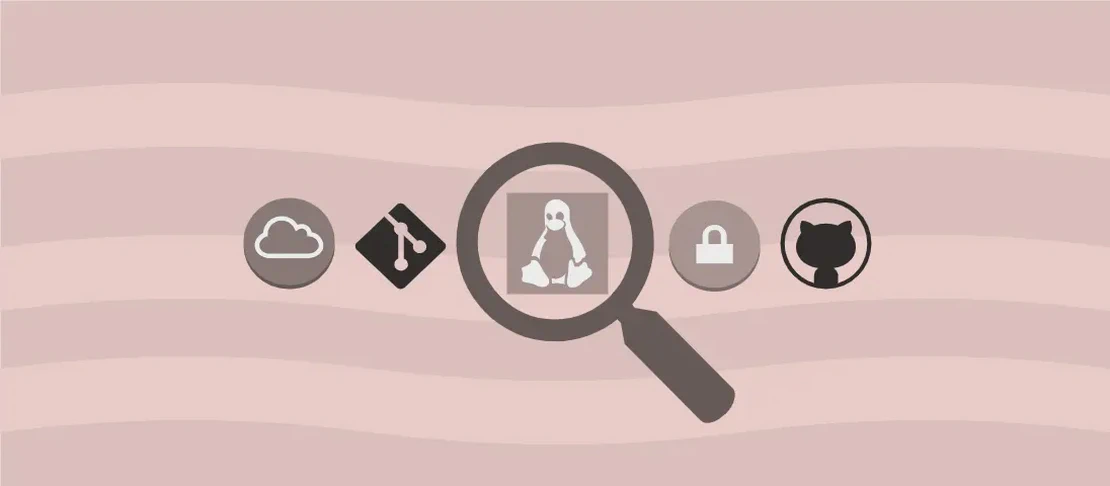
How to Use the 'coffee' Command (with Examples)
The ‘coffee’ command is a versatile tool designed for developers working with CoffeeScript, a little language that compiles into JavaScript. CoffeeScript makes code more readable by offering a cleaner, less verbose syntax, which reduces the chances of errors and improves productivity. The ‘coffee’ command allows users to execute CoffeeScript scripts directly or to compile them into JavaScript files, enabling integration with JavaScript projects. It also features an interactive REPL to experiment with code snippets and file watching capabilities for efficient development workflows.
Use Case 1: Run a CoffeeScript Script
Code:
coffee path/to/file.coffee
Motivation: Running a CoffeeScript script directly is useful for quickly testing or executing your written code without the need to manually compile it into JavaScript. It provides a streamlined workflow during development or debugging.
Explanation:
coffee
: This is the command that initiates the CoffeeScript execution process.path/to/file.coffee
: This argument specifies the path to the CoffeeScript file you want to run. The.coffee
extension is used to denote a CoffeeScript file.
Example Output:
Suppose your CoffeeScript file contains a simple line like console.log 'Hello, CoffeeScript!'
, the output upon running the above command will be:
Hello, CoffeeScript!
Use Case 2: Compile to JavaScript and Save to a File with the Same Name
Code:
coffee --compile path/to/file.coffee
Motivation: Compiling CoffeeScript into JavaScript is necessary when you are ready to deploy your CoffeeScript code within a JavaScript application. This allows you to utilize the JavaScript ecosystem while writing code in a more concise syntax.
Explanation:
coffee
: Initiates the CoffeeScript command-line interface.--compile
: This flag tells the command to compile the CoffeeScript code rather than execute it, transforming.coffee
script into its.js
counterpart.path/to/file.coffee
: Specifies the source file to be compiled.
Example Output:
If the CoffeeScript contains alert 'Compilation Successful!'
, the --compile
option will produce a JavaScript file (file.js
) with equivalent content in JavaScript syntax, like alert('Compilation Successful!');
.
Use Case 3: Compile to JavaScript and Save to a Given Output File
Code:
coffee --compile path/to/file.coffee --output path/to/file.js
Motivation: Specifying an output file path gives you control over where the compiled JavaScript file is saved, allowing for organized directory structures or integration into specific build processes.
Explanation:
coffee
: Starts the command-line interface for CoffeeScript.--compile
: Signals the intention to compile the script into JavaScript.path/to/file.coffee
: Input source file to be compiled.--output path/to/file.js
: Specifies the destination file where the compiled JavaScript code should be stored.
Example Output:
Expect to find the JavaScript code at path/to/file.js
after execution. For instance, console.log 'Custom Output!'
in CoffeeScript would be compiled to console.log('Custom Output!');
in the specified JavaScript file.
Use Case 4: Start a REPL (Interactive Shell)
Code:
coffee --interactive
Motivation: The REPL (Read-Evaluate-Print Loop) mode is perfect for testing snippets of CoffeeScript code in real-time. It allows developers to iterate fast, try out syntax, and see immediate output, reducing the feedback loop during experimentation.
Explanation:
coffee
: Engages the CoffeeScript CLI.--interactive
: Launches the REPL, an interactive shell environment for running CoffeeScript commands line by line.
Example Output: Entering the REPL will prompt something like:
coffee>
There you can type something simple like square = (x) -> x * x
and then call square 2
, which will immediately output 4
.
Use Case 5: Watch Script for Changes and Re-run Script
Code:
coffee --watch path/to/file.coffee
Motivation: Watching a script for changes facilitates a more dynamic and efficient workflow, particularly during development when code is frequently updated. Each change in the file triggers a re-run of the script, allowing you to see the results of the latest code modification instantly.
Explanation:
coffee
: Calls the command to either execute or process scripts.--watch
: Instructs the tool to monitor the specified file for any changes continuously.path/to/file.coffee
: Indicates the specific CoffeeScript file to observe.
Example Output:
On making a change to your CoffeeScript file, such as changing console.log 'Hello'
to console.log 'Hello Again'
, you will see:
Hello Again
It automatically prints this new output upon saving the changes.
Conclusion:
The ‘coffee’ command provides an array of utilities for developers working with CoffeeScript, from executing scripts to compiling and interacting through a REPL. These functionalities enhance productivity and offer a seamless transition between CoffeeScript and JavaScript, making it an essential part of any CoffeeScript developer’s toolkit.