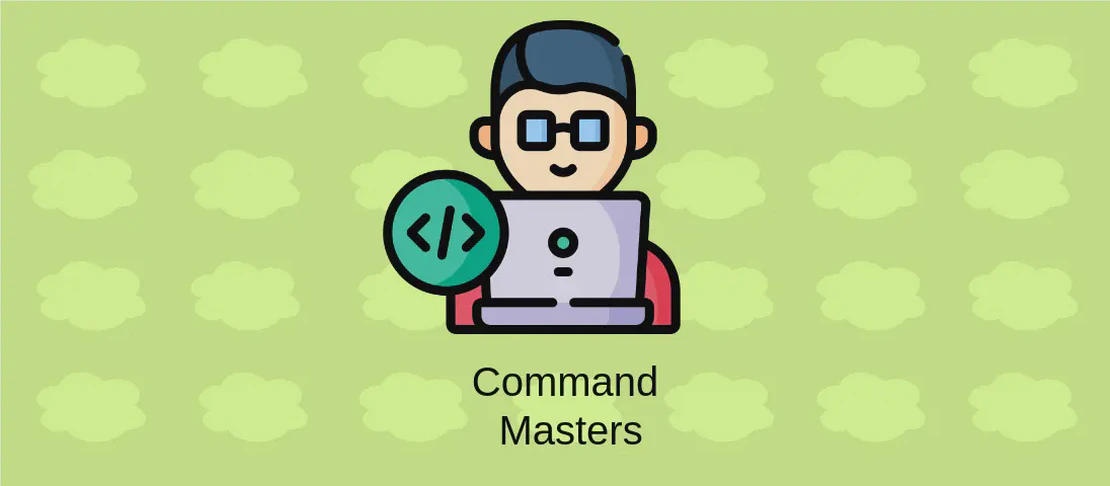
Understanding the 'cargo publish' Command in Rust (with Examples)
The cargo publish
command in Rust serves a critical role in the Rust ecosystem by allowing developers to upload their packages (or crates) to a cargo registry. This process is vital for sharing Rust libraries with others, facilitating collaboration, and making them publicly available for use in various projects. However, before publishing, developers need to authenticate using a token with cargo login
. This article will dive into the various use cases of the cargo publish
command, complete with practical examples to illustrate its applications.
Use case 1: Perform Checks, Create a .crate
File, and Upload it to the Registry
Code:
cargo publish
Motivation:
The primary motivation for this use case is to release a Rust package to a public registry, such as crates.io, where it can be accessed and utilized by the broader developer community. This step is essential when your package is stable and ready for public consumption, allowing others to benefit from your work and potentially contribute back.
Explanation:
cargo
: The package manager for Rust, which is used for building, downloading dependencies, and uploading packages.publish
: The subcommand of cargo that handles the publishing process. It performs a series of checks on your package, creates a.crate
file, and uploads it to the specified registry.
Example Output:
Updating crates.io index
Packaging your_project v0.1.0 (/path/to/your_project)
Verifying your_project v0.1.0 (/path/to/your_project)
Updating crates.io index
Compiling your_project v0.1.0 (/path/to/your_project)
Finished dev [unoptimized + debuginfo] target(s) in 1.01s
Uploading your_project v0.1.0 (/path/to/your_project)
Successfully uploaded to http://crates.io
Use case 2: Perform Checks and Create a .crate
File Without Uploading
Code:
cargo publish --dry-run
Motivation:
This option is vital when you want to verify that your package is ready for publishing without actually uploading it to the registry. It allows developers to perform all the checks that occur during a publish without the risk of releasing an unfinished or buggy version to a public registry.
Explanation:
cargo
: Again, the Rust package manager handling various tasks.publish
: Initiates the package verification and crate creation process.--dry-run
: A flag that ensures all steps leading up to uploading are performed, but the actual upload is skipped. This provides a safe environment for checks.
Example Output:
Packaging your_project v0.1.0 (/path/to/your_project)
Verifying your_project v0.1.0 (/path/to/your_project)
Compiling your_project v0.1.0 (/path/to/your_project)
Finished dev [unoptimized + debuginfo] target(s) in 0.92s
(info): Created `.crate` file at: /path/to/your_project/target/package/your_project-0.1.0.crate
(info): Dry run successful, no upload performed
Use case 3: Use the Specified Registry
Code:
cargo publish --registry my-registry
Motivation:
For situations where you want to publish your package to a registry other than the default crates.io, this functionality is indispensable. Developers may have private registries set up within their organization to host internal Rust crates securely, and this command allows them to specify the registry to which the package should be published.
Explanation:
cargo
: The Rust package manager facilitating crate management.publish
: Initiates the publishing process for your package.--registry my-registry
: Specifies a registry defined in your cargo configuration file where you want the package to be uploaded. This flexibility is crucial for handling multiple registries, especially in enterprise environments utilizing private registries for internal packages.
Example Output:
Updating custom registry index
Packaging your_project v0.1.0 (/path/to/your_project)
Verifying your_project v0.1.0 (/path/to/your_project)
Updating custom registry index
Compiling your_project v0.1.0 (/path/to/your_project)
Finished dev [unoptimized + debuginfo] target(s) in 1.03s
Uploading your_project v0.1.0 (/path/to/your_project)
Successfully uploaded to http://my-custom-registry
Conclusion:
The cargo publish
command is a powerful tool in the Rust developer’s toolkit, enabling the distribution of Rust packages to the community or private registries. By understanding its various use cases, such as performing dry runs, and specifying registries, developers can ensure a smooth and controlled publishing process. Each of these options serves specific purposes that cater to both public and private distribution needs, demonstrating the flexibility and robustness of Cargo as a package manager.