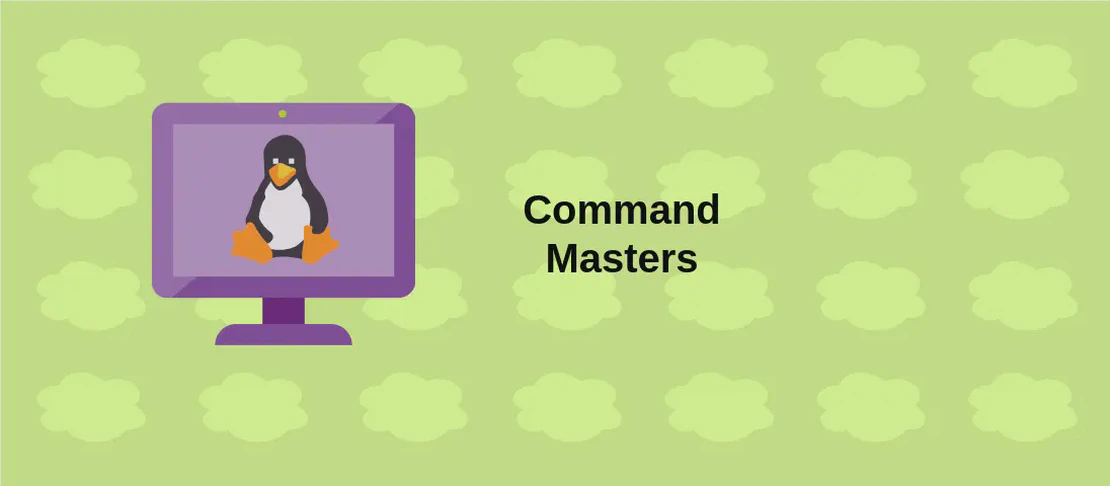
How to use the command clj (with examples)
The clj
command is a tool provided by Clojure that allows you to start a REPL (Read-Evaluate-Print Loop) or invoke a specific function with data. It is a versatile command that can be used in various scenarios to interact with Clojure projects.
Use case 1: Start a REPL (interactive shell)
Code:
clj
Motivation: Starting a REPL allows you to interactively experiment and evaluate Clojure expressions. It is useful for quickly testing code snippets, exploring libraries, and debugging.
Explanation:
The clj
command without any additional arguments starts a REPL, which provides an interactive shell where you can enter and evaluate Clojure expressions.
Example output:
nREPL server started on port 60502 on host 127.0.0.1 - nrepl://127.0.0.1:60502
REPL-y 0.4.4, nREPL 0.10.0
Clojure 1.10.3
Java HotSpot(TM) 64-Bit Server VM 15.0.2+7-27
Docs: (doc function-name-here)
(find-doc "part-of-name-here")
Source: (source function-name-here)
Javadoc: (javadoc java-object-or-class-here)
Exit: Control+D or (exit) or (quit)
Results: Stored in vars *1, *2, *3, an exception in *e
user=>
Use case 2: Execute a function
Code:
clj -X namespace/function_name
Motivation: Invoking a specific function with data allows you to run a particular piece of code without starting a full REPL. It is useful for running scripts or performing specific tasks defined within a namespace.
Explanation:
The -X
option tells the clj
command to execute a specific function in the given namespace. You provide the namespace and the function name separated by a forward slash (/).
Example output:
Hello, Clojure!
Use case 3: Run the main function of a specified namespace
Code:
clj -M -m namespace args
Motivation: Running the main function of a namespace allows you to execute the entry point of a Clojure program. It is useful for running standalone applications or projects.
Explanation:
The -M
option tells the clj
command to run the main function. You need to provide the namespace that contains the main function and any additional arguments required by the program.
Example output:
Application started!
Use case 4: Prepare a project by resolving dependencies, downloading libraries, and making/caching classpaths
Code:
clj -P
Motivation: Preparing a project by resolving dependencies and making/caching classpaths is necessary when working with Clojure projects that rely on external libraries. It ensures that all required dependencies are available and accessible.
Explanation:
The -P
option tells the clj
command to prepare the project. It resolves dependencies, downloads libraries, and creates or updates classpaths as necessary.
Example output:
Dependencies resolved.
Libraries downloaded.
Classpaths created/updated.
Use case 5: Start an nREPL server with the CIDER middleware
Code:
clj -Sdeps '{:deps {nrepl {:mvn/version "0.7.0"} cider/cider-nrepl {:mvn/version "0.25.2"}}}' -m nrepl.cmdline --middleware '["cider.nrepl/cider-middleware"]' --interactive
Motivation: Starting an nREPL server with the CIDER middleware allows you to connect to an nREPL session from editors like Emacs or use tools that integrate with nREPL, such as CIDER.
Explanation:
The -Sdeps
option specifies the dependencies required for the nREPL server and CIDER middleware. The nrepl
and cider/cider-nrepl
libraries are specified with their respective versions using Maven coordinates. The -m
option specifies the main namespace (nrepl.cmdline
) to be run. The --middleware
option specifies the CIDER middleware to be included. The --interactive
option starts the REPL in interactive mode.
Example output:
nREPL server started on port 7888.
Use case 6: Start a REPL for ClojureScript and open a web browser
Code:
clj -Sdeps '{:deps {org.clojure/clojurescript {:mvn/version "1.10.758"}}}' --main cljs.main --repl
Motivation: Starting a REPL for ClojureScript allows you to interactively develop and test ClojureScript code. By opening a web browser, you can see the results of the code directly.
Explanation:
The -Sdeps
option specifies the dependency required for ClojureScript (org.clojure/clojurescript
). The --main
option specifies the main namespace (cljs.main
) to be run. The --repl
option starts the REPL for ClojureScript.
Example output:
ClojureScript 1.10.758
cljs.user=>
Conclusion:
The clj
command is a versatile tool that provides various use cases to interact with Clojure projects. From starting a REPL to running functions and preparing projects, the command provides flexibility and convenience for Clojure development.