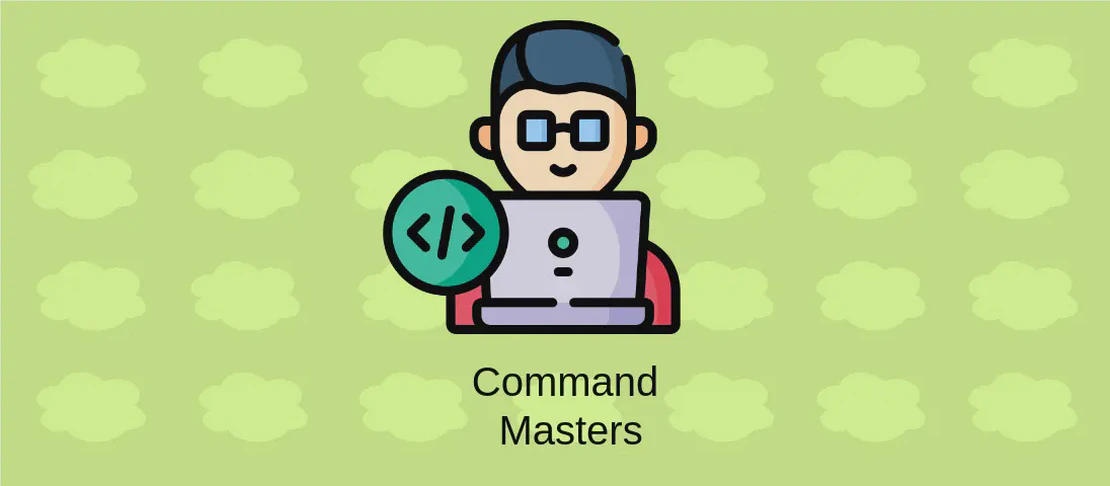
How to Use the Command 'cargo metadata' (with Examples)
Cargo is the Rust package manager and build system. It helps manage Rust projects by handling the downloading of dependencies, compiling source files, and more. The cargo metadata
command is a tool that provides information about the workspace members and resolved dependencies of the current Rust package in JSON format. This command is highly useful for developers who need to programmatically access metadata about their Rust projects, such as dependency graphs, workspace member lists, and platform-specific build information for automation or analysis purposes.
Use Case 1: Print the Workspace Members and Resolved Dependencies of the Current Package
Code:
cargo metadata
Motivation: This use case is particularly useful for developers who need to delve into the intricate details of a Rust project’s dependency structure. When working within a large Rust workspace with multiple packages and complex dependencies, it becomes critical to understand which packages and versions are being pulled in. This information is essential for debugging version conflicts, ensuring compatibility, and optimizing the build process.
Explanation:
cargo
is the Rust package manager command-line tool.metadata
is a subcommand ofcargo
that outputs metadata in JSON format for the current package.
Example Output:
{
"packages": [
{
"name": "example_package",
"version": "0.1.0",
...
}
],
"workspace_members": [
"example_package 0.1.0 (path+file:///.../example_package)"
],
"resolve": {
"nodes": [
{
"id": "example_package 0.1.0 (path+file:///.../example_package)",
"dependencies": []
}
]
},
...
}
Use Case 2: Print Only the Workspace Members Without Fetching Dependencies
Code:
cargo metadata --no-deps
Motivation: This use case is tailored for situations where a developer is only interested in the packages constituting a workspace, without wanting to fetch or resolve any of the dependencies. This can be especially useful during early stages of project setup or when making structural changes to the workspace, as it allows developers to quickly verify the organization of their workspace members.
Explanation:
--no-deps
is an option that prevents the fetching and resolving of dependencies. It restricts the output to only include the workspace members, making the operation faster by avoiding unnecessary network calls or resolution computations.
Example Output:
{
"packages": [
{
"name": "example_package",
"version": "0.1.0",
...
}
],
"workspace_members": [
"example_package 0.1.0 (path+file:///.../example_package)"
],
...
}
Use Case 3: Print Metadata in a Specific Format Based on the Specified Version
Code:
cargo metadata --format-version 1
Motivation:
This use case is ideal for developers who work with tools or scripts dependent on a specific version of the JSON format provided by cargo metadata
. Changes to the JSON format can potentially break compatibility with existing scripts, therefore specifying a format version ensures consistency and stability across different runs and environments.
Explanation:
--format-version version
specifies the version of the JSON output format. As the output format could change between Cargo updates, fixing the format version helps maintain compatibility with scripts or tools that process this metadata.
Example Output:
{
"version": 1,
"packages": [
{
"name": "example_package",
"version": "0.1.0",
...
}
],
...
}
Use Case 4: Print Metadata with the resolve
Field Including Dependencies Only for a Given Target Triple
Code:
cargo metadata --filter-platform x86_64-unknown-linux-gnu
Motivation: When deploying Rust applications across multiple platforms, it’s necessary to understand how dependencies resolve for a specific target architecture or operating system. This use case is important for developers who target specific platforms and need to analyze or cross-compile their projects, making sure that all required platform-specific dependencies are correctly included.
Explanation:
--filter-platform target_triple
restricts the dependencies shown in theresolve
field to those relevant to the specified target triple, wheretarget_triple
represents the target architecture/platform (e.g.,x86_64-unknown-linux-gnu
). While dependencies inpackages
remain comprehensive across all platforms, this option helps focus on the subset pertinent to specific deployment targets.
Example Output:
{
"packages": [
{
"name": "example_package",
"version": "0.1.0",
...
}
],
"resolve": {
"nodes": [
{
"id": "example_package 0.1.0 (path+file:///.../example_package)",
"dependencies": [
"dependency_a"
]
}
]
},
...
}
Conclusion:
The cargo metadata
command is a powerful tool offering detailed insights into Rust projects. Its various flags and options allow for flexible query configurations, enabling developers to extract exactly the information they need. Whether users are looking to audit dependencies, optimize their build processes, or automate project analysis, cargo metadata
provides critical data to help maintain efficient and consistent Rust development workflows.