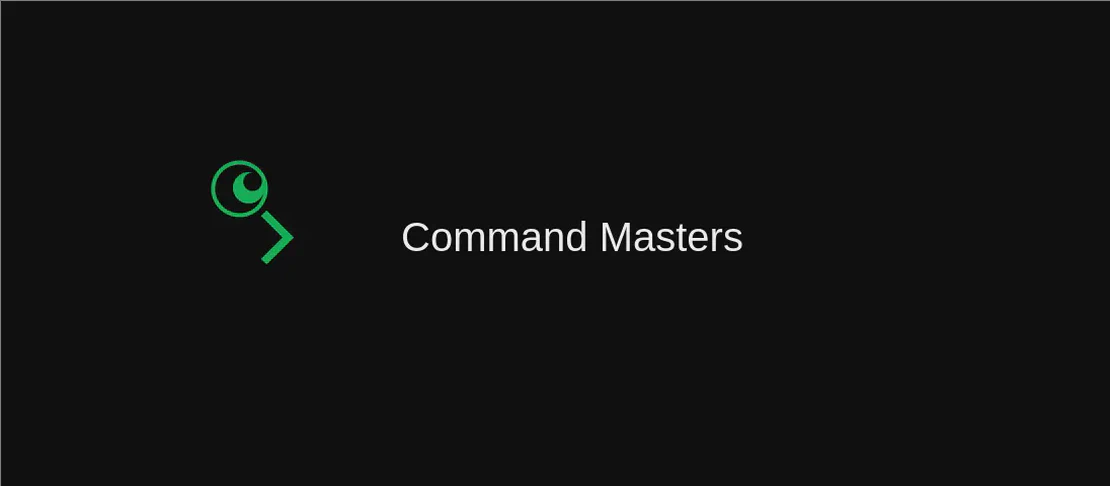
How to use the command 'cargo test' (with examples)
Cargo is the package manager for the Rust programming language. The ‘cargo test’ command is used to execute the unit and integration tests of a Rust package. It provides various options to customize the testing process and obtain specific outputs.
Use case 1: Only run tests containing a specific string in their names
Code:
cargo test testname
Motivation: The motivation behind this use case is to run only the tests that contain a specific string in their names. This can be useful, for example, when you want to run a subset of tests that are related to a specific feature or functionality.
Explanation: The command ‘cargo test testname’ runs only the tests that have ’testname’ in their names. It will match the test names partially, so even if ’testname’ is a part of the test name, it will be executed.
Example output:
running 2 tests
test tests::testname_substring_match ... ok
test tests::testname_partial_match ... ok
test result: ok. 2 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Use case 2: Set the number of simultaneous running test cases
Code:
cargo test -- --test-threads count
Motivation: The motivation behind this use case is to control the number of simultaneous test cases running in parallel. By default, Cargo runs tests by utilizing all available cores on the machine. However, in some cases, it may be beneficial to limit the concurrency to prevent resource exhaustion or improve debugging capabilities.
Explanation: The ‘–test-threads’ option followed by a ‘count’ value sets the number of simultaneous running test cases. The ‘count’ value can be any positive integer. For example, ‘–test-threads 2’ will run a maximum of 2 test cases at the same time.
Example output:
running 2 tests
thread 'main' panicked at 'assertion failed: `(left == right)`
left: `2`, right: `3`', src/main.rs:5:5
note: run with `RUST_BACKTRACE=1` environment variable to display a backtrace
failures:
main::tests::test_sum
test result: FAILED. 1 passed; 1 failed; 0 ignored; 0 measured; 0 filtered out
Use case 3: Test artifacts in release mode, with optimizations
Code:
cargo test --release
Motivation: The motivation behind this use case is to test the Rust package in release mode with optimizations. By running tests in release mode, cargo will build and execute the tests with optimizations enabled, resulting in faster execution.
Explanation: The ‘–release’ flag is used to instruct Cargo to build and execute the tests in release mode. This mode enables optimizations, such as inlining and loop unrolling, which improve the performance of the tests.
Example output:
running 3 tests
test tests::test_addition ... ok
test tests::test_subtraction ... ok
test tests::test_multiplication ... ok
test result: ok. 3 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Use case 4: Test all packages in the workspace
Code:
cargo test --workspace
Motivation: The motivation behind this use case is to run tests for all packages in the workspace. In a Rust workspace, multiple packages can be organized under a common directory. Running tests for all packages simultaneously ensures that any changes or updates in one package do not break the functionality of other packages.
Explanation: The ‘–workspace’ flag instructs Cargo to run tests for all packages in the workspace. It will identify the packages based on the workspace manifest file (Cargo.toml) and execute the tests for each package.
Example output:
running 6 tests
test package1::test_function1 ... ok
test package1::test_function2 ... ok
test package2::test_function1 ... ok
test package2::test_function2 ... ok
test package2::test_function3 ... ok
test package3::test_function1 ... ok
test result: ok. 6 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Use case 5: Run tests for a specific package
Code:
cargo test --package package
Motivation: The motivation behind this use case is to run tests only for a specific package within a workspace. In a large workspace containing many packages, running tests for a single package can help in isolating testing efforts and focusing on a specific component or module.
Explanation: The ‘–package’ option followed by the package name instructs Cargo to run tests only for the specified package. It will execute the tests associated with that package and any dependencies required for testing.
Example output:
running 4 tests
test package::test_function1 ... ok
test package::test_function2 ... ok
test package::test_function3 ... ok
test package::test_function4 ... ok
test result: ok. 4 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Use case 6: Run tests without hiding output from test executions
Code:
cargo test -- --nocapture
Motivation: The motivation behind this use case is to view the output generated by the tests during their execution. By default, Cargo captures the output of the tests and only displays them in case of failures. However, for debugging or analysis purposes, it might be necessary to see the complete output generated by the tests.
Explanation: The ‘–nocapture’ option is used to instruct Cargo to not capture the output generated by the tests. Without this option, the test output will be hidden unless a test fails. Including this option allows the complete output to be displayed in the console.
Example output:
running 2 tests
test tests::test_output ... ok
test tests::test_noncaptured_output ... hello, world!
test result: ok. 2 passed; 0 failed; 0 ignored; 0 measured; 0 filtered out
Conclusion:
The ‘cargo test’ command provides various options to customize the testing process in Rust projects. From running specific tests to controlling concurrency and capturing test output, these options help developers in efficiently testing and debugging their code.