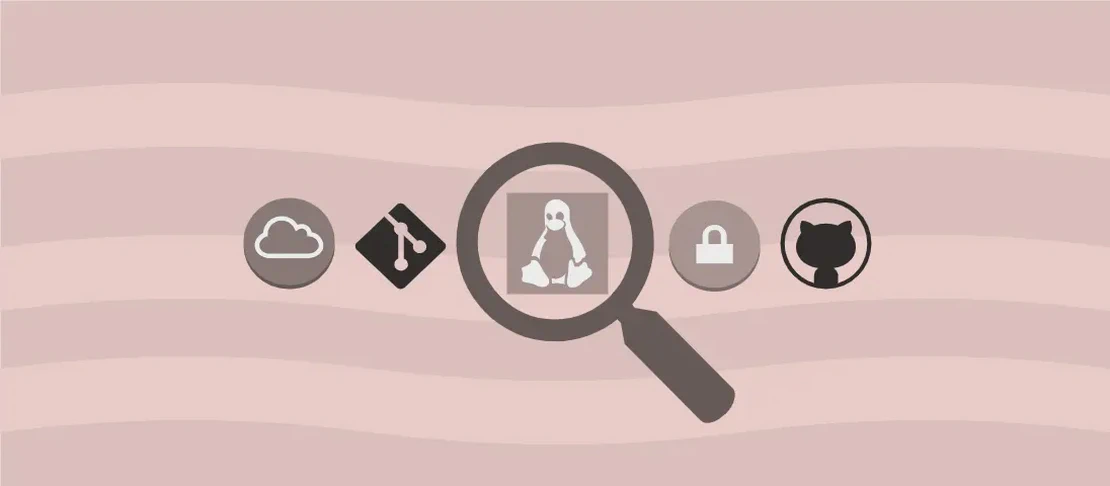
How to use the command 'cargo remove' (with examples)
The ‘cargo remove’ command is a useful tool for removing dependencies from a Rust project’s Cargo.toml manifest. It allows developers to easily remove unnecessary dependencies in order to reduce the size and complexity of their projects. This article will provide examples of how to use the ‘cargo remove’ command in different scenarios.
Use case 1: Remove a dependency from the current project
Code:
cargo remove dependency
Motivation: Sometimes, a project may have a dependency that is no longer needed or is causing conflicts with other dependencies. In such cases, it is best to remove the dependency from the project to avoid any issues.
Explanation: In this use case, the command ‘cargo remove’ is followed by the name of the dependency that needs to be removed from the project. This will prompt Cargo to remove the specified dependency from the project’s Cargo.toml manifest.
Example output:
Removing dependency...
Dependency successfully removed from the project.
Use case 2: Remove a development or build dependency
Code:
cargo remove --dev|build dependency
Motivation: Development and build dependencies are often used during the development and build process but are not required for the final production code. Removing these dependencies can help reduce the size and complexity of the project.
Explanation: To remove a development or build dependency, the ‘–dev’ or ‘–build’ flag is added before the dependency name in the command. This informs Cargo that the specified dependency should be removed from the respective section in the Cargo.toml manifest.
Example output:
Removing development/build dependency...
Development/build dependency successfully removed from the project.
Use case 3: Remove a dependency of the given target platform
Code:
cargo remove --target target dependency
Motivation: In some cases, a project may have dependencies that target specific platforms, such as a different operating system or architecture. If a project no longer needs a dependency for a specific target platform, it can be removed to simplify the project structure.
Explanation: To remove a dependency of a specific target platform, the ‘–target’ flag is followed by the target platform, and then the dependency name in the command. This allows Cargo to remove the specified dependency from the targeted platform section in the Cargo.toml manifest.
Example output:
Removing target dependency...
Target dependency successfully removed from the project.
Conclusion:
The ‘cargo remove’ command provides convenient functionality for removing dependencies from a Rust project’s Cargo.toml manifest. By following the provided examples, developers can easily remove unnecessary dependencies, whether they are general dependencies, development/build dependencies, or dependencies specific to a target platform. Removing unnecessary dependencies helps streamline projects and improve overall project maintainability.