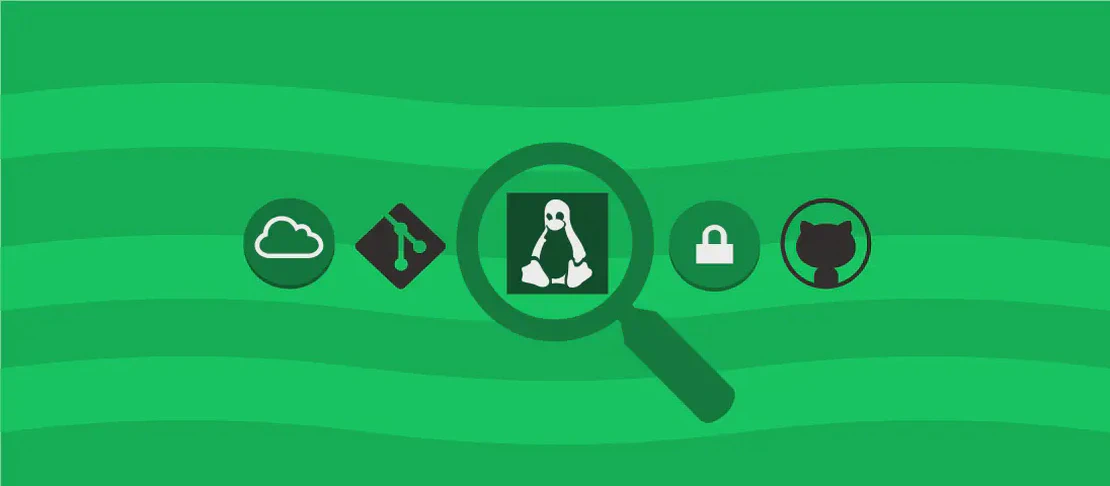
How to Use the Command 'cbt' (with examples)
Google Cloud’s cbt is a command-line utility designed to interact efficiently with Bigtable, a petabyte-scale, fully managed NoSQL database service that is ideal for analytical and operational workloads. This tool allows users to perform a variety of tasks such as reading rows, listing tables, and fetching specific data from Bigtable. Here’s an exploration of some common use cases:
Use Case 1: Listing Tables in the Current Project
Code:
cbt ls
Motivation:
Understanding which tables are available in your Bigtable instance is fundamental when starting a data exploration or manipulation task. This command is particularly useful in scenarios where multiple users manage numerous tables across a project. It gives users a quick overview of the available resources without needing to access the Google Cloud Console.
Explanation:
cbt
: The command-line tool for interacting with Bigtable.ls
: Short for ’list’, this command lists all the tables in the current project. It simplifies the process by providing a direct command to retrieve this information instead of navigating through a graphical interface.
Example Output:
my-table-1
my-table-2
user-data
sales-records
Use Case 2: Print Count of Rows in a Specific Table
Code:
cbt count "table_name"
Motivation:
Knowing the row count of a table is essential for tasks like gauging the volume of data, planning load testing, or performing data validation. This command helps users estimate resource usage or assess changes over time when dealing with dynamic datasets.
Explanation:
cbt
: The main command line tool.count
: This argument counts the number of rows in the specified table."table_name"
: The name of the table for which you want to count the rows. This argument specifies the target table.
Example Output:
Row count for table 'user-data': 123456
Use Case 3: Display a Single Row with Only One Cell Revision Per Column
Code:
cbt lookup "table_name" "row_key" cells-per-column=1
Motivation:
Often, you may need to retrieve the most recent update for each column in a specific row. This is useful in scenarios where column data is frequently updated, but only the latest value is relevant—for example, when examining current status or recent transactions.
Explanation:
cbt
: The tool facilitating interaction with Bigtable.lookup
: This argument fetches data for a specific row in a table."table_name"
: The target table name."row_key"
: The unique identifier for the row of interest.cells-per-column=1
: Limits the output to the most recent cell revision per column, ensuring that only the latest data point is displayed.
Example Output:
family1:qualifier1 @ 2023/10/03-15:16:27.123000 value1
family2:qualifier2 @ 2023/10/03-15:16:27.123000 value2
Use Case 4: Display a Row with Specific Columns
Code:
cbt lookup "table_name" "row_key" columns="family1:qualifier1,family2:qualifier2,..."
Motivation:
This use case is beneficial when you need data from particular columns within a row without retrieving the entire dataset. It is efficient for focused data analysis and unnecessary data transfer reduction, especially in large tables with numerous columns.
Explanation:
cbt
: The command utility for accessing Bigtable.lookup
: Requests specific row data."table_name"
: Specifies which table to query."row_key"
: Indicates the unique identifier of the row to be retrieved.columns="family1:qualifier1,family2:qualifier2,...
": Filters the result to include only specified columns, reducing data to relevant fields.
Example Output:
family1:qualifier1 @ 2023/10/03-15:16:27.123000 value1
family2:qualifier2 @ 2023/10/03-15:16:27.123000 value2
Use Case 5: Search Rows by a Regex Pattern
Code:
cbt read "table_name" regex="row_key_pattern" count=5
Motivation:
Using a regex pattern to search rows is crucial when matching or filtering row keys by specific patterns like prefixes, structures, or properties. This is particularly useful for finding subsets of data efficiently without iterating over the full table.
Explanation:
cbt
: The interface to Bigtable.read
: Command for reading rows from a table."table_name"
: The target table.regex="row_key_pattern"
: Applies the regex pattern to search for row keys that match.count=5
: Limits the search result to the first five matching rows, preventing information overload and improving response times.
Example Output:
row1
row3
row5
row7
row9
Use Case 6: Read Rows in a Specific Range
Code:
cbt read table_name start=start_row_key end=end_row_key keys-only=true
Motivation:
Reading rows within a specific key range is often necessary when working with ordered datasets or time-based records. By retrieving just the keys, users can quickly perform an initial exploratory analysis to decide on further processing actions.
Explanation:
cbt
: The Bigtable command interface.read
: Extracts data from a table.table_name
: Indicates the table to access.start=start_row_key
: Specifies the starting point for the row key range.end=end_row_key
: Sets the endpoint for the row key range.keys-only=true
: Ensures that only the keys, not the entire row data, are returned, speeding up the operation and reducing bandwidth usage.
Example Output:
start_row_key
middle_row_key
another_row_key
Conclusion:
The cbt
utility is a critical tool for efficiently managing and retrieving data from Google Cloud’s Bigtable. These commands facilitate a range of operations from basic table management to detailed data extraction, making the jobs of system administrators and data analysts more straightforward and efficient.