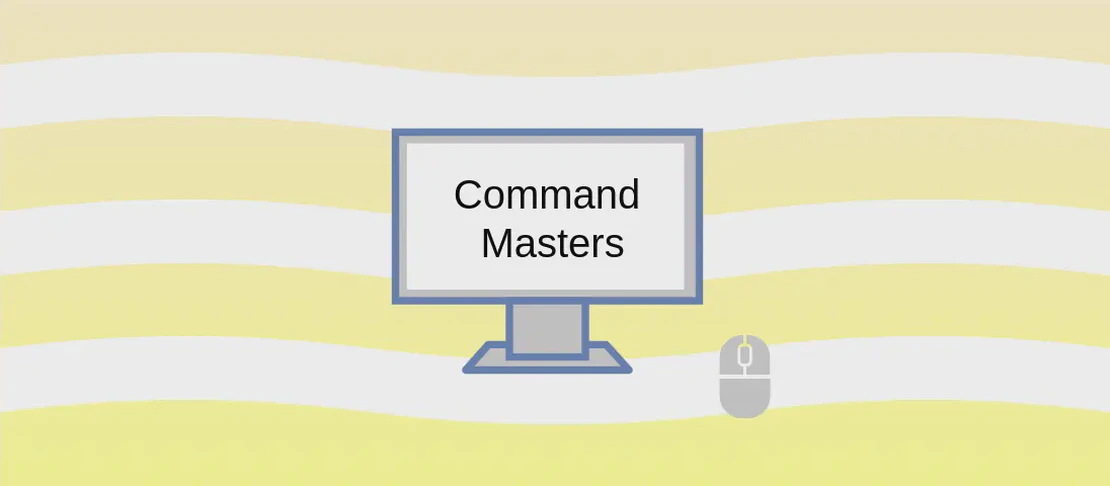
How to Use the Command 'cf' (with Examples)
The ‘cf’ command is a powerful tool for managing applications and services on Cloud Foundry, a popular open-source platform-as-a-service (PaaS). By utilizing this command-line interface (CLI), developers can deploy, scale, and maintain applications seamlessly. The command allows for a wide range of operations such as logging into the Cloud Foundry environment, deploying applications, managing services, and monitoring application performance through logs.
Use Case 1: Log in to the Cloud Foundry API
Code:
cf login -a api_url
Motivation:
Logging into the Cloud Foundry API is a crucial first step because it establishes a secure connection between your local environment and the Cloud Foundry platform where your applications will reside. This command verifies your credentials and grants you access to the resources and services provided by Cloud Foundry.
Explanation:
cf
: The command-line interface being used.login
: The sub-command instructing ‘cf’ to initiate the login process.-a api_url
: Specifies the API endpoint URL of the Cloud Foundry environment you are logging into. This URL is essential because it identifies the specific instance of Cloud Foundry that you wish to access.
Example Output:
API endpoint: api.run.pivotal.io
Email> john.doe@example.com
Password>
Authenticating...
OK
Targeted org john-org
Targeted space development-space
API endpoint: https://api.run.pivotal.io (API version: 2.136.0)
User: john.doe@example.com
Org: john-org
Space: development-space
Use Case 2: Push an App Using the Default Settings
Code:
cf push app_name
Motivation:
Pushing an app to Cloud Foundry is a fundamental operation that deploys your code into a live environment. This command makes it possible to deliver new features or updates quickly and efficiently by using Cloud Foundry’s default configuration settings. It simplifies the deployment process, ideally suited for developers looking for quick start-ups without delving deep into custom configurations.
Explanation:
cf
: Indicates the use of the Cloud Foundry command-line interface.push
: Tells ‘cf’ to deploy or update an application.app_name
: The desired name for your application instance within Cloud Foundry. It needs to be unique across your development space to avoid conflicts.
Example Output:
Pushing from manifest to org john-org / space development-space as john.doe@example.com...
Using manifest file /path/to/manifest.yml
Getting app info...
Creating app with these attributes...
+ name: app_name
path: /path/to/app
buildpacks: some_buildpack
command: some_command
disk quota: 1G
health check type: process
instances: 1
memory: 512M
Creating app app_name...
Mapping routes...
Comparing local files to remote cache...
Packaging files to upload...
Uploading files...
OK
Stopping app...
Starting app...
Use Case 3: View the Services Available from Your Organization
Code:
cf marketplace
Motivation:
Being aware of the services available in your Cloud Foundry organization is vital for integrating additional functionality into your applications. This command lists the various add-on services that can be used, such as databases, messaging queues, and monitoring tools, enabling developers to augment their app’s capabilities with minimal setup.
Explanation:
cf
: Specifies the use of the Cloud Foundry CLI.marketplace
: Queries the available services within your organization, providing a list of service offerings you can utilize.
