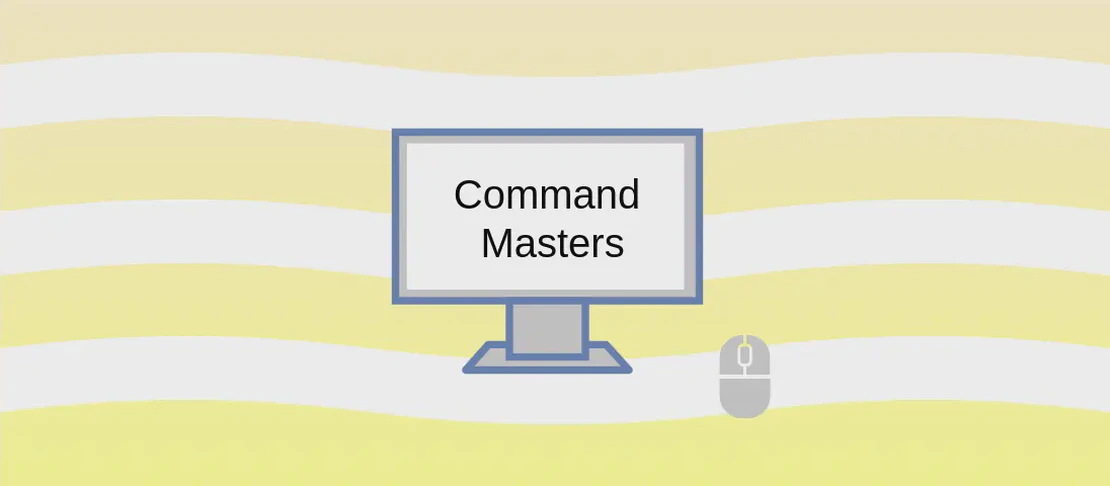
How to Use the Command 'cargo install' (with Examples)
Cargo is the Rust package manager, which serves as a vital part of the workflow for building and managing Rust projects. The cargo install
command is primarily used to build and install Rust binaries. It enables developers to effortlessly bring in external crates (Rust packages) and binaries into their environment, making it easier to manage and use dependencies locally or globally.
Use case 1: Installing a Package from crates.io
Code:
cargo install package@version
Motivation:
This use case is essential when you wish to integrate a Rust library or tool directly from crates.io, the official Rust package registry. By installing a package using cargo install package@version
, you gain immediate access to a myriad of utilities that can improve productivity or introduce new capabilities to your Rust applications. The ability to specify a version ensures compatibility with your existing setup or codebase, eliminating unexpected breakages due to new updates in the package.
Explanation:
cargo install
: This command tells Cargo to build and install a package.package
: The name of the package you want to install from crates.io. If no version is specified, Cargo will install the latest available version.@version
: (Optional) This allows you to specify a particular version of the package you wish to install. This can be especially useful when you require a specific release that another package depends on or when a newer version may contain breaking changes that you wish to avoid.
Example Output: Upon successful installation, the command will print messages detailing the downloading and building process of the package, followed by a statement confirming installation. It typically looks like this:
Updating crates.io index
Downloading package_name v0.x.y
Compiling package_name v0.x.y
Finished release [optimized] target(s) in 2m 34s
Installing …/bin/package_name
Use case 2: Installing a Package from a Git Repository
Code:
cargo install --git repo_url
Motivation: Sometimes, the functionality you need might not be available in a stable release on crates.io. It might be hosted in a Git repository, possibly containing the latest code or a specific feature branch that hasn’t been released yet. A developer may prefer this method when collaborating directly with a package’s source code or when testing new features before they’re officially published.
Explanation:
cargo install
: The base command to install a Rust package.--git
: This flag tells Cargo to fetch the package from a Git repository instead of crates.io.repo_url
: The URL of the Git repository containing the package’s source code. Cargo uses this to clone the repository and build the package.
Example Output: Output will include information about cloning the repository from the specified URL and then compiling the package from the project within:
Cloning into 'package_name' from 'repo_url'...
Compiling package_name v0.x.y (…/path/to/repo)
Finished release [optimized] target(s) in 3m 12s
Installing …/bin/package_name
Use case 3: Building from a Specific Branch, Tag, or Commit when Installing from a Git Repository
Code:
cargo install --git repo_url --branch|tag|rev branch_name|tag|commit_hash
Motivation: This use case is relevant when the stability or specific changes made on a branch, tag, or commit are required. For instance, bug fixes or experimental features may be incorporated into a branch while the main release remains outdated. Developers and contributors often use this flexibility to test or develop against different states of the package’s history.
Explanation:
cargo install
: Initiates the install process.--git
: Indicates the package source is a Git repository.repo_url
: The URL from which the codebase is cloned.--branch|tag|rev
: Determines the specific point in the repository history to be fetched.branch_name|tag|commit_hash
: Name of the branch, tag, or unique identifier of the commit you wish to use.
Example Output: The output specifies the branch, tag, or commit accessed, illustrating the particular state of the project being built:
Cloning from repo_url, branch 'branch_name'...
Checking out files: 100%
Compiling package_name v0.x.y (.../path/to/cloned_repo)
Finished release [optimized] target(s) in 1m 59s
Installing …/bin/package_name
Use case 4: Listing All Installed Packages and Their Versions
Code:
cargo install --list
Motivation: As a developer accumulates various Rust packages over time, it becomes crucial to keep track of what’s installed. Knowing all installed packages and their versions aids in dependency management, ensuring you have consistent environments, and can help in identifying if specific tools are causing conflicts or require updates.
Explanation:
cargo install
: Initiates the Cargo command.--list
: A special flag that prompts Cargo to show all installed packages and their respective versions.
Example Output: This results in a list of all installed binaries, providing a quick overview:
package_name v0.x.y: …/bin/package_name
other_package v0.a.b: …/bin/other_package
Conclusion:
By using the cargo install
command, Rust developers can readily manage binary installations and dependencies, ensuring they have the right tools and versions at their disposal. Whether pulling the latest packages from crates.io, diving into specific commits from a Git repository, or just ensuring your installed suite is up to date, cargo install
is a robust mechanism that simplifies the developer’s workflow.