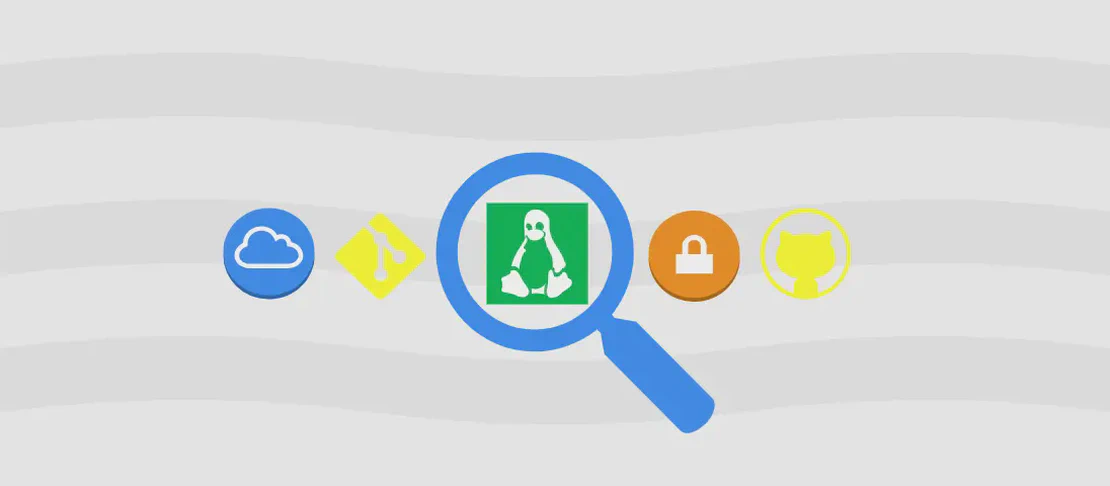
How to use the command 'cargo update' (with examples)
The cargo update
command is a vital aspect of Rust’s package manager, Cargo. It helps developers manage and update dependencies within their Rust projects. By modifying the Cargo.lock
file, this command enables developers to ensure their projects use the most recent, stable version of dependencies, which is essential for maintaining compatibility and taking advantage of bug fixes and performance improvements in third-party libraries. This article explores various ways to leverage cargo update
effectively through a series of detailed use cases.
Use case 1: Update dependencies to the latest version
Code:
cargo update
Motivation:
When working on a Rust project, developers need to ensure that their dependencies are up-to-date. Dependencies frequently receive updates that might include bug fixes, security patches, or enhancements. By running cargo update
, you can pull in these updates to the dependencies specified in your Cargo.lock
file, ensuring that you’re building your project with the latest compatible library versions.
Explanation:
cargo
: This is the Rust package manager.update
: This subcommand modifies theCargo.lock
file to utilize the newest available versions specified by your existingCargo.toml
.
Example Output: Upon running the command, you might see output like the following:
Updating crates.io index
Updating dependency1 v0.1.0 -> v0.2.0
Updating dependency2 v1.0.2 -> v1.0.4
This output indicates that the specified dependencies have been updated to newer versions available in the crates registry.
Use case 2: Display what would be updated without modification
Code:
cargo update --dry-run
Motivation:
Before committing to changes in your dependencies, it’s often helpful to preview what will be updated. Using the --dry-run
option allows developers to see this information without actually modifying the Cargo.lock
file. This is particularly useful for assessing potential impacts of updates or discussing changes with team members.
Explanation:
--dry-run
: This flag tells Cargo to simulate the update process and display the changes without actually performing them.
Example Output: The command might return:
Would update dependency1 v0.1.0 -> v0.2.0
Would update dependency2 v1.0.2 -> v1.0.4
This listing indicates the versions of the dependencies that would be changed if the cargo update
command were run without --dry-run
.
Use case 3: Update only specific dependencies
Code:
cargo update --package dependency1 --package dependency2 --package dependency3
Motivation: In large projects with numerous dependencies, you might want to update only a subset of them rather than all dependencies at once. This approach reduces the risk of inadvertently introducing breaking changes or other issues by limiting the updates to known, safe targets.
Explanation:
--package
: The flag specifies the particular package(s) you want to update. Each following argument is the name of a dependency as described in yourCargo.toml
.
Example Output: Executing this command may result in output like:
Updating dependency1 v0.1.0 -> v0.2.0
Updating dependency2 v1.0.2 -> v1.1.0
Updating dependency3 v2.0.0 -> v2.0.1
This indicates that only the specified packages are updated in their respective versions.
Use case 4: Set a specific dependency to a specific version
Code:
cargo update --package dependency --precise 1.2.3
Motivation:
Sometimes a project might require locking a dependency to a specific version due to compatibility or testing requirements. The --precise
flag allows developers to update a package to a precisely defined version, giving control over the library versions used in the project.
Explanation:
--package dependency
: This specifies the target dependency package for the update.--precise 1.2.3
: This flag, combined with a version number, locks the package to exactly version 1.2.3.
Example Output: The command yields output such as:
Updating dependency v1.2.0 -> v1.2.3
This indicates that the version of dependency
in use has been explicitly updated to 1.2.3.
Conclusion:
The cargo update
command is a flexible tool that aids Rust developers in managing dependencies efficiently. Whether performing a global update, previewing changes, targeting specific packages, or pinning to a precise version, understanding and utilizing these options ensures your projects are using the optimal versions of all dependencies. Keeping dependencies current or stable as needed enhances the stability, security, and performance of Rust applications.