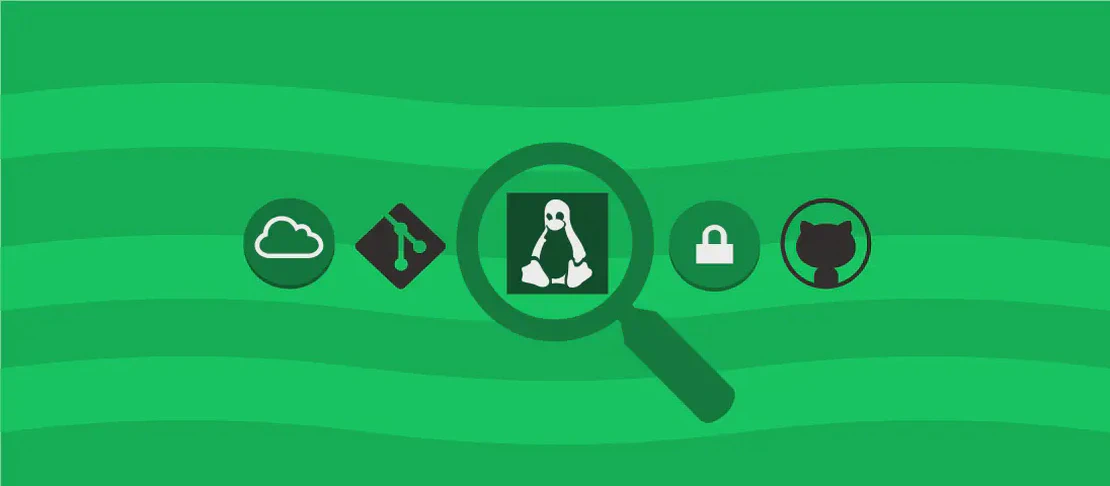
Mastering the AWS Cloud Development Kit CLI (with examples)
The AWS Cloud Development Kit (CDK) is a powerful tool that allows developers to define cloud infrastructure using code. The CDK CLI enables the process of building applications with AWS CDK swift and efficient by providing a command-line interface to interact with AWS CloudFormation and streamline various cloud operations. Through the CLI, developers can manage resources, deploy and maintain stacks, and achieve complex infrastructure configurations easily.
Use case 1: Listing the Stacks in Your Application
Code:
cdk ls
Motivation: Listing stacks is a fundamental task that you might want to perform to understand what your application currently consists of. Whether you’re managing multiple projects or ensuring that the correct infrastructure is in place, ‘cdk ls’ provides a quick overview of all stacks in your AWS CDK application, allowing you to verify the components at a glance.
Explanation:
The cdk ls
command is straightforward with no additional arguments necessary. It simply lists all the deployed stacks associated with your application, giving you a concise view of the current infrastructure setup.
Example Output:
MyFirstStack
DataProcessingStack
WebServerStack
Use case 2: Synthesizing a CloudFormation Template from a CDK Stack
Code:
cdk synth stack_name
Motivation:
The cdk synth
command allows you to view the AWS CloudFormation template generated from your CDK stack. This is essential for understanding what AWS resources will be created or updated when deploying, enabling you to inspect the infrastructure as code before actual deployment. This preview aids in preventing errors by allowing a thorough review of configurations.
Explanation:
synth
: This part of the command refers to “synthesize,” which means transforming the CDK application code into an intermediate AWS CloudFormation template.stack_name
: You should replacestack_name
with the actual name of the stack whose template you want to inspect. This specifies the exact stack from your application for which the CloudFormation template will be generated.
Example Output:
Resources:
MyBucketF68F3FF0:
Type: "AWS::S3::Bucket"
MyQueueD7E3AB1C:
Type: "AWS::SQS::Queue"
Use case 3: Deploying One or More Stacks
Code:
cdk deploy stack_name1 stack_name2
Motivation:
Deploying stacks is a critical operation in cloud development as it applies your infrastructure updates or newly added components to the AWS environment. The cdk deploy
command enables you to execute these actions securely and efficiently, applying changes that have been reviewed and synthesized. This command streamlines the deployment process, reducing manual intervention in setting up cloud resources.
