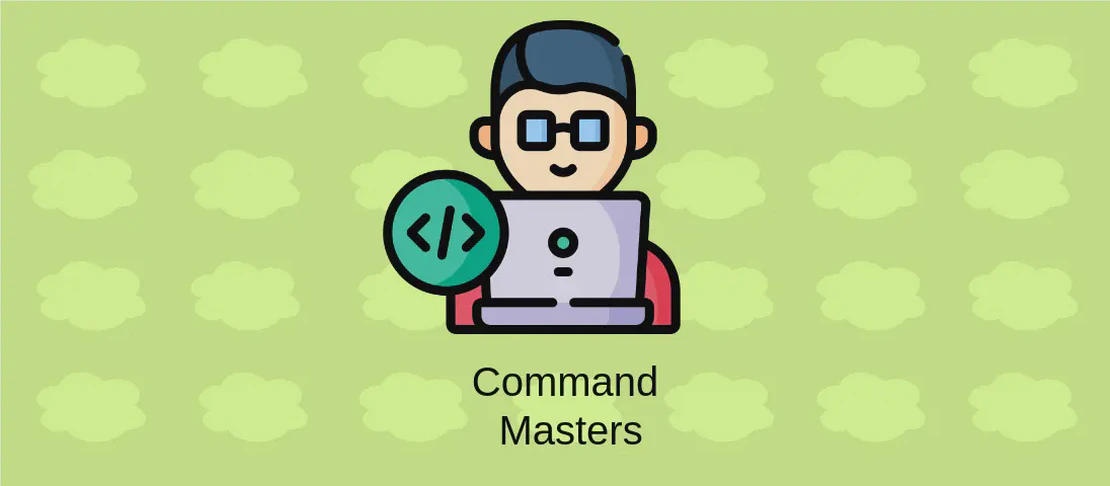
How to Use the Command `cloc` (with Examples)
cloc
is a versatile command-line tool designed to efficiently count the number of lines of code (LOC) in a set of files or an entire directory. It is particularly useful for developers who want to analyze codebases, track productivity, or determine the size of software projects. The tool supports multiple programming languages and distinguishes between code lines, comment lines, and blank lines. Additionally, cloc
can be used to compare differences between two directory structures. Below, we explore several important use cases of cloc
along with detailed motivation and explanations.
Use Case 1: Count all the lines of code in a directory
Code:
cloc path/to/directory
Motivation:
This use case is fundamental for developers or project managers who want to get a quick overview of the size of their codebase. By counting all the lines of code in a directory, you can quantify the amount of coding work done, which can be an indicator of project complexity or progress over time. It’s also useful for comparing the size of different projects.
Explanation:
cloc
: Invokes thecloc
command-line tool.path/to/directory
: This argument specifies the directory whose files you want to analyze. It should be replaced with the actual path of the directory of interest.
Example Output:
-------------------------------------------------------------------------------
Language files blank comment code
-------------------------------------------------------------------------------
Python 5 120 150 970
JavaScript 3 85 60 450
HTML 2 30 25 220
-------------------------------------------------------------------------------
TOTAL 10 235 235 1640
-------------------------------------------------------------------------------
Use Case 2: Count all the lines of code in a directory, displaying a progress bar during the counting process
Code:
cloc --progress=1 path/to/directory
Motivation:
When analyzing large directories, users may find it beneficial to see real-time feedback on the progress of cloc
. This ability helps in better time management, allowing the user to estimate how long the analysis will take and verify that the program is working correctly.
Explanation:
--progress=1
: This argument enables a simple progress bar that updates ascloc
processes each file. Useful for providing visual feedback on long-running operations.path/to/directory
: Specifies the directory to analyze.
Example Output:
Counting: 1000 files, 10 files/sec (at 10% done)...
[##################---------------------------] 45%
...
-------------------------------------------------------------------------------
Language files blank comment code
-------------------------------------------------------------------------------
Java 12 250 300 1200
Python 10 100 140 800
-------------------------------------------------------------------------------
TOTAL 22 350 440 2000
-------------------------------------------------------------------------------
Use Case 3: Compare 2 directory structures and count the differences between them
Code:
cloc --diff path/to/directory/one path/to/directory/two
Motivation:
Software projects evolve over time, and differences between versions are crucial for understanding progress, refactoring, or regressions. By comparing two directories, developers can gain insights into which files have been modified, added, or deleted and to what extent, providing clarity on changes made.
Explanation:
--diff
: Tellscloc
to perform a differential analysis between the two specified directories.path/to/directory/one
: The source directory for comparison.path/to/directory/two
: The target directory to compare against the source.
Example Output:
-------------------------------------------------------------------------------
Language files # Added # Removed # Modified
-------------------------------------------------------------------------------
Python 3 50 10 20
JavaScript 2 20 5 10
-------------------------------------------------------------------------------
TOTAL 5 70 15 30
-------------------------------------------------------------------------------
Use Case 4: Ignore files that are ignored by VCS, such as files specified in .gitignore
Code:
cloc --vcs git path/to/directory
Motivation:
To maintain focus on the source code that is actively part of a version-controlled project, developers may wish to exclude files that are ignored by configurations like .gitignore
. This is useful for ensuring that analytical results pertain solely to files that are actually intended to be part of the version-controlled repository.
Explanation:
--vcs git
: This tellscloc
to use the version control system rules specified for Git, such as those in.gitignore
, to exclude certain files and directories from the analysis.path/to/directory
: Specifies the directory to analyze, ignoring files as per VCS settings.
Example Output:
-------------------------------------------------------------------------------
Language files blank comment code
-------------------------------------------------------------------------------
JavaScript 4 70 40 350
CSS 2 20 30 150
HTML 3 30 20 200
-------------------------------------------------------------------------------
TOTAL 9 120 90 700
-------------------------------------------------------------------------------
Use Case 5: Count all the lines of code in a directory, displaying the results for each file instead of each language
Code:
cloc --by-file path/to/directory
Motivation:
For granular analysis of the codebase, it might be helpful to break down the line count on a per-file basis rather than a per-language basis. This can be useful for identifying particularly large or comment-heavy files, or for allocating work and conducting detailed reviews.
Explanation:
--by-file
: Directscloc
to output results for each individual file rather than aggregating them by programming language.path/to/directory
: The directory that contains the files to analyze.
Example Output:
-------------------------------------------------------------------------------
File blank comment code
-------------------------------------------------------------------------------
index.js 20 15 100
style.css 10 10 75
main.py 15 25 120
-------------------------------------------------------------------------------
TOTAL 45 50 295
-------------------------------------------------------------------------------
Conclusion:
The cloc
command-line tool is an excellent resource for software professionals who need to measure and understand their source code’s structure and size. Whether counting lines, comparing directories, or analyzing the impact of a .gitignore
file, cloc
provides flexible options to accommodate various scenarios in software development and maintenance. With these detailed examples and explanations, utilizing cloc
should be straightforward for any user with basic command-line experience.