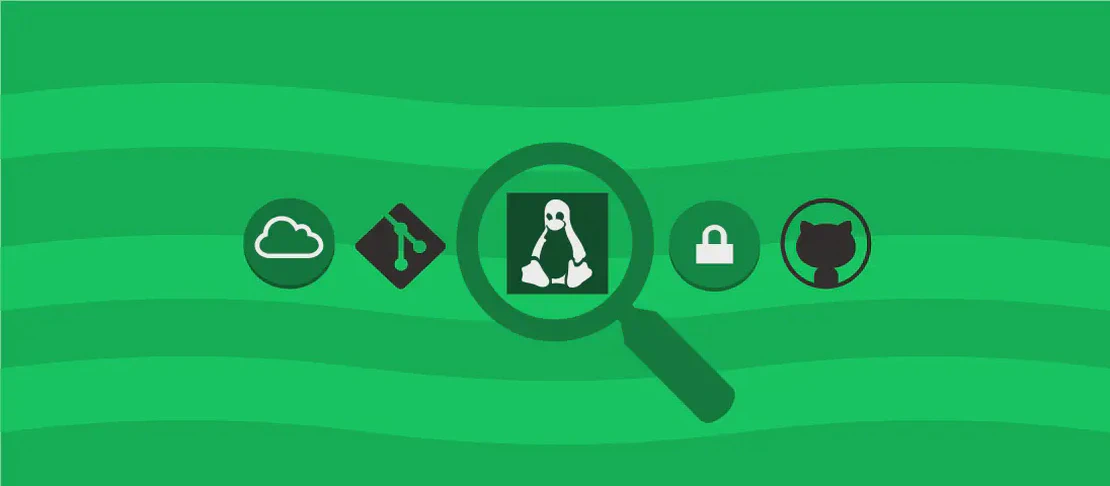
Exploring the `coproc` Command in Bash (with examples)
The coproc
command in Bash enables users to create interactive asynchronous subshells. This built-in command can be invaluable for executing tasks concurrently, handling multiple inputs and outputs, and improving efficiency in shell scripting workflows. By using coprocesses, scripts can manage operations without waiting for previous commands to finish, allowing for parallel computation and more dynamic interactivity.
Use case 1: Run a subshell asynchronously
Code:
coproc { command1; command2; ...; }
Motivation:
Running a subshell asynchronously allows you to execute multiple commands without having to wait for each one to complete before the next starts. This can be particularly beneficial when you want to perform operations such as data processing or file handling in parallel, improving the speed and efficiency of your scripts.
Explanation:
coproc
: The Bash built-in command that initializes a coprocess.{ command1; command2; ...; }
: A block of commands, enclosed in braces, that are executed asynchronously in a child process.
Example output:
When you run a coprocess containing echo "Hello"
followed by echo "World"
, the output might not appear immediately since the commands are run in the background:
Hello
World
Use case 2: Create a coprocess with a specific name
Code:
coproc name { command1; command2; ...; }
Motivation:
Naming a coprocess can simplify managing and referencing multiple concurrent processes. This is especially useful in complex scripts where coordinating specific tasks is crucial.
Explanation:
coproc name
: Initiates a coprocess with the given identifiername
.{ command1; command2; ...; }
: A sequence of commands to execute within the coprocess.
Example output:
If you create a coprocess named myproc
for ls
and date
, you would later refer to myproc
using this handle to interact with its input or output.
Use case 3: Write to a specific coprocess stdin
Code:
echo "input" >&"${{name}}[1]"
Motivation:
This functionality is crucial when a script needs to send data to a running coprocess, such as sending commands to a process like bc
, which performs calculations.
Explanation:
echo "input"
: Command to output text.>&"${{name}}[1]"
: Redirects the output