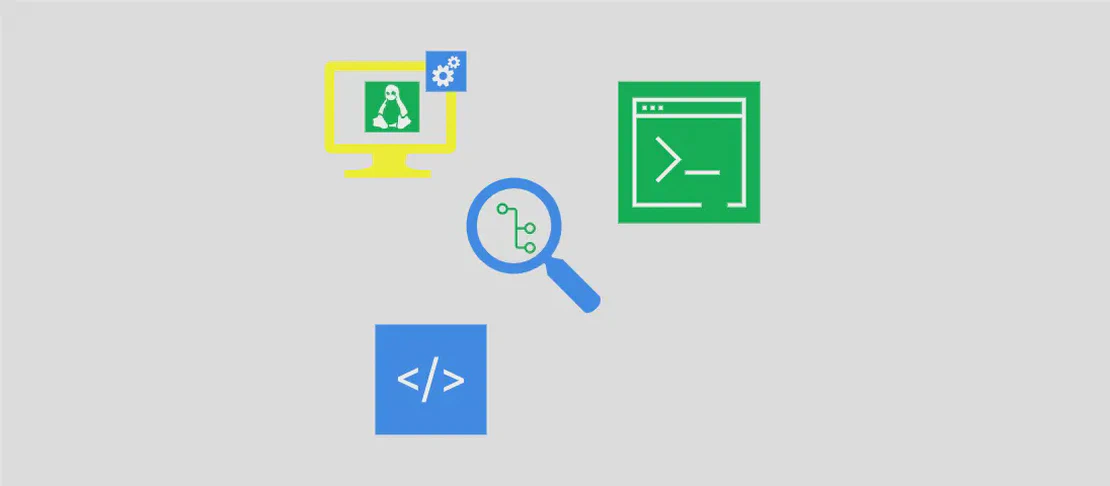
How to Use the Command 'cmake' (with Examples)
CMake is a cross-platform build automation tool designed to streamline the process of managing build systems in complex software projects. It provides a powerful set of features that allow developers to generate native build scripts for different platforms, specify build configurations, and customize installation procedures efficiently.
Generate a build recipe in the current directory with CMakeLists.txt
from a project directory
Code:
cmake path/to/project_directory
Motivation:
This command is ideal for initializing the build process of a project. It reads the CMakeLists.txt
file within the specified project directory and generates a native build system in the current working directory. This foundational step is necessary for setting up the development environment on any supported platform.
Explanation:
cmake
: Initiates the CMake process, a utility to control the compilation process.path/to/project_directory
: Specifies the directory containing theCMakeLists.txt
file, which describes the project configuration.
Example Output:
-- The C compiler identification is GNU 9.3.0
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Configuring done
-- Generating done
-- Build files have been written to: /current/directory
Generate a build recipe, with build type set to Release
with CMake variable
Code:
cmake path/to/project_directory -D CMAKE_BUILD_TYPE=Release
Motivation:
Specifying the build type is crucial when optimizing the software for distribution. A Release
build typically includes optimizations that enhance performance by removing debugging symbols and leveraging compiler optimizations.
Explanation:
cmake
: The command line tool used to generate build files.path/to/project_directory
: Path to the directory containing the necessary configuration files.-D CMAKE_BUILD_TYPE=Release
: Defines the build type variable, allowing CMake to generate a build optimized for release, focusing on performance enhancements.
Example Output:
-- Build type: Release
-- Configuring done
-- Generating done
-- Build files have been written to: /current/directory
Generate a build recipe using generator_name
as the underlying build system
Code:
cmake -G generator_name path/to/project_directory
Motivation:
Selecting a specific build system generator is crucial when targeting different development environments or making use of specific development tools. This command is extremely versatile when developers need to produce build scripts compatible with their chosen build systems like Ninja or Unix Makefiles.
Explanation:
cmake
: The core tool for configuring and generating builds.-G generator_name
: Specifies the generator to use for creating the build system. It allows compatibility with a variety of build systems.path/to/project_directory
: Indicates the location of the source project files.
Example Output:
-- Configuring done
-- Generating done
-- Build files have been written to: /current/directory_using_generator_name
Use a generated recipe in a given directory to build artifacts
Code:
cmake --build path/to/build_directory
Motivation:
Building from a previously generated recipe is crucial to compiling code and producing executable artifacts. This command assembles all the project’s components taken from the described build configuration.
Explanation:
cmake
: The entry point for managing the build process.--build path/to/build_directory
: Directs CMake to utilize the given build directory to produce the project outputs.
Example Output:
Scanning dependencies of target my_application
[ 25%] Building C object CMakeFiles/my_application.dir/file.c.o
[100%] Built target my_application
Install the build artifacts into /usr/local/
and strip debugging symbols
Code:
cmake --install path/to/build_directory --strip
Motivation:
Stripping debugging symbols reduces the size of the build artifacts, which is important in a release environment where storage space and speed are priorities. Installing these stripped binaries directly into the system path ensures seamless execution and accessibility.
Explanation:
cmake
: The command for orchestrating various phases of building and installing.--install path/to/build_directory
: Points to the build directory, from which artifacts will be installed.--strip
: Removes debugging symbols, optimizing the artifacts for deployment.
Example Output:
-- Installing: /usr/local/bin/my_application
Install the build artifacts using the custom prefix for paths
Code:
cmake --install path/to/build_directory --strip --prefix path/to/directory
Motivation:
There are times when customization of the installation location is required, such as within encapsulated environments or when the install location needs to be isolated from the system. This feature offers flexibility by allowing specification of custom paths.
Explanation:
cmake
: Performs functions related to building, testing, and packaging.--install path/to/build_directory
: The directory from which the installation is performed.--strip
: Ensures that debugging symbols are not included in the installed artifacts.--prefix path/to/directory
: Customizing the destination path for installation, which adds flexibility to deployment.
Example Output:
-- Installing: path/to/directory/bin/my_application
Run a custom build target
Code:
cmake --build path/to/build_directory --target target_name
Motivation:
Custom build targets are useful when only certain parts of a project need to be built, such as a library or a test suite. This command allows for precise control over what is built, optimizing development time by focusing only on necessary components.
Explanation:
cmake
: Acts as a driver for build operations.--build path/to/build_directory
: Identifies the build environment.--target target_name
: Specifies a unique target within the build system, enabling specific component builds.
Example Output:
Scanning dependencies of target specific_component
[ 50%] Building C object CMakeFiles/specific_component.dir/custom_code.c.o
[100%] Built target specific_component
Display help, obtain a list of generators
Code:
cmake --help
Motivation:
Understanding available commands, options, and generators is essential for effective use of CMake. This command helps in navigating through various functionalities CMake offers, making it easier for both beginners and experienced developers to find the needed information.
Explanation:
cmake
: The command-line tool for build management.--help
: Instructs CMake to display available options, including an extensive list of generators for build systems across platforms.
Example Output:
Usage
cmake [options] <path-to-source>
cmake --build <dir> [options] [--target <tgt>...]
Available generators are:
Unix Makefiles = Generates standard UNIX makefiles.
Ninja = Generates build.ninja files.
....
Conclusion:
As a versatile and essential tool in modern software development, CMake facilitates cross-platform support, simplifies configuration, and enhances control of the build process. From generating optimized build recipes to customizing installations, each use case illustrates the important features and capabilities that empower developers to efficiently manage their workflows across various platforms and environments.