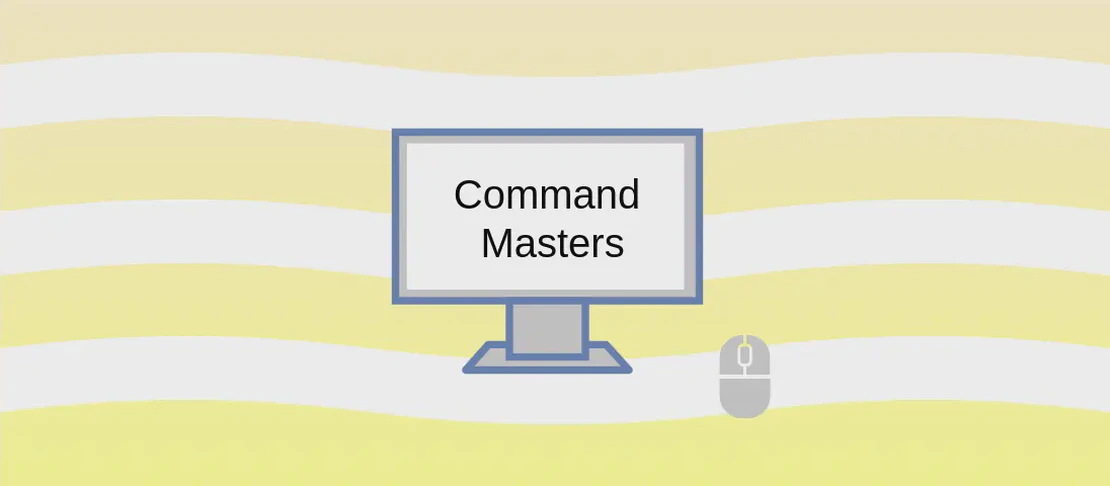
Harnessing the Power of 'cdecl' for C and C++ Declarations (with examples)
The cdecl
tool is an invaluable resource for programmers who work with C and C++ code. It is specifically designed to simplify the often convoluted syntax and semantics of C and C++ type declarations. By allowing users to both create and interpret these declarations in a human-readable form, cdecl
makes programmers’ lives easier, improving their productivity and efficiency. Whether you’re trying to figure out what a complex pointer-to-function declaration means or need to ensure your own declarations are syntactically correct, cdecl
offers you the perfect solution.
Use case 1: Compose English phrase into C declaration, and create compilable output
Code:
cdecl -c phrase
Motivation:
This use case is designed to help programmers who need to define a complex variable or function in C but struggle to articulate the nuanced syntax. By translating English phrases directly into C code, cdecl
saves time and reduces errors, ensuring that the resulting code is syntactically correct and ready for compilation.
Explanation:
-c
: This flag stands for “compose” and instructscdecl
to generate a C declaration from a given English phrase. The option ensures that the output is complete with semicolons and braces, making it directly usable in programming environments.phrase
: The phrase you provide serves as input, representing the intended functionality or declaration that you require in C syntax.
Example output:
Suppose you input the phrase “pointer to array of integers.” The output could be:
int (*ptr)[];
In this example, cdecl
interprets the phrase and outputs a standard C declaration of a pointer to an array of integers, complete with the necessary syntax and punctuation.
Use case 2: Explain C declaration in English
Code:
cdecl explain C_declaration
Motivation:
C and C++ declarations can often be difficult to decipher, especially for complex data types involving pointers, arrays, or functions. This use case serves programmers who encounter such intricate declarations and need a straightforward English explanation to understand or debug the code.
Explanation:
explain
: This command tellscdecl
to interpret a given C declaration and convert it into a more accessible English description.C_declaration
: This placeholder represents the specific C declaration you want to interpret. It allows the user to understand the structure and type of a given piece of code.
Example output:
For the C declaration int (*func_ptr[])()
, cdecl
could return:
array of pointer to function returning int
In this instance, the cdecl
tool helps to demystify the C syntax by translating it into clear, human-readable language that describes the declaration accurately.
Use case 3: Cast a variable to another type
Code:
cdecl cast variable_name to type
Motivation:
Casting is a frequent necessity in C and C++ programming, often required to convert one data type into another for compatibility or functionality purposes. This use case assists in constructing the correct casting syntax, ensuring both clarity and precision in the code.
Explanation:
cast
: This command instructscdecl
to generate a casting expression.variable_name
: This represents the name of the variable you wish to cast. It specifies what you are working with and reduces ambiguity in code translation.to type
: This part specifies the target data type you want to cast the variable to, providing clarity on the transformation being performed.
Example output:
If you input cdecl cast x to float
, the output might be:
(float)x;
This shows how cdecl
automatically structures a valid C cast expression, ensuring your syntax is correct and reducing the likelihood of casting errors.
Use case 4: Run in interactive mode
Code:
cdecl -i
Motivation:
Interactive mode is particularly beneficial for programmers who want to work through multiple C and C++ declarations or queries in one session without launching multiple instances or processes. It allows real-time feedback and interaction with cdecl
, making it a flexible tool during coding sessions.
Explanation:
-i
: This flag stands for “interactive.” It enables an ongoing session in which the user may enter multiple commands or queries without restarting the tool.
Example output:
When running cdecl
in interactive mode, the console remains open, and you can input various commands like explain
or compose
. Each command is processed immediately, with cdecl
providing output or results in response to these interactional inputs, streamlining workflow efficiency.
Type queries/commands here:
cdecl> explain char **argv
cdecl> array of pointer to char
cdecl> cast y to double
cdecl> (double)y;
This interactive session offers a seamless environment for exploring and understanding complex C/C++ syntax.
Conclusion:
The cdecl
command-line tool simplifies a programmer’s task by transforming complex C/C++ declarations to and from plain English. With capabilities spanning interactive mode operation, composing new declarations, explaining existing ones, and casting variables, cdecl
is an essential companion for both seasoned and novice developers working in these programming languages.