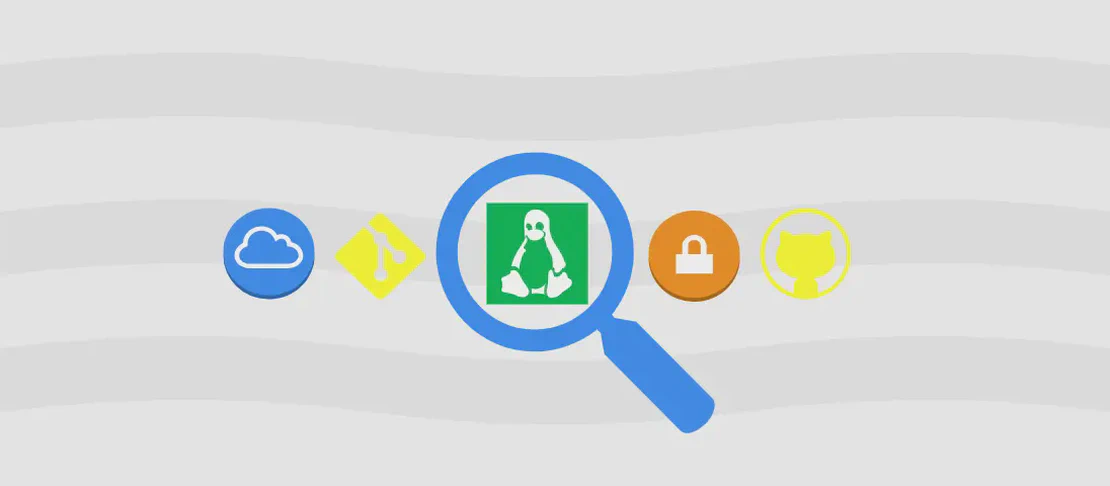
How to Use the Command 'cargo rustc' (with examples)
cargo rustc
is a specialized utility in the Rust development environment, primarily used for compiling Rust packages with additional flexibility. It serves a similar purpose to cargo build
but enhances it by allowing developers to pass extra options directly to the Rust compiler (rustc
). This added flexibility enables more fine-tuned control over the compilation process, making it ideal for optimizing binaries and managing compilation specifics that are critical for project success.
Use case 1: Build the package and pass options to rustc
Code:
cargo rustc -- rustc_options
Motivation:
One fundamental requirement during software development is the ability to customize the build process. By using the cargo rustc -- rustc_options
syntax, developers can pass specific options to the Rust compiler, which is not possible with the basic cargo build
command. This flexibility is essential for developers who need to fine-tune compiler behavior to meet unique requirements of their code or project architecture.
Explanation:
cargo rustc
: This invokes the command to compile a Rust package.--
: This signifies that the following options should be passed directly torustc
, rather than being interpreted by cargo.rustc_options
: These are the specific compiler options that you wish to apply.
Example Output:
Compiling your_crate v0.1.0 (/path/to/your/crate)
Finished dev [unoptimized + debuginfo] target(s) in 0.84s
The output indicates successful compilation, along with the build type and the compilation time.
Use case 2: Build artifacts in release mode, with optimizations
Code:
cargo rustc --release
Motivation:
Developers often need to ensure that their final products run as efficiently as possible. Building in release mode applies optimizations that reduce the size of the final binary and increase its runtime speed. This is ideal for preparing software for production environments where performance is crucial.
Explanation:
cargo rustc
: The base command for compiling the package.--release
: This option tells cargo to employ optimizations, resulting in a more efficient binary tailored for production use.
Example Output:
Compiling your_crate v0.1.0 (/path/to/your/crate)
Finished release [optimized] target(s) in 1.77s
The output highlights the optimized nature of the release build and indicates the binary’s readiness for production deployment.
Use case 3: Compile with architecture-specific optimizations for the current CPU
Code:
cargo rustc --release -- -C target-cpu=native
Motivation:
Certain applications can achieve better performance if optimized for the specific architecture of the CPU they run on. This can leverage CPU-specific features such as advanced SIMD instructions or architectural enhancements, thus maximizing the efficiency of the compiled program on a given machine.
Explanation:
cargo rustc --release
: As before, this compiles the package in release mode with optimizations.--
: This indicates that the next options are for therustc
.-C target-cpu=native
: This flag tells the Rust compiler to generate code optimized for the current host CPU architecture.
