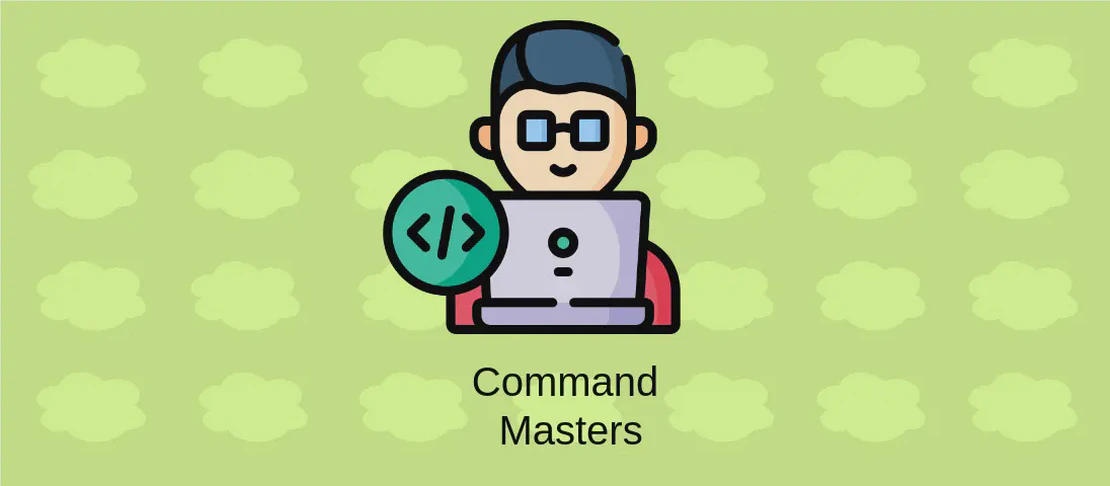
How to use the command 'clang' (with examples)
Clang is a compiler for C, C++, and Objective-C programming languages, and it is part of the LLVM project. Renowned for its fast compile times and superior diagnostics, Clang can also act as a drop-in replacement for GCC, meaning it can execute many of the same tasks, often with enhanced error-checking features. Whether you’re compiling multiple files, optimizing performance, or managing dependencies, Clang’s robust command-line interface offers a variety of options tailored to developers’ needs.
Compile multiple source files into an executable
Code:
clang path/to/source1.c path/to/source2.c ... -o|--output path/to/output_executable
Motivation:
In many projects, your program’s logic is split across multiple source files for better organization and maintenance. Compiling them into a single executable enables you to run and test the full program. By using Clang to compile multiple files simultaneously, you can ensure consistency across the compiled artifacts and potentially shorten the build time.
Explanation:
clang
: The command-line tool used to compile source files.path/to/source1.c path/to/source2.c ...
: The paths to the input source files to be compiled.-o|--output path/to/output_executable
: Specifies the output file’s name or path, often an executable.
Example Output:
When you run the above command, if there are no errors in your C files, you will get a single output file (often an executable). If the output file is named program
, you can run it in the terminal by typing ./program
.
Activate output of all errors and warnings
Code:
clang path/to/source.c -Wall -o|--output output_executable
Motivation:
Warnings often indicate potential issues or bad practices that might not stop the program from running but could lead to bugs or maintenance difficulties later. By enabling Wall`, you ensure a more robust code by addressing these warnings early.
Explanation:
path/to/source.c
: The path to the source code to be compiled.-Wall
: Activates all the commonly used warning messages, aiding in identifying likely coding mistakes.-o|--output output_executable
: Specifies the output filename or path.
Example Output:
When this command is executed, the terminal will display warnings about potential issues in your source file, such as unused variables or suspicious code constructs. The warnings list aids developers in refining their code.
Show common warnings, debug symbols in output, and optimize without affecting debugging
Code:
clang path/to/source.c -Wall -g|--debug -Og -o|--output path/to/output_executable
Motivation:
While debugging, developers require more context about the internal workings of their code. Generating debugging symbols allows you to inspect variable states and program execution flow closely. The -Og
option strikes a balance by optimizing the program for performance but ensures that debugging information remains accurate, so you can follow the code execution as closely as possible to the source code logic.
