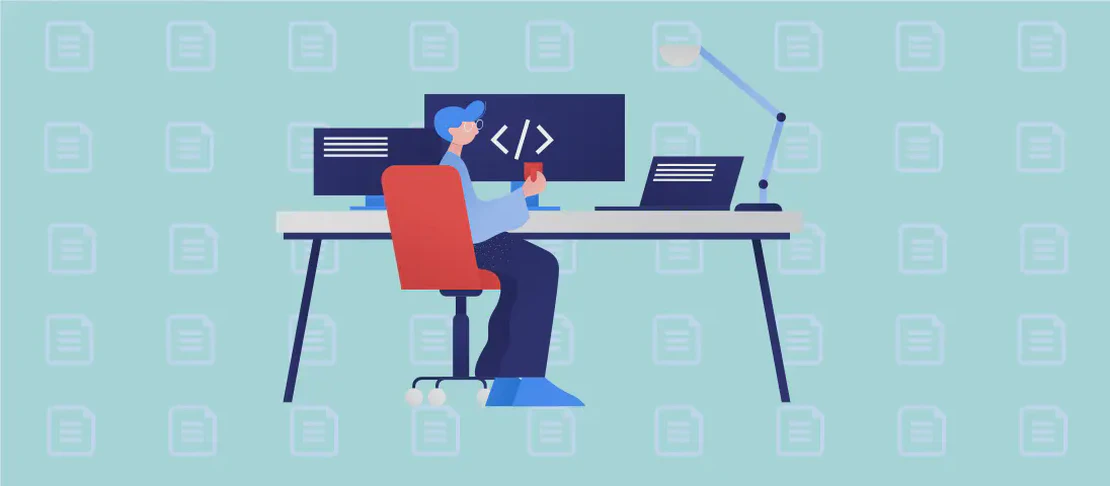
Mastering Composer: A Comprehensive Guide to PHP Dependency Management (with examples)
Composer is a package-based dependency manager for PHP projects that simplifies the process of managing and installing libraries and packages. It has become an essential tool for PHP developers by enabling them to easily declare, install, and update project dependencies. Below, we illustrate various use cases of the Composer command through practical examples.
Interactively Create a Composer.json File
Code:
composer init
Motivation:
Creating a composer.json
file is the first step in any PHP project that requires dependency management. The composer.json
file is a manifest that describes project dependencies, scripts, and other metadata. An interactive setup ensures that developers do not miss essential fields and provides a guided approach to setting up a new project.
Explanation:
composer init
: This command starts an interactive script that asks a series of questions to set up acomposer.json
file. You will input details such as the project name, description, author, minimum stability, and the PHP packages you want to include as dependencies. It’s an introductory and user-friendly method to establish the foundation of your PHP project.
Example Output:
This command will guide you through creating your composer.json config.
Package name (<vendor>/<name>) [user/package]:
...
Add a Package as a Dependency
Code:
composer require user/package
Motivation:
When a new feature or library is needed, adding it to the project via dependencies is necessary. Adding dependencies through composer require
ensures that the composer.json
and composer.lock
files are updated, locking the project to specific package versions for consistency across different environments.
Explanation:
composer require
: This command adds a new package to your project as a dependency. It modifies thecomposer.json
to include the package under the “require” section.user/package
: Replace ‘user/package’ with the actual package name. Composer fetches this package from Packagist, the default PHP package repository, and installs it in your project.
Example Output:
Using version ^1.0 for user/package
./composer.json has been updated
...
Install All Dependencies
Code:
composer install
Motivation:
Once your composer.json
is set up with the desired dependencies, you need to install them to start using them in your project. This use case is particularly relevant for newly cloned projects where the composer.lock
file ensures that all dependencies reflect the exact versions intended by the original setup.
Explanation:
composer install
: The command reads thecomposer.json
file and installs all the listed dependencies. It also checks thecomposer.lock
file to ensure that the installed packages adhere to the locked versions, maintaining version consistency.
Example Output:
Loading composer repositories with package information
Installing dependencies (including require-dev)
Package operations: 5 installs, 0 updates, 0 removals
- Installing vendor/package (version)...
Uninstall a Package
Code:
composer remove user/package
Motivation: Project requirements change, and sometimes dependencies are no longer necessary. Removing unnecessary packages can reduce project complexity, file size, and potential vulnerabilities.
Explanation:
composer remove
: This command deletes a package from your project and updates thecomposer.json
andcomposer.lock
files by removing the respective entry.user/package
: The specific package name to be removed from your project.
Example Output:
Dependency "user/package" is not used in any licenses.
./composer.json has been updated
Loading composer repositories with package information
...
Update All Dependencies
Code:
composer update
Motivation: Libraries are updated regularly with improvements, bug fixes, and new features. Keeping your dependencies up to date is crucial to benefit from these improvements and to ensure security vulnerabilities are patched.
Explanation:
composer update
: The command updates all the project dependencies to the latest versions permitted bycomposer.json
. It also updates thecomposer.lock
file to reflect these changes, ensuring that future installations will use the new version set.
Example Output:
Loading composer repositories with package information
Updating dependencies (including require-dev)
Dependency operations: 10 installs, 45 updates, 0 removals
...
Update Only Composer.lock
Code:
composer update --lock
Motivation:
When you modify the composer.json
manually, such as changing version constraints, you may want to update the composer.lock
without affecting the package installations. This ensures that composer.lock
matches composer.json
exactly without pulling in new versions unnecessarily.
Explanation:
composer update
: This updates thecomposer.lock
.--lock
: Argument used to specify that only the lock file should be updated, not the actual installed dependencies.
Example Output:
Lock file operations: 0 installs, 0 updates, 0 removals
Writing lock file
Investigate Dependency Conflicts
Code:
composer why-not user/package
Motivation: Sometimes a package may not be installed due to version conflicts with other dependencies or requirements not being met. Understanding why a package cannot be installed is critical for resolving these issues.
Explanation:
composer why-not
: This command diagnoses why a specific package cannot be installed, providing a conflict tree that can highlight which requirements are not being met.user/package
: The package you are having issues with will help in determining any conflicts.
Example Output:
There is no installed package depending on "user/package"
or All packages are compatible with "user/package" no need for action
Update Composer to its Latest Version
Code:
composer self-update
Motivation: Keeping Composer itself updated is necessary to ensure that you have the latest features and security patches. New composer releases often include performance improvements and resolve known issues.
Explanation:
composer self-update
: This command updates composer to the latest stable released version, ensuring you have the latest capabilities and improvements.
Example Output:
Updating to version 2.x.x (stable channel).
Conclusion:
Composer is a robust and powerful tool for PHP developers, simplifying the management of project dependencies. By understanding and leveraging its capabilities, developers can ensure that their projects are well-maintained, secure, and aligned with best practices in software development. From initiating a project to maintaining updated dependencies, Composer is an indispensable part of the modern PHP development ecosystem.