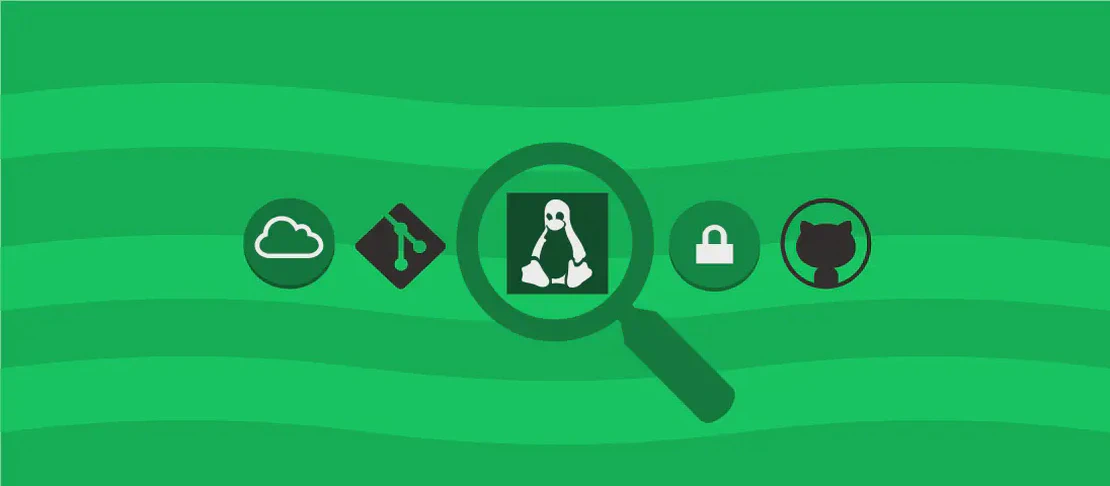
How to use the command 'clang++' (with examples)
Clang++ is a compiler tool that is a part of the LLVM project, designed specifically for compiling C++ source files. As a high-performance compiler, it is known for its advanced diagnostics, which help developers identify and fix code issues efficiently. Clang++ is frequently used for compiling C++ projects, optimizing code, analyzing code structure, and performing cross-platform development. It complies with the latest C++ standards ensuring robust support for modern C++ features.
Use case 1: Compile a set of source code files into an executable binary
Code:
clang++ path/to/source1.cpp path/to/source2.cpp -o path/to/output_executable
Motivation:
The primary motivation for this use case is the need to transform human-readable C++ source files into a machine-readable executable. This is a fundamental task for any C++ developer seeking to run their application. After writing a program in C++, the developer needs to compile the source code files to produce a runnable binary. This is essential for testing, deploying, and distributing software.
Explanation:
clang++
: This invokes the Clang++ compiler to process the provided C++ files.path/to/source1.cpp path/to/source2.cpp
: These are the source code files that contain the program logic and are to be compiled.-o path/to/output_executable
: This option specifies the desired name and location of the output executable file. The-o
flag indicates that what follows is the output file path.
Example Output:
After running the above command, you should see no output if the compilation is successful. However, you should see an executable file created at path/to/output_executable
. If there are errors in the code, the Clang++ compiler will display the errors and warnings indicating what issues need to be resolved.
Use case 2: Activate output of all errors and warnings
Code:
clang++ path/to/source.cpp -Wall -o output_executable
Motivation:
Activating all errors and warnings is critical for maintaining high code quality. Newbie and experienced programmers alike benefit from compiling with warnings enabled as it helps identify potential issues that might not necessarily stop the compile process but could lead to runtime errors or undefined behavior. This practice aids in debugging and adhering to best programming practices.
Explanation:
clang++
: Invokes the Clang++ compiler to compile the given C++ file.path/to/source.cpp
: This is the single source file specified for the compilation process.-Wall
: This is a compiler flag that prompts Clang++ to output all warnings during compilation. It is tremendously helpful for identifying possible coding problems.-o output_executable
: Specifies the creation of an output executable with no specified path, so it will be created in the current directory.
Example Output:
The compiler will display all warnings in addition to errors found in path/to/source.cpp
. This helps the developer to correct code warnings that could become potential issues later, allowing for thorough refinement of the source code. Successful compilation results in output_executable
.
Use case 3: Show common warnings, debug symbols in output, and optimize without affecting debugging
Code:
clang++ path/to/source.cpp -Wall -g -Og -o path/to/output_executable
Motivation:
This use case addresses the need to debug and optimize the program simultaneously. The balancing act between maintaining debuggability and optimizing code can be crucial during development, especially when diagnosing complex issues or incrementally improving performance without losing the ability to debug effectively.
Explanation:
clang++
: Calls upon the Clang++ compiler to compile the C++ source file.path/to/source.cpp
: The source code file that you wish to compile.-Wall
: Triggers the output of all warnings for better code quality checks.-g
: Directs the compiler to generate debug information for the compiled program, enabling the use of a debugger to troubleshoot the code.-Og
: This flag optimizes the program in a manner that does not interfere with debugging, providing a compromise between no optimization and debugging information.-o path/to/output_executable
: Instructions for naming and positioning the generated executable file.