Explanation:
deploy
: This directs the CDK CLI to start deployment to AWS.stack_name1 stack_name2
: Listing multiple stack names means you want to deploy multiple stacks simultaneously. This is useful if your application has dependencies between stacks or if you want to orchestrate updates across different parts of your infrastructure.
Example Output:
Stack ARN:
arn:aws:cloudformation:region:account-id:stack/MyFirstStack/uuid
Outputs:
MyFirstStack.EndpointURL = http://myfirststack-bucket.s3-website.region.amazonaws.com
Use case 4: Destroying One or More Stacks
Code:
cdk destroy stack_name1 stack_name2
Motivation:
The need to destroy stacks arises when you want to clean up unused resources or remove parts of your infrastructure that are no longer needed. The cdk destroy
command automates this process, ensuring that resources are properly terminated, while preventing unnecessary costs associated with unused AWS services. It’s particularly useful in environments where infrastructure needs to be transient, such as in development or testing scenarios.
Explanation:
destroy
: Commanding the CLI to delete resources associated with specified stacks.stack_name1 stack_name2
: Specifies which stacks are to be destroyed, ensuring that the process affects only the intended segments of your infrastructure.
Example Output:
Are you sure you want to delete: MyFirstStack (y/n)? y
MyFirstStack: destroying...
Destroy complete.
Use case 5: Comparing Local and Deployed Stacks
Code:
cdk diff stack_name
Motivation:
Comparison between deployed and local stack configurations is pivotal for detecting discrepancies and understanding the impact of changes before deployment. The cdk diff
command allows developers to visualize differences between their working code and the current live stack, serving as a preventive step against mistakes that could lead to unintentional infrastructure changes.
Explanation:
diff
: This part of the command initiates a differential analysis.stack_name
: Indicates which stack you want to compare. The command reveals changes in resources, properties, or any new elements between what’s proposed in the stack code and what’s currently deployed.
Example Output:
Stack MyAppStack
Resources
[~] AWS::S3::Bucket MyBucket MyBucketF68F3FF0
+ CORS: [{"AllowedMethods":["GET"]...}]
Use case 6: Creating a New CDK Project
Code:
cdk init -l python
Motivation:
Starting a new AWS CDK project is the first step towards building infrastructure with code. The cdk init
command initializes the project environment according to the specified programming language, setting up necessary boilerplate files and directories. This jumpstarts the development process, helping you to focus on the custom logic and infrastructure setup without manually orchestrating foundational project structures.
Explanation:
init
: Indicates initialization of a new CDK project.-l python
: The-l
flag specifies the programming language to be used for the CDK project. In this example, it’s set to Python, but other languages such as TypeScript, JavaScript, Java, C#, and others can be specified.
Example Output:
Initializing a new CDK project in Python...
Use case 7: Opening the CDK API Reference in Your Browser
Code:
cdk docs
Motivation:
Accessing the CDK API documentation quickly can significantly enhance productivity. The cdk docs
command automatically opens the default browser to the AWS CDK API reference page, facilitating easy exploration and understanding of different classes, modules, and properties offered by the toolkit. This is an invaluable resource for both new and seasoned developers looking to maximize their use of the CDK.
Explanation:
docs
: A command that tells the CLI to open the official AWS CDK documentation in a web browser. This saves time that would otherwise be spent searching for the right documentation manually.
Example Output:
Launching web browser with AWS CDK documentation...
Conclusion:
The AWS CDK CLI commands provide an incredible range of functionalities that streamline the process of infrastructure management in AWS. By gaining a solid understanding of these commands, developers can deploy, manage, and configure complex cloud environments efficiently. Each command—whether deploying stacks, inspecting configurations, or initializing new projects—works to refine the cloud development process, enabling powerful infrastructure operations with ease.