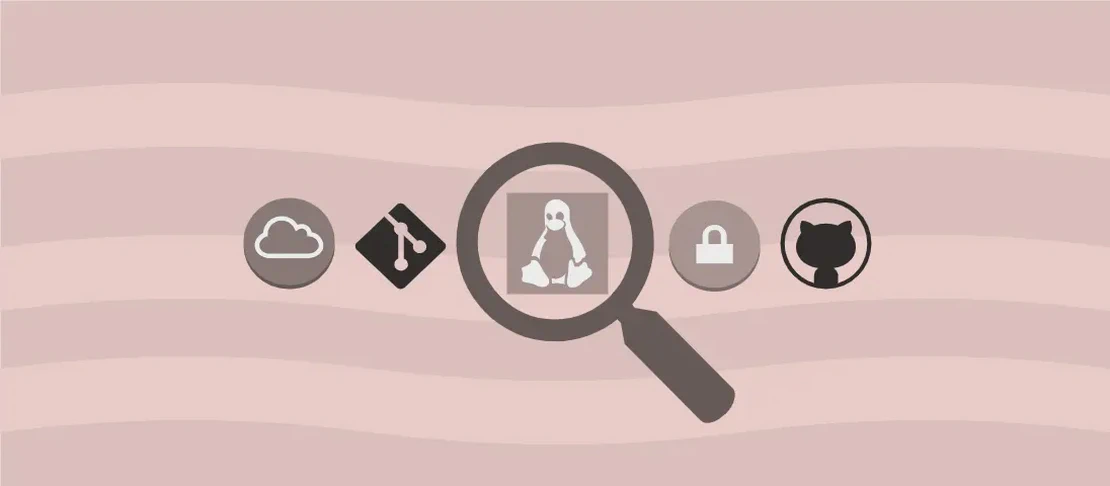
Visualizing Rust Project Dependencies with 'cargo tree' (with examples)
Cargo is the Rust package manager that allows developers to automate numerous tasks in the Rust development environment, one of which is managing dependencies. The cargo tree
command is a particularly powerful tool for visualizing the dependency graph of a Rust project. This command provides a tree-like representation of the project’s dependencies, making it easier for developers to manage and understand the structure of their code. Developers can customize the output using various flags to gain specific insights into their project’s dependencies. The following sections will explore different use cases of the cargo tree
command to illustrate its versatility and utility.
Use Case 1: Display a Dependency Tree of the Current Project
Code:
cargo tree
Motivation:
When working on a Rust project, it’s essential to understand all the libraries and components that your project depends on. Viewing a complete dependency tree gives you a clear picture of how your project is structured and can help diagnose issues related to dependency conflicts or updates. It also ensures you’re aware of all indirect dependencies that might affect your project.
Explanation:
The command cargo tree
generates a tree-like representation of the dependency graph for the current Rust project. This visual format allows you to see not only your direct dependencies but also their dependencies, and so on, giving a comprehensive view of everything that is connected to your project.
Example Output:
my_project v0.1.0 (/path/to/my_project)
├── rand v0.8.3
│ ├── getrandom v0.2.3
│ │ └── cfg-if v1.0.0
│ └── libc v0.2.92
└── serde v1.0.126
├── serde_derive v1.0.126 (proc-macro)
└── serde_derive_internals v0.26.0
Use Case 2: Only Show Dependencies Up to the Specified Depth
Code:
cargo tree --depth 1
Motivation:
In many situations, developers might be interested only in the direct dependencies of their project rather than the entire hierarchy. For instance, when updating a dependency or when focused on understanding or discussing higher-level architecture without diving into too many details, it becomes critical to filter out the noise created by nested dependencies.
Explanation:
The --depth
argument followed by a number specifies the maximum depth to which the tree should be expanded. In this case, the depth is set to 1, which means that only direct dependencies of the current project are shown.
Example Output:
my_project v0.1.0 (/path/to/my_project)
├── rand v0.8.3
└── serde v1.0.126
Use Case 3: Do Not Display the Given Package (and Its Dependencies) in the Tree
Code:
cargo tree --prune rand
Motivation:
When working with complex dependency trees, there might be cases where a particular package and its dependencies are not relevant to the current analysis. This could be the case when troubleshooting or when conducting a focused exploration of a specific segment of the dependency chain. Pruning unnecessary branches helps in simplifying the graph for easier interpretation.
Explanation:
The --prune
argument specifies the package that should be omitted from the tree. This can be useful when the inclusion of certain packages clutters the tree and obscures more relevant dependencies.
Example Output:
my_project v0.1.0 (/path/to/my_project)
└── serde v1.0.126
Use Case 4: Show All Occurrences of Repeated Dependencies
Code:
cargo tree --no-dedupe
Motivation:
Sometimes, checking for cases where a dependency appears multiple times in various branches of the project tree is necessary. Such repetitions might arise from different versions being required by separate dependencies, thus leading to “dependency hell.” This can help in pinpointing why multiple versions of a dependency are being used, and in deciding how best to resolve them.
Explanation:
The --no-dedupe
argument instructs Cargo to include all instances of a dependency in the output, without ignoring duplicates. This is particularly useful for identifying multiple instances of the same dependency that may differ in version or source.
Example Output:
my_project v0.1.0 (/path/to/my_project)
├── rand v0.8.3
│ └── getrandom v0.2.3
└── serde v1.0.126
└── getrandom v0.1.16
Use Case 5: Only Show Normal/Build/Development Dependencies
Code:
cargo tree --edges normal
Motivation:
Rust projects can have different types of dependencies: normal, build, and development. It is often useful to focus exclusively on one category to better manage dependencies and understand their scope. For instance, understanding normal runtime dependencies versus build time, or development testing dependencies helps in optimizing builds and execution environments.
Explanation:
The --edges
argument filters the displayed dependencies based on their type. By specifying normal
, the command limits the output to dependencies that are required for the normal operation of the project, excluding those needed only during build or testing.
Example Output:
my_project v0.1.0 (/path/to/my_project)
└── serde v1.0.126
Conclusion:
The cargo tree
command is an essential tool in any Rust developer’s toolbox, providing essential insights into how various components of a project are interrelated. By leveraging different options and flags, developers can tailor the output to their needs, whether to comprehend the full scope of their dependencies, troubleshoot issues, maintain optimal project structure, or prepare for updates across complex dependency chains. Understanding these use cases provides a solid foundation for effective dependency management in Rust projects.