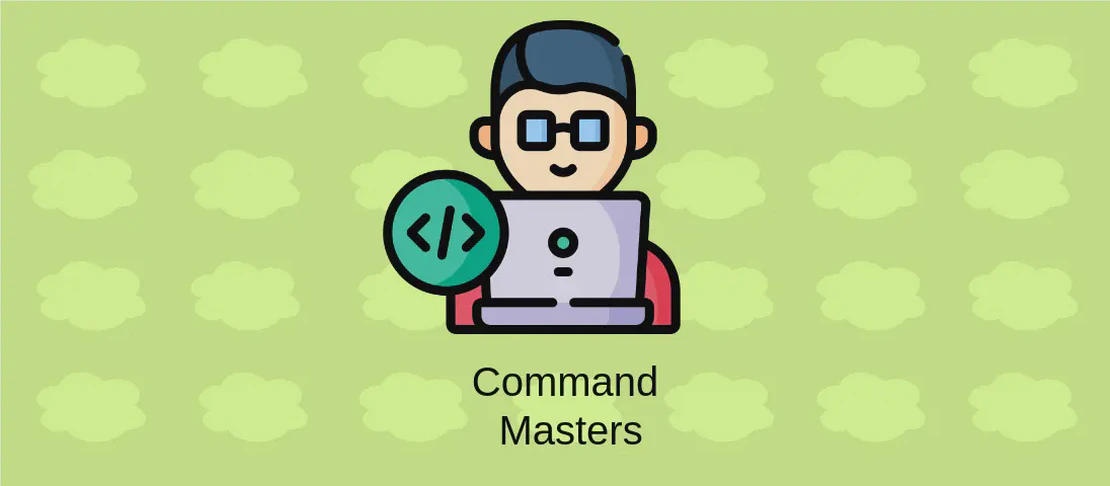
Mastering Cargo Search Command in Rust (with examples)
The cargo search
command is an essential tool for Rust developers looking to find and integrate crates, or packages, into their projects. It serves as a bridge to crates.io, the Rust community’s package registry, allowing developers to discover and compare libraries efficiently. cargo search
displays the results in a TOML format that can be immediately utilized in a project’s Cargo.toml
file, streamlining the process of dependency management. Below, we explore the key use cases of this command with examples.
Use case 1: Searching for Packages
Code:
cargo search regex
Motivation:
Imagine you are developing a Rust application that requires complex pattern matching. You decide to use a regex library to simplify this task. The first step is to find an appropriate crate with the required functionality, which is where cargo search
comes into play. By searching for packages with a keyword that describes the functionality you need, you can quickly shortlist potential crates to integrate into your project.
Explanation:
cargo
: This is the Rust package manager and building system, which provides various commands to manage Rust projects.search
: This subcommand is used to search for packages available on crates.io.regex
: This is the search query, representing the functionality or feature you are looking for. It’s a keyword or phrase that will be matched against package names and descriptions.
Example Output:
regex = "1.5.4" # An implementation of regular expressions for Rust. This library provides a straightforward Regex type and allows pattern matching with a concise API.
regex-automata = "0.1.0" # A low-level abstraction for regular expressions, built on top of a deterministic finite automaton (DFA).
This output shows you various available libraries related to “regex,” each with a version number and brief description, enabling you to choose the most suitable one for your project.
Use case 2: Limiting the Number of Results
Code:
cargo search --limit 5 serde
Motivation: When you conduct a search for a widely-used functionality like serialization, such as with the keyword “serde,” cargo may return many results, making it challenging to sift through them. By limiting the number of results, you can focus on the top recommendations, potentially saving time and effort when evaluating libraries. This can be particularly useful if you need a quick solution or if you’re exploring crates.io during an initial scouting phase for your project.
Explanation:
cargo
: This is the Rust package manager, handling tasks such as creating, building, and managing Rust projects.search
: This allows you to look for crates available on crates.io.--limit 5
: This option restricts the number of search results to just five entries. The default limit is ten, but you can specify any number up to the maximum of 100, allowing you to customize the breadth of your search results.serde
: This is the search query. In this context, you’re interested in crates related to serialization and deserialization.
Example Output:
serde = "1.0.130" # A framework for serializing and deserializing Rust data structures efficiently and generically.
serde_derive = "1.0.130" # Custom derive macros for Serde that implement Serialize and Deserialize traits on types.
serde_json = "1.0.72" # A JSON parser and serializer for Rust, derived from the serde framework.
The truncated result list helps you focus on the most prominent or popular results about “serde,” which are typically well-maintained and widely used by the community.
Conclusion:
The cargo search
command is a powerful ally in the Rust programmer’s toolkit. It enhances the process of finding and selecting crates by allowing developers to conduct keyword searches and filter search results according to their needs. Whether you are seeking a dependency to address specific functionality or aiming to limit search results to make your selection process more manageable, cargo search
is a flexible and efficient resource to support your development efforts.