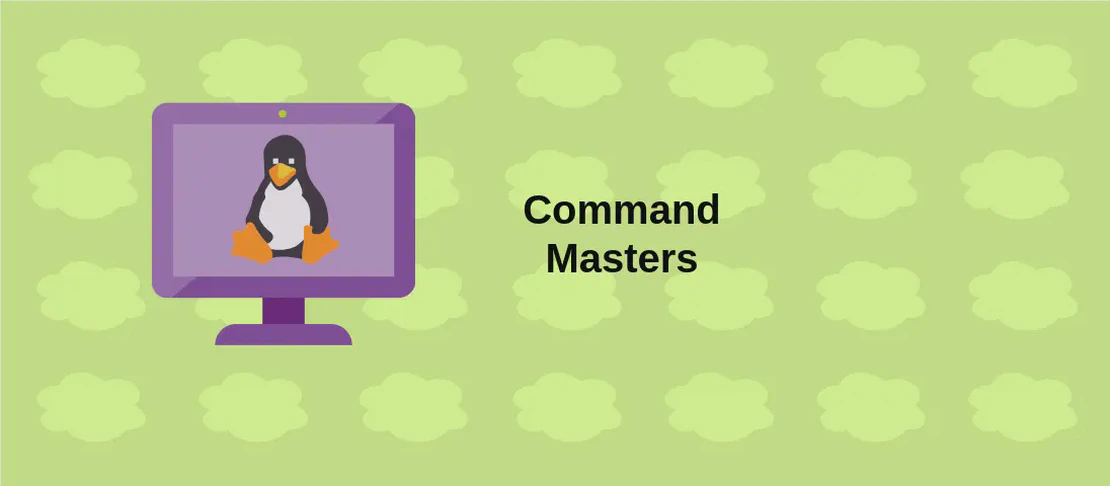
How to run Rust programs with 'cargo run' (with examples)
The cargo run
command is an essential tool for Rust developers, as it efficiently combines the building and execution of a Rust project within one step. Cargo is Rust’s package manager and build system, and cargo run
is particularly useful for compiling your code and running the resultant binary without having to type multiple, separate commands. It automatically detects the necessary dependencies, builds the project, and then executes it, thereby streamlining the development workflow.
Running the default binary target
Code:
cargo run
Motivation:
This is the simplest and most common use case. When you’re working on developing a Rust application and you want to quickly build and test your main project, cargo run
is a straightforward command that compiles the whole project and runs the default binary target in one go.
Explanation:
cargo
is Rust’s package manager, which handles project creation, building, and compilation.run
initiates the process of first compiling the project, then executing the main binary file it produces.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 1.23s
Running `target/debug/my_project`
Hello, Cargo!
Running a specified binary
Code:
cargo run --bin name
Motivation:
When a Rust project contains multiple binary targets, such as projects with different functionalities or tools, you might want to compile and execute just one specific binary. This command comes in handy to select and run the specific binary you’re interested in.
Explanation:
--bin name
specifies which binary target within the package should be built and run. Thename
corresponds to a binary target within theCargo.toml
file.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 0.78s
Running `target/debug/name`
Executing specific binary...
Running a specified example
Code:
cargo run --example name
Motivation:
Examples in Rust projects are small programs provided to demonstrate how parts of the library or application can be used in practice. This use case helps you run only the examples directory programs, which is beneficial for testing or learning.
Explanation:
--example name
indicates that you want to compile and run a project example with the givenname
, referring to programs located in theexamples
directory of your project.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 0.95s
Running `target/debug/examples/name`
Example output for <example>
Activating a space or comma-separated list of features
Code:
cargo run --features feature1 feature2
Motivation:
Features in Cargo are optional functionalities that can be turned on or off for compiling crates. When different builds require different features, this command allows you to specify which additional features should be enabled during the build.
Explanation:
--features feature1 feature2
allows you to activate specific additional features during the compilation. Each feature name corresponds to an optional configuration in the project’s dependencies as defined inCargo.toml
.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 1.12s
Running `target/debug/my_project`
Running with feature set: feature1, feature2
Disabling the default features
Code:
cargo run --no-default-features
Motivation:
If a project comes with default features, and a specific build requires them to be turned off for testing or debugging purposes, this command is useful to compile the project without those default features.
Explanation:
--no-default-features
tells Cargo to refrain from activating all default features specified in theCargo.toml
, which is often used when you want to avoid certain default configurations.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 1.05s
Running `target/debug/my_project`
Running without any default features
Activating all available features
Code:
cargo run --all-features
Motivation:
When you want to ensure that all optional components of a crate work together properly, you can use this command to enable every single available feature. This can be particularly useful for comprehensive testing.
Explanation:
--all-features
activates every possible feature defined in the project’sCargo.toml
, which is an excellent way to ensure compatibility across all features.
Example output:
Compiling my_project v0.1.0
Finished dev [unoptimized + debuginfo] target(s) in 1.45s
Running `target/debug/my_project`
Running with all features activated
Running with the given profile
Code:
cargo run --profile name
Motivation:
When you have multiple profiles configured in your project, such as dev
, release
, or custom profiles, this command allows you to specifically run the project with a desired profile to suit different needs like debugging or performance analysis.
Explanation:
--profile name
specifies which profile to use during the build. Profiles are configurations that alter the build process for specific needs, such as setting optimization levels or debug information.
Example output:
Compiling my_project v0.1.0 (profile: name)
Finished profile [unoptimized + debuginfo] target(s) in 1.30s
Running `target/name/my_project`
Running with profile: <profile name>
Conclusion:
The cargo run
command is a powerful utility within Rust’s ecosystem, simplifying the process of building and maintaining Rust projects by offering flexibility through various options and configurations. Whether you’re working with multiple binaries, examples, features, or profiles, cargo run
can accommodate various development scenarios, streamlining tasks and enhancing productivity.