Explanation:
-g|--debug
: Generates debugging information in the output to be utilized with debuggers like gdb.-Og
: Optimizes the code but without hindering debugging capabilities, providing a smoother debugging experience.-Wall
: As previously mentioned, this turns on all default warnings.
Example Output:
Running this command prepares an executable that can be debugged with tools like gdb. If executed without errors, the output will contain sufficient information for efficient debugging sessions.
Include libraries from a different path
Code:
clang path/to/source.c -o|--output path/to/output_executable -Ipath/to/header -Lpath/to/library -llibrary_name
Motivation:
Projects might depend on external libraries to extend functionality or utilize existing components. When these libraries or headers are not within the standard system directories, specifying their locations ensures that the compiler includes them correctly during the build process.
Explanation:
-Ipath/to/header
: Adds the directory path/to/header` to the list of directories for searching headers.-Lpath/to/library
: Addspath/to/library
to the list of directories where library files are searched during linking.-llibrary_name
: Indicates which external library to link against, identified bylibrary_name
.
Example Output:
This command links your program with specified external libraries and outputs an executable. When you run this executable, it will utilize the libraries you specified in the command.
Compile source code into LLVM Intermediate Representation (IR)
Code:
clang -S|--assemble -emit-llvm path/to/source.c -o|--output path/to/output.ll
Motivation:
LLVM IR is a low-level programming language that is easy for compilers to transform and optimize. Saving your source code in LLVM IR can help in further analysis and optimization phases if needed. It is beneficial when developing language tools or doing advanced compiler research and development work.
Explanation:
-S|--assemble
: Compiles to an assembly code rather than a machine code.-emit-llvm
: Specifies to output in LLVM Intermediate Representation form.-o|--output path/to/output.ll
: Specifies where the output.ll
file should be saved.
Example Output:
The command generates an .ll
file representing the LLVM IR of your source code, which can be inspected and modified for advanced compiler tasks.
Compile source code into an object file without linking
Code:
clang -c|--compile path/to/source.c
Motivation:
By compiling to an object file, you break the build process into compiling and linking phases, which can be useful when dealing with larger projects to separate concerns and save time for unchanged files.
Explanation:
-c|--compile
: Compiles the source file to an object file but stops short of linking.path/to/source.c
: The input source file to compile.
Example Output:
This results in an object file (.o
file) being created for each input source file. The object file can later be linked to form a complete executable using a separate linking command.
Optimize the compiled program for performance
Code:
clang path/to/source.c -O1|2|3|fast -o|--output path/to/output_executable
Motivation:
Optimizing a program for performance means exploiting speed or code size benefits during execution. Different optimization levels like O1, O2, O3, and fast offer varying degrees of enhancement, and choosing the right level can significantly impact runtime efficiency.
Explanation:
-O1|2|3|fast
: Specifies the level of optimization. Higher numbers generally mean higher optimization:-O1
: Some optimizations.-O2
: More optimizations (default).-O3
: Maximal optimizations.-Ofast
: Aggressively optimize without strict compliance to language standards.
Example Output:
With these optimizations, your compiled executable’s performance should improve, noticeable in faster runtime or reduced memory usage, depending on the nature of your application.
Display version
Code:
clang --version
Motivation:
Knowing which version of Clang you’re using can be essential for debugging, comparing features, or ensuring compatibility with system requirements and project specifications.
Explanation:
--version
: An option that outputs all version information related to the Clang tool you are running.
Example Output:
Executing this command provides the version of Clang installed on your system, along with associated LLVM versions. This information aids in ensuring compatibility with other tools or meeting specific project requirements.
Conclusion:
Clang, with its variety of options, provides powerful capabilities for compiling and optimizing C, C++, and Objective-C applications. From checking code quality with warnings to incorporating external libraries, each use case showcases Clang’s flexibility, helping developers not only compile their projects but also enhance debugging, optimize for performance, and manage project dependencies effectively. Armed with these examples, developers can leverage Clang’s rich feature set to meet diverse programming needs.