Example Output:
Getting services from marketplace...
service plans description
mysql 100mb, 1gb, 5gb, 10gb MySQL Database
rabbitmq standard, custom RabbitMQ Messaging Service
redis cache, persistent Redis In-Memory Data Structure Store
Use Case 4: Create a Service Instance
Code:
cf create-service service plan service_name
Motivation:
Creating a service instance within Cloud Foundry is an essential task when you want to provide backing services such as databases or caches to your applications. This command helps in provisioning a specific service plan that suits your application’s needs, automatically configuring it to be ready for use.
Explanation:
cf
: Denotes the use of the Cloud Foundry CLI.create-service
: Instructs ‘cf’ to establish a new instance of a specified service.service
: The type of service you wish to provision (e.g., MySQL, Redis).plan
: The specific plan to use, which dictates the performance and cost parameters (e.g., standard, premium).service_name
: The unique identifier for this instance, which should be used when binding the service to an application.
Example Output:
Creating service instance mysql-instance in org john-org / space development-space as john.doe@example.com...
OK
Create in progress. Use 'cf services' or 'cf service mysql-instance' to check operation status.
Use Case 5: Connect an Application to a Service
Code:
cf bind-service app_name service_name
Motivation:
Binding a service to an application connects the two, allowing the application to utilize the capabilities of the service instance (e.g., a database or an analytics service). This is a pivotal step in ensuring that your application can communicate with third-party or platform-provided services, which can amplify its functionality.
Explanation:
cf
: Specifies the use of the Cloud Foundry CLI.bind-service
: Indicates to Cloud Foundry that you want to connect an application to a service.app_name
: The application that will be bound to the service.service_name
: The service instance that needs to be connected to the application.
Example Output:
Binding service mysql-instance to app_name in org john-org / space development-space as john.doe@example.com...
OK
TIP: Use 'cf restage app_name' to ensure your env variable changes take effect
Use Case 6: Run a Script Whose Code is Included in the App, but Runs Independently
Code:
cf run-task app_name "script_command" --name task_name
Motivation:
Running tasks independently from the main application process allows for executing background jobs, maintenance scripts, or one-off operations without affecting the primary app functionality. This capability is crucial for performing database migrations, data processing, or similar tasks that are not part of the regular application execution flow.
Explanation:
cf
: Indicates the Cloud Foundry CLI being used.run-task
: Facilitates the execution of a standalone task in the context of the specified application.app_name
: The application context under which the task will run.script_command
: The actual command or script that you intend to execute.--name task_name
: An optional parameter to name the task, allowing for easier reference and management.
Example Output:
Creating task for app app_name in org john-org / space development-space as john.doe@example.com...
OK
Task has been submitted successfully for execution.
Use Case 7: Start an Interactive SSH Session with a VM Hosting an App
Code:
cf ssh app_name
Motivation:
Establishing an SSH session with a virtual machine hosting your application is invaluable for debugging and inspecting your app’s runtime environment. This feature allows developers to troubleshoot issues directly on the instance hosting their application, enabling faster resolutions and deeper insights.
Explanation:
cf
: Specifies the use of the Cloud Foundry CLI.ssh
: Initiates a secure shell session with the VM instance running your application.app_name
: The application whose hosting VM will be accessed for SSH.
Example Output:
Invoking 'ssh' app_name in org john-org / space development-space as john.doe@example.com...
Welcome to the Cloud Foundry SSH Terminal!
~ >
Use Case 8: View a Dump of Recent App Logs
Code:
cf logs app_name --recent
Motivation:
Reviewing recent application logs is critical for monitoring application performance, diagnosing errors, and tracking operational issues. This command provides a snapshot of your application’s log output, enabling you to quickly pinpoint problems or just verify that everything is functioning as expected.
Explanation:
cf
: The Cloud Foundry CLI being utilized.logs
: Requests the log output for a specified app.app_name
: The application whose logs you wish to view.--recent
: An optional argument requesting only the most recent logs, making it easier for users to focus on current issues or changes without sifting through older logs.
Example Output:
Retrieving logs for app app_name in org john-org / space development-space as john.doe@example.com...
OUT 2023-10-01T15:32:45.12+0000 [APP/PROC/WEB/0] OUT Starting application...
OUT 2023-10-01T15:32:46.45+0000 [APP/PROC/WEB/0] OUT Server running on port 8080...
...
Conclusion:
The ‘cf’ command-line interface is an indispensable asset for developers working with Cloud Foundry, offering a versatile array of functions for efficient cloud-based application management. Through its straightforward but powerful commands, users can log in to different environments, deploy and manage apps, bind services, execute auxiliary tasks, and even dive into logs or live SSH sessions for troubleshooting. Mastering these commands not only boosts development productivity but also strengthens an application’s ability to adapt and scale in dynamic cloud ecosystems.