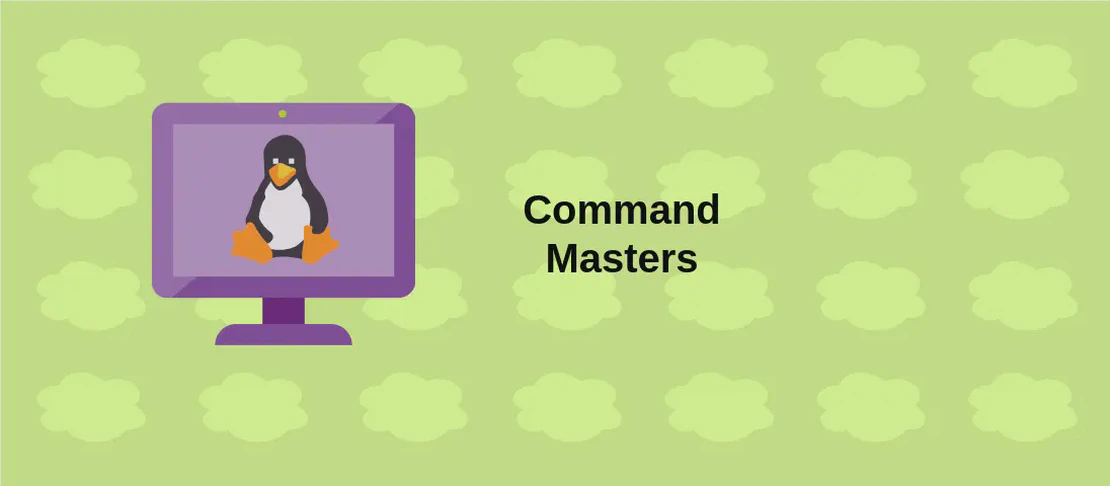
How to Harness 'clang-tidy' for C/C++ Code Optimization (with examples)
Clang-tidy is a versatile static analysis tool that forms part of the LLVM project. It provides C/C++ developers with an efficient way to detect style violations, potential bugs, and security vulnerabilities within their codebase. By integrating static analysis into your development workflow, clang-tidy empowers programmers to produce cleaner, more secure, and maintainable code. The tool is especially valuable because it runs across different platforms and integrates well with other components in the LLVM ecosystem. Below, we’ll explore various use cases to demonstrate the power and versatility of clang-tidy.
Use case 1: Run default checks on a source file
Code:
clang-tidy path/to/file.cpp
Motivation:
Running default checks on a source file allows developers to quickly perform a comprehensive analysis on a file using clang-tidy’s preset configuration. This is beneficial for those who are just beginning to integrate clang-tidy into their workflow or who need a quick pass to catch common issues in their code.
Explanation:
path/to/file.cpp
: This argument specifies the path to the C++ source file that clang-tidy will analyze. By providing this path, clang-tidy knows which file to examine for coding style and potential issues.
Example output:
The output will include warnings or notes about potential issues found in the code. It may look something like this:
path/to/file.cpp:11:10: warning: initialization of 8-byte variable 'x' with '5' is illegible and could be incorrect [bugprone-integer-division]
Use case 2: Don’t run any checks other than the cppcoreguidelines
checks on a file
Code:
clang-tidy path/to/file.cpp -checks=-*,cppcoreguidelines-*
Motivation:
Sometimes, the developer may want to focus exclusively on ensuring that the code adheres to the C++ Core Guidelines without being distracted by other checks. This can help streamline the process of adherence to best practices defined within the C++ Core Guidelines.
Explanation:
path/to/file.cpp
: Similar to the previous use case, this specifies the file to be analyzed.-checks=-*
: This option disables all the default checks. The-*
effectively clears the existing list, so no checks are performed unless specified otherwise.cppcoreguidelines-*
: This enables all checks that start with “cppcoreguidelines-”. The clang-tidy tool will only perform checks relevant to the C++ Core Guidelines.
Example output:
The output will include only issues related to cppcoreguidelines, as in:
path/to/file.cpp:22:5: warning: loop variable 'element' of type 'const Type &' creates a copy from type 'Type' [cppcoreguidelines-pro-bounds-constant-array-index]
Use case 3: List all available checks
Code:
clang-tidy -checks=* -list-checks
Motivation:
Before customizing analysis with clang-tidy, developers may want to view all the checks available. This helps in understanding the wide array of options available to tailor the static analysis to meet their specific requirements.
Explanation:
-checks=*
: This enables all possible checks in clang-tidy to be listed.-list-checks
: Rather than analyzing a file, this instructs clang-tidy to simply list the checks that are available.
Example output:
The clang-tidy command will list all available checks, providing detailed information like:
Available checks:
abseil-*
android-*
boost-*
bugprone-*
...
Use case 4: Specify defines and includes as compilation options
Code:
clang-tidy path/to/file.cpp -- -Imy_project/include -Ddefinitions
Motivation:
During the analysis of C/C++ code, clang-tidy needs to properly parse it as it would be by the compiler. Sometimes the source code depends on certain macros and include paths for successful compilation. This use case configures the analysis environment to match the build environment.
Explanation:
path/to/file.cpp
: Indicates the file to be analyzed.--
: This separates clang-tidy options from the compilation flags. Everything following--
is considered part of the compiler invocation.-Imy_project/include
: Adds an include directory. This path helps clang-tidy find header files referenced in the source code.-Ddefinitions
: Defines macro substitutions for any preprocessor definitions expected by the source code.
Example output:
If there were included headers or macro definitions missing from the setup, the output may contain notes like:
path/to/file.cpp:8:10: note: 'MY_MACRO' macro was not defined [-Wunused-macros]
Conclusion:
Incorporating ‘clang-tidy’ into your development process can significantly enhance the quality of your C/C++ code by providing thorough static analysis. Whether you’re a beginner looking to get a handle on basic style issues or an experienced developer honing in on specific guidelines, clang-tidy offers flexible configurations to suit your needs. By understanding these use cases, you can effectively integrate this powerful tool to promote cleaner, more readable, and more maintainable code in your projects.