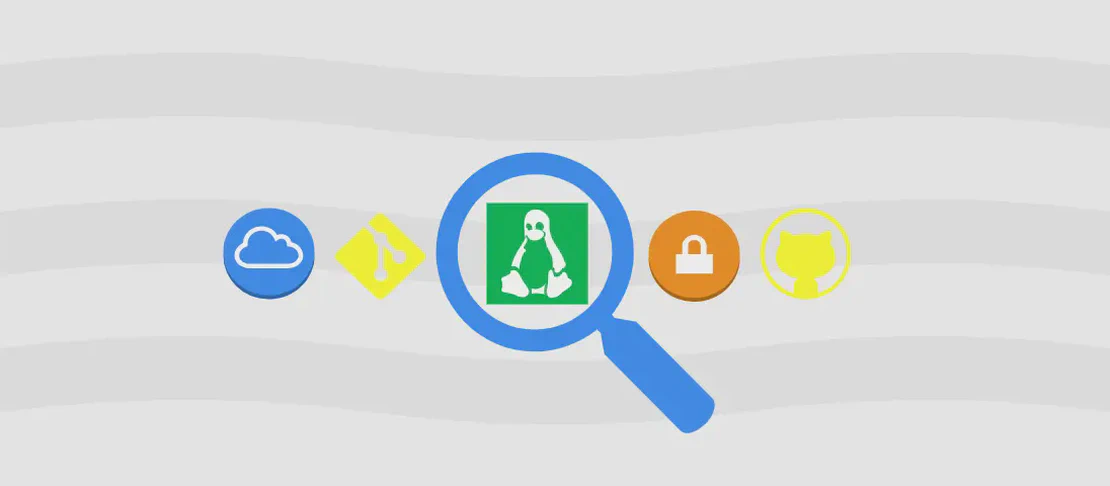
How to use the command 'conda' (with examples)
Conda is an open-source package management system and environment management system that allows users to install, run, and update software packages and their dependencies in a reliable and reproducible manner. It is designed for creating, managing, and deploying multiple environment setups with potentially conflicting package requirements. This flexibility is particularly useful for data scientists, developers, and anyone working with various software stacks. Conda can handle software installations involving any programming language, making it highly versatile and widely used across different fields.
Use case 1: Create a new environment, installing named packages into it
Code:
conda create --name environment_name python=3.9 matplotlib
Motivation:
Creating a new environment allows developers to manage dependencies specific to a project without interfering with other projects. In this example, a virtual environment is created to work on a Python project that requires Python 3.9 and the Matplotlib library for data visualization. This setup isolates the specific versions needed, ensuring compatibility and reducing potential version conflicts.
Explanation:
conda create
: The command to create a new environment.--name environment_name
: Specifies the name of the new environment, making it easy to identify.python=3.9
: Specifies that Python version 3.9 should be installed within this environment.matplotlib
: Installs the Matplotlib package, a popular library for creating static, animated, and interactive visualizations in Python.
Example Output:
Collecting package metadata (current_repodata.json): done
Solving environment: done
## Package Plan ##
environment location: /path/to/envs/environment_name
added / updated specs:
- matplotlib
- python=3.9
The following NEW packages will be INSTALLED:
# Package installation details ...
Proceed ([y]/n)? y
Downloading and Extracting Packages
# Package details ...
done
Use case 2: List all environments
Code:
conda info --envs
Motivation:
Listing all environments is crucial for managing and organizing your development workspace. This command helps you quickly see all active and available environments, allowing for better management and switching between different project setups.
Explanation:
conda info
: Provides information about conda’s current status.--envs
: Lists all the environments managed by conda on your system.
Example Output:
# conda environments:
#
base * /path/to/anaconda3
environment_name /path/to/envs/environment_name
another_env /path/to/envs/another_env
Use case 3: Load an environment
Code:
conda activate environment_name
Motivation:
Activating an environment is necessary to use the specific packages and Python version installed in it. For instance, if you are ready to work on a project that is stored in the environment called environment_name
, this command ensures that your terminal session uses the exact setup you need.
Explanation:
conda activate
: Activates a specified conda environment, modifying your terminal’s environment variables to include the dependencies of the selected environment.environment_name
: The name of the environment you wish to load or activate.
Example Output:
(environment_name) user@hostname:~$
Use case 4: Unload an environment
Code:
conda deactivate
Motivation:
Deactivating an environment is essential when you’re done working on a particular project. This command restores your terminal session to the base environment of conda or the system’s default settings, helping prevent accidental changes in an active project setup.
Explanation:
conda deactivate
: Reverts the terminal back to the default environment or previous settings upon deactivation of a conda environment, removing the active environment context.
Example Output:
user@hostname:~$
Use case 5: Delete an environment (remove all packages)
Code:
conda remove --name environment_name --all
Motivation:
When an environment is no longer needed, deleting it helps free up system resources. This command completely removes the environment and all its packages, ensuring no residual files are left behind, which can help streamline system maintenance.
Explanation:
conda remove
: Command used to remove an environment or packages.--name environment_name
: Specifies which environment to remove by name.--all
: Designates that the entire environment, with all its associated packages, should be removed.
Example Output:
Remove all packages in environment /path/to/envs/environment_name:
removed package details …
Proceed ([y]/n)? y
Environment removed.
Use case 6: Install packages into the current environment
Code:
conda install python=3.4 numpy
Motivation:
This command is beneficial when you need to add or update packages within your current working environment to suit changing project requirements. For example, including NumPy, a fundamental package for numerical computation, alongside Python in your setup ensures you have the necessary tools for scientific calculations.
Explanation:
conda install
: Command to add new packages to an existing environment.python=3.4
: Specifies that Python version 3.4 should be installed, or verified as the current version.numpy
: Installs the NumPy package, frequently used for handling large arrays and matrices.
Example Output:
Collecting package metadata (current_repodata.json): done
Solving environment: done
## Package Plan ##
environment location: /path/to/current/env
added / updated specs:
- python=3.4
- numpy
Proceed ([y]/n)? y
Downloading and Extracting Packages
# Package details ...
done
Use case 7: List currently installed packages in current environment
Code:
conda list
Motivation:
Listing packages in the current environment is necessary for auditing and evaluating the software setup. This practice helps users understand what packages are available, their versions, and possibly identify if further changes or updates are required.
Explanation:
conda list
: Displays all the packages and their respective versions currently installed in the active conda environment.
Example Output:
# packages in environment at /path/to/envs/environment_name:
#
# Name Version Build Channel
matplotlib 3.4.3 py39h06a4308_0
numpy 1.21.2 py39h20f2e39_0
python 3.9.7 h12debd9_1
# Additional package details ...
Use case 8: Delete unused packages and caches
Code:
conda clean --all
Motivation:
Regularly cleaning up unused packages and caches helps to recover disk space and maintain system cleanliness. This command removes unnecessary package caches, log files, and unused tarballs, keeping your system neat and efficient.
Explanation:
conda clean
: Command used to remove unnecessary files and directories.--all
: Cleans all non-essential files related to conda environments and package installations, including cache files, logs, and unused package versions.
Example Output:
Cache location: /path/to/anaconda3/pkgs
Will remove the following packages:
# List of unused packages...
Proceed ([y]/n)? y
Cache cleared.
Conclusion:
Conda offers a robust and versatile means to manage environments and packages for a wide array of projects, allowing developers to maintain focused and isolated workspaces. Understanding and using the various ‘conda’ commands efficiently can streamline your workflow, enhance productivity, and reduce system bloat, especially when handling multiple project environments or evolving software requirements. With these examples, users can easily adapt ‘conda’ to suit their personal or professional needs.