Example Output:
Compiling your_crate v0.1.0 (/path/to/your/crate)
Finished release [optimized] target(s) in 1.62s
The example demonstrates a build process that prioritizes the current machine’s CPU features, optimizing the resulting binary for its environment.
Use case 4: Compile with speed optimizations
Code:
cargo rustc -- -C opt-level 1|2|3
Motivation:
During development phases, balancing between build times and execution speed is often necessary. With -C opt-level
coupled with levels 1, 2, or 3, developers can control the extent of optimizations, ranging from basic performance improvements (1) to aggressive optimizations (3) that enhance runtime speed, making it crucial for applications where execution time is a critical metric.
Explanation:
cargo rustc
: Initiates the compilation process.--
: Directs subsequent options torustc
.-C opt-level 1|2|3
: Specifies the optimization level, with higher numbers indicating more aggressive optimizations aimed at improving performance, sometimes at the expense of longer compile times.
Example Output:
Compiling your_crate v0.1.0 (/path/to/your/crate)
Finished dev [unoptimized + debuginfo] target(s) in 0.84s
Here, developers can notice the varying compilation time as they experiment with different optimization levels to find the best fit between build and execution time.
Use case 5: Compile with size optimizations
Code:
cargo rustc -- -C opt-level s|z
Motivation:
In scenarios where the binary size is crucial, such as embedded systems or situations with storage constraints, optimizing for size is paramount. Option -C opt-level s
focuses on reducing the size of the binary, while z
also disallows some vectorizations to achieve even smaller binaries.
Explanation:
cargo rustc
: The command for compiling the Rust package.--
: To pass options directly to therustc
.-C opt-level s|z
: Indicates optimization for size, withs
focusing on size reduction andz
aiming for minimal binary size by disabling some other optimizations.
Example Output:
Compiling your_crate v0.1.0 (/path/to/your/crate)
Finished dev [optimized] target(s) in 1.04s
The output communicates a build process fine-tuned for minimal binary size, which is especially beneficial in constrained environments.
Use case 6: Check if your package uses unsafe code
Code:
cargo rustc --lib -- -D unsafe-code
Motivation:
Safety is a primary pillar of Rust. Developers often prefer to enforce compilations that avoid unsafe code constructs to maintain Rust’s guarantees of memory safety. By using -D unsafe-code
, the compiler will treat any occurrence of unsafe code as a compilation error, promoting a more robust and secure codebase.
Explanation:
cargo rustc --lib
: Specifies that only the library portion should be compiled.--
: Allows the following to be passed torustc
.-D unsafe-code
: The-D
flag treats specified lints as errors; here, it ensures no unsafe code is used.
Example Output:
error: usage of unsafe code is not allowed
--> src/main.rs:XX:XX
|
XX | unsafe { ... }
| ^^^^^^^^^^^^^
error: aborting due to previous error
This output pinpoints where unsafe code is present, supporting efforts to uphold code safety standards.
Use case 7: Build a specific package
Code:
cargo rustc --package package
Motivation:
In projects with multiple packages, developers might want to compile only a specific one to save time and resources. This is useful in large multi-package workspaces where building everything together isn’t necessary and can speed up the development cycle for individual packages.
Explanation:
cargo rustc
: Indicates it’s a compile action.--package package
: Targets a specific package for compilation, wherepackage
should be replaced with the actual package’s name.
Example Output:
Compiling specific_package v0.1.0 (/path/to/specific_package)
Finished dev [unoptimized + debuginfo] target(s) in 0.92s
The output demonstrates efficient use of resources by focusing compilation effort on a specified package.
Use case 8: Build only the specified binary
Code:
cargo --bin name
Motivation:
Sometimes, developers need to focus on a single binary target out of multiple, especially when working on projects with multiple executables. Building only the specified binary can significantly shorten the build process, saving both time and computational resources.
Explanation:
cargo
: The tool used for Rust package management tasks.--bin name
: Specifies that only the binary namedname
should be built.
Example Output:
Compiling main_binary v0.1.0 (/path/to/your/crate)
Finished dev [unoptimized + debuginfo] target(s) in 0.73s
The output indicates that only the target binary has been built, showcasing an efficient process tailoring the build to primary requirements.
Conclusion:
cargo rustc
is a powerful command that extends the functionality of Rust’s build system, granting developers the ability to customize their build processes to suit specific needs. Whether the focus is on optimization, safety, or efficiency, cargo rustc
provides the necessary control to ensure that Rust projects are built to personal or project-specific standards.