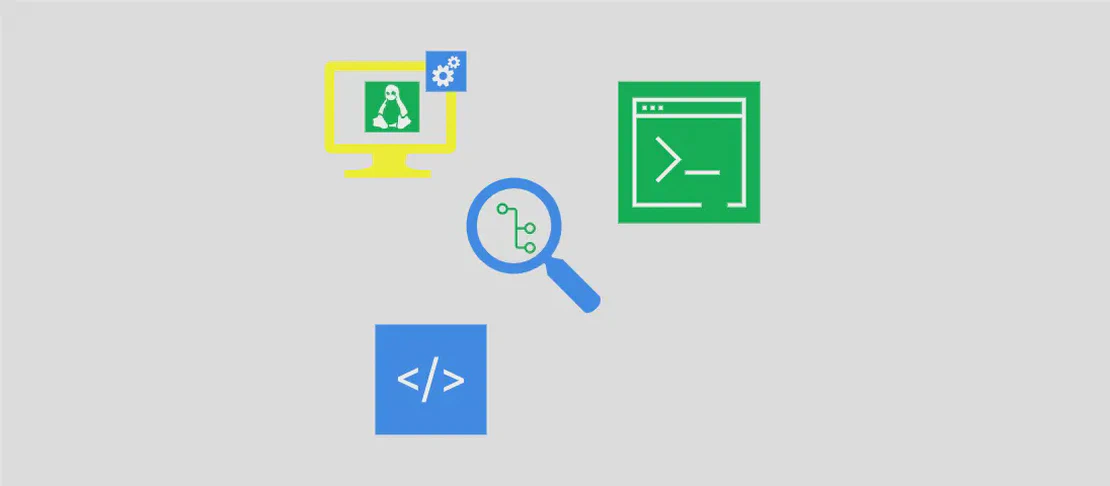
How to Use the Command 'clang-format' (with examples)
Clang-Format is a versatile tool widely used by developers to automatically format source code written in languages like C, C++, Java, JavaScript, Objective-C, Protobuf, and C#. This utility helps maintain consistent coding styles across large codebases, making the code more readable and easier to maintain. It transforms unformatted or inconsistently formatted code into a neat and organized form by adhering to coding style rules.
Use case 1: Format a file and print the result to stdout
Code:
clang-format path/to/file
Motivation:
Using this command is ideal when you wish to quickly see what changes would be applied to a particular source file without permanently modifying it. This is especially handy during code reviews or when experimenting with different formatting styles where viewing potential formatting helps decide whether or not to apply it.
Explanation:
clang-format
: This launches the Clang-Format tool.path/to/file
: Replace this with the actual path of the file you wish to format. The command reads this file, applies the formatting rules, and outputs the formatted code directly to the console (stdout).
Example Output:
When applied to a C++ file, you might see unformatted lines neatly aligned with consistent indentation, spacing, and line breaks.
int main() { std::cout << "Hello, World!"; }
Use case 2: Format a file in-place
Code:
clang-format -i path/to/file
Motivation:
This command is useful when you want to apply formatting directly to your source file without needing to manually view or compare changes. It’s a preferred option during automated processes, such as pre-commit hooks or batch formatting, where files are required to conform to style guidelines before being committed to a code repository.
Explanation:
-i
: This option tells Clang-Format to edit the file in place.path/to/file
: Specifies the file’s path which will be formatted and over-written with Clean-Format’s output.
Example Output:
The original file is reformatted in place, so opening the file afterwards will show changes like aligned braces and properly spaced operators.
int main()
{
std::cout << "Hello, World!";
}
Use case 3: Format a file using a predefined coding style
Code:
clang-format --style Google path/to/file
Motivation:
This command is particularly beneficial for teams or projects that adhere to a popular coding standard, such as Google’s C++ style guide. By using a predefined coding style, you ensure a common style is enforced, enhancing readability and maintainability across the codebase.
Explanation:
--style
: This option specifies the predefined style to use for formatting. Clang-Format includes several popular styles, such as LLVM, GNU, Google, Chromium, Microsoft, Mozilla, and WebKit.Google
: This is an example predefined style. Choose any of the available styles that suit your project’s needs.path/to/file
: Path to the file that should be formatted according to the Google style guide.
Example Output:
A file will be reformatted according to the rules defined in the Google coding standard, including specific indentation levels, brace alignment, and space handling.
int main() {
std::cout << "Hello, World!";
}
Use case 4: Format a file using the .clang-format
file in one of the parent directories of the source file
Code:
clang-format --style=file path/to/file
Motivation:
This approach is beneficial for projects with custom style requirements documented in their own .clang-format
file. It allows teams to define and maintain a unique set of formatting rules that might not fit any of the standard preset styles, ensuring the entire codebase conforms to bespoke standards.
Explanation:
--style=file
: This option directs Clang-Format to use the project’s.clang-format
configuration file to enforce custom formatting rules.path/to/file
: This is the file to be formatted. Clang-Format will search for the.clang-format
configuration in the file’s directory hierarchy and apply those specific rules.
Example Output:
With a custom style, the file might show unique aspects of formatting such as distinct indentation rules or line splitting that reflect the project’s personalized requirements.
int main()
{
/* customized formatting */
std::cout << "Hello, World!";
}
Use case 5: Generate a custom .clang-format
file
Code:
clang-format --style Google --dump-config > .clang-format
Motivation:
This command is invaluable when first setting up a project or switching to a consistent code format. By generating a .clang-format
file that matches one of the predefined styles, you provide a baseline that developers can build upon, customizing further to meet unique project standards.
Explanation:
--style Google
: This indicates the base style from which to dump the current configuration. Any config tweaks will begin from this template.--dump-config
: This option instructs Clang-Format to output the current style configuration settings.> .clang-format
: Redirects the command’s output into a.clang-format
file for persisting the style settings.
Example Output:
The output is saved directly as a configuration file named .clang-format
, which can then be used to apply the exported style to the project files.
---
BasedOnStyle: Google
IndentWidth: 4
ColumnLimit: 80
...
Conclusion:
Clang-Format offers a robust solution for enforcing coding styles and enhancing code clarity across various programming languages. Whether you seek quick checks, in-place formatting, or custom style enforcement, Clang-Format equips developers with the tools needed to maintain and improve code quality systematically. Embrace these use cases to optimize your coding workflows and ensure your codebase remains clean, consistent, and maintainable.