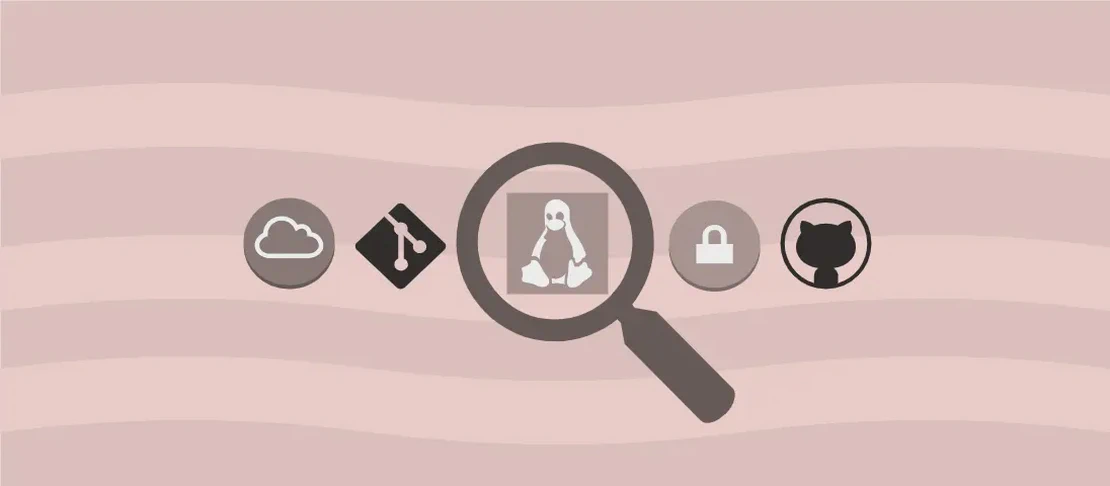
Mastering the 'cat' Command in Unix-Based Systems (with examples)
- Linux
- December 17, 2024
The ‘cat’ (short for “concatenate”) command is a powerful tool in Unix-based operating systems that is widely used for reading, concatenating, and creating files. It reads files sequentially, writing them to standard output and is commonly used to display file contents and merge multiple files. Despite its simplicity, ‘cat’ offers several options that enhance its utility in various scenarios, making it indispensable for both beginners and experienced users alike.
Use case 1: Print the contents of a file to stdout
Code:
cat path/to/file
Motivation: This is perhaps the simplest and most common use of the ‘cat’ command: displaying the contents of a file directly to the terminal. For quick checks on file contents, such as log files, configuration files, or plain text files, this functionality saves time and is easy to execute.
Explanation:
cat
: Invokes the ‘cat’ command.path/to/file
: Specifies the path to the file whose contents you want to display. Replace this placeholder with the actual file path.
Example output: When you execute the command, the entire content of the specified file will be streamed to your terminal. If the file, for example, contains a list of items, it would appear exactly as it is stored within the file.
Use case 2: Concatenate several files into an output file
Code:
cat path/to/file1 path/to/file2 ... > path/to/output_file
Motivation: This use case is valuable when you need to merge several files into a new single file. This situation commonly arises in text processing, where data from multiple files need to be collated into one for analysis or documentation purposes.
Explanation:
cat
: Initiates the ‘cat’ command.path/to/file1 path/to/file2 ...
: Lists the files to be concatenated. Each file path should be separated by a space.>
: This redirection operator signifies that the output should be written to a file.path/to/output_file
: Specifies the destination file for the concatenated content. If this file does not exist, it will be created.
Example output: The output will not be shown in the terminal, as it is redirected into a new file. The new file will contain the content of ‘file1’, followed by ‘file2’, and so on, emulating the order specified in the command.
Use case 3: Append several files to an output file
Code:
cat path/to/file1 path/to/file2 ... >> path/to/output_file
Motivation: Appending files is useful when you have a destination file that already contains data but needs to be updated with more content from other files. This is common in scenarios where logs or collected data sets are progressively accumulated over time.
Explanation:
cat
: Calls the ‘cat’ command.path/to/file1 path/to/file2 ...
: Lists the files to be appended.>>
: This redirection operator means append the contents (instead of overwriting) to the existing file.path/to/output_file
: Denotes the file to which the content will be appended. This file must exist prior to execution.
Example output: While nothing is displayed in the terminal, the specified output file’s content will be augmented with the content from the files listed in the command, preserving existing data.
Use case 4: Write stdin
to a file
Code:
cat - > path/to/file
Motivation: This use case provides a succinct method for taking input directly from the terminal (via standard input) and writing it verbatim to a file. It is incredibly useful for quickly creating or editing text files without invoking a text editor.
Explanation:
cat
: Launches the command.-
: Implies reading from standard input (stdin).>
: Directs the resultant output to a filesystem object.path/to/file
: Identifies the file to write the stdin content. A new file is created if none exists, or if the file exists, it is overwritten.
Example output: Running this command will allow you to enter text (line by line). Once finalized (usually by pressing ‘Ctrl+D’ in Unix-like environments), the text is written to the specified file.
Use case 5: Number all output lines
Code:
cat -n path/to/file
Motivation: When dealing with large text files, keeping track of specific lines can become cumbersome. Numbering each line can make references and editing tasks simpler, especially in coding, documentation, or error tracking contexts.
Explanation:
cat
: Triggers the command.-n
: This flag instructs ‘cat’ to preface each line with its line number.path/to/file
: Points to the file whose contents will be displayed with numbered lines.
Example output: As a result, the terminal will display the contents of the file prefixed with line numbers, enhancing the readability and management of the text data.
Use case 6: Display non-printable and whitespace characters
Code:
cat -v -t -e path/to/file
Motivation: This advanced use case is vital in debugging scenarios where invisible or non-standard characters might be influencing data processing operations. It is frequently employed for identifying problematic characters in dataset or configuration files.
Explanation:
cat
: Invokes the cat utility.-v
: Makes non-printing characters visible, except for tabs and the end-of-line character.-t
: Displays tabs as ‘^I’.-e
: Shows the end-of-line as ‘$’.path/to/file
: Indicates the file whose character content is being evaluated.
Example output: On execution, all non-printable characters will become visible alongside the tabs and newline markers, providing a transparent view into the file’s character structure.
Conclusion:
The ‘cat’ command is far more versatile than its initial simplicity suggests. From displaying text files and combining various datasets to formatting line numbers or revealing hidden character details, ‘cat’ serves a range of purposes crucial for everyday file handling and system administration. Through these examples, we witness its multifaceted applications under different real-world scenarios, highlighting why it remains a cornerstone in the Unix and Linux command repertoire.