Example Output:
Should compile errors exist, they are printed to the console. Successful compilation leads to path/to/output_executable
creation. When linked to a debugger, the executable will retain optimization and debug symbols, ensuring ease of debugging.
Use case 4: Choose a language standard to compile for
Code:
clang++ path/to/source.cpp -std=c++20 -o path/to/output_executable
Motivation:
C++ continually evolves, introducing new standards that enhance functionality, performance, and safety. Specifying a language standard ensures that the compiler applies appropriate rules and features introduced in the version. It enables developers to take advantage of modern styles, types, and libraries while ensuring consistent behavior.
Explanation:
clang++
: The Clang++ compiler directive to compile a C++ file.path/to/source.cpp
: This is the input C++ file for compilation.-std=c++20
: Option for specifying the use of the C++20 standard, which introduces new language and library features.-o path/to/output_executable
: Output binary configuration, indicating where and under what name the executable file is generated.
Example Output:
If the source code uses C++20-specific features, this command ensures they are interpreted correctly. Successful compliance with the standard results in executing path/to/output_executable
being generated.
Use case 5: Include libraries located at a different path than the source file
Code:
clang++ path/to/source.cpp -o output_executable -Ipath/to/header_path -Lpath/to/library_path -lpath/to/library_name
Motivation:
In many C++ projects, external libraries enhance functionality beyond the standard library offerings. Including libraries from different paths is necessary to leverage specialized functions, extend feature sets, or link to previously compiled code, promoting efficient project modularity and reuse.
Explanation:
clang++
: Calls the Clang++ compiler.path/to/source.cpp
: Specified source file for compilation.-o output_executable
: Designates output executable name and location.-Ipath/to/header_path
: Adds directory to the list where header files are searched, assisting the preprocessor in finding necessary headers.-Lpath/to/library_path
: Specifies the location where the compiler searches for library files during linking.-lpath/to/library_name
: Directs the compiler to link against a specific library by name.
Example Output:
Properly specifying the include and library directories ensures successful linking, creating output_executable
with linked external resources facilitated by specified libraries.
Use case 6: Compile source code into LLVM Intermediate Representation (IR)
Code:
clang++ -S -emit-llvm path/to/source.cpp -o path/to/output.ll
Motivation:
Generating LLVM Intermediate Representation (IR) provides deeper insight into the compiled program’s structure and behavior. it is very useful for optimization analysis and understanding low-level code transformations. It’s especially crucial for developers focusing on compiler development, optimization, and verification.
Explanation:
clang++
: Initiates the use of the Clang++ compiler.-S
: Prompts the compiler to stop after emitting assembly code.-emit-llvm
: Requests the output to be in LLVM’s intermediate representation instead of typical executable format.path/to/source.cpp
: C++ source file targeted for IR generation.-o path/to/output.ll
: Specifies the output file as an LLVM IR file.
Example Output:
Successful generation of LLVM IR is evidenced by path/to/output.ll
creation containing an intermediate representation of the source code, facilitating insights into optimization levels.
Use case 7: Optimize the compiled program for performance
Code:
clang++ path/to/source.cpp -O2 -o path/to/output_executable
Motivation:
Optimizing for performance is crucial if an application demands high-efficiency levels, serving critical industries, from gaming to scientific computation. Performance optimization assures faster execution times and reduced resource usage, crucial for programs under intense computational load or execution constraints.
Explanation:
clang++
: Calls upon Clang++ to compile C++ file.path/to/source.cpp
: Source file slated for optimization during compilation.-O2
: Applies a mid-level optimization strategy that balances code size and execution speed without excessive compilation time.-o path/to/output_executable
: Prescribes output executable’s location and name.
Example Output:
The successful application of -O2 optimization results in path/to/output_executable
, demonstrating improved performance characteristics compared to non-optimized compilations. Timing tests would typically show this enhancement.
Use case 8: Display version
Code:
clang++ --version
Motivation:
Checking the compiler version is essential for developers to ensure compatibility with system requirements, libraries, and specific features. Knowing the version aids in debugging any possible issues that could confer with older or newer standards or hardware.
Explanation:
clang++
: Executes Clang++ tool.--version
: A flag instructing the compiler to display the current version details without performing any file compilation.
Example Output:
Running this command results in a display of Clang++ version information, detailing the release number, target architecture, and other build-specific data confirming the features and capabilities included in this build of Clang++.
Conclusion:
The clang++
tool is highly versatile, accommodating numerous workflows and needs in C++ development. Whether compiling source files, optimizing performance, or extending functionality with libraries, clang++
adapts to diverse development scenarios. Its comprehensive support for modern C++ standards and meticulous diagnostic capabilities ensures it remains a crucial asset in the developer’s toolkit. Mastering its various uses maximizes your efficiency and code quality on all fronts.