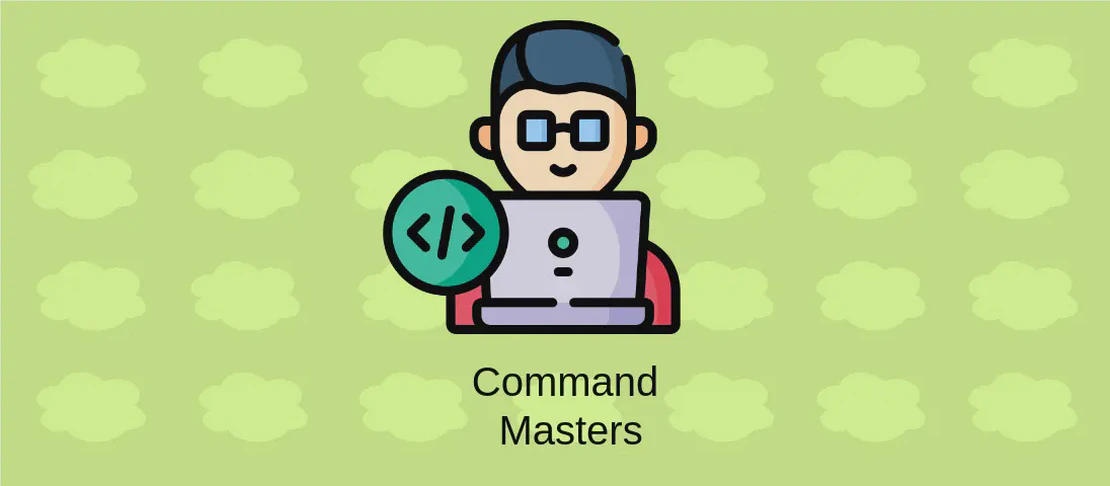
How to use the command `case` in Bash (with examples)
The case
command in Bash is a powerful built-in construct used for creating multi-choice conditional statements. It functions similarly to a switch-case statement found in other programming languages and is used for matching a variable against one or more patterns and executing corresponding commands. This allows programmers to branch logic in scripts in a clean, concise, and readable manner. It is particularly useful when one needs to execute different pieces of code depending on the value of a specific variable.
Use case 1: Match a variable against string literals to decide which command to run
Code:
case $tocount in
words) wc -w README; ;;
lines) wc -l README; ;;
esac
Motivation for using this example:
This example illustrates a basic use case of the case
command in which the value of a variable ($tocount
) determines which specific command to execute. This type of conditional branching is essential in scripting for situations where a variable might take on several discrete values and each value results in different processing. In this scenario, we want to count either the number of words or lines in a file based on the user’s input. The case
command simplifies the logic and improves readability compared to using multiple if-elif
statements.
Explanation for every argument:
tocount
: This is the variable we are matching against, representing what we intend to count (either words or lines).words)
: This pattern matches when the variabletocount
is exactly ‘words’. The command following this pattern (wc -w README
) will be executed if there is a match.wc -w README;
: Uses thewc
(word count) command with the-w
option to count the number of words in the file named README.lines)
: This pattern matches whentocount
is ’lines’. The subsequent commandwc -l README
will run if matched.wc -l README;
: Uses thewc
command with the-l
option to count the number of lines in the README file.;;
: Marks the end of the command list for the preceding pattern.esac
: Ends thecase
command block, similar to howfi
ends anif
block.
Example output:
Assume $tocount
is set to “words”, and the README file has 350 words. The output would be:
350 README
Use case 2: Combine patterns with |, use * as a fallback pattern
Code:
case $tocount in
[wW]|words) wc -w README; ;;
[lL]|lines) wc -l README; ;;
*) echo "what?"; ;;
esac
Motivation for using this example:
This example demonstrates how case
can be expanded to allow for greater flexibility in pattern matching by combining multiple patterns and providing a fallback option. This can greatly enhance user experience and error handling in scripts. Suppose you want to accept more flexible input, such as different capitalizations or synonyms. Additionally, providing a fallback ensures that the script gracefully handles unexpected input, alerting the user without causing a crash or undefined behavior.
Explanation for every argument:
[wW]|words
: Matches iftocount
is either ‘w’, ‘W’, or ‘words’. This pattern allows for flexible input, accommodating both case variations of a single letter (‘w’) as well as the full word (‘words’).|
: The pipe (|
) serves as an OR logical operator between different patterns. If any of the patterns to either side match, the associated command is executed.wc -w README;
: Iftocount
matches any of these patterns, this command will count words in theREADME
file.[lL]|lines
: Similarly, this matches ’l’, ‘L’, or ’lines’.wc -l README;
: When this pattern matches, the command counts lines in theREADME
file.*
: The asterisk symbol serves as a wildcard pattern that matches any string when no other pattern matches. It acts as a default or fallback case when inputs do not meet any listed conditions.echo "what?"
: In case of an unexpected value oftocount
, this command outputs the string “what?”, providing a simple form of error messaging.;;
: Again, marks the end of a command list for the corresponding pattern.esac
: Closes thecase
statement block.
Example output:
Assume $tocount
is set to “WOrds” and the README file has 450 words. The output would be:
450 README
And if $tocount
were set to “characters”, which doesn’t match any pattern, the output would be:
what?
Conclusion:
The case
command in Bash scripting provides a versatile and human-readable way to handle multiple conditional branches. Through string literal matching and pattern combinations with fallback options, it allows scripts to manage diverse user input scenarios and maintain good error handling practices. Whether you’re executing simple scripts or developing more complex functions, understanding and utilizing the case
construct can significantly enhance code structure and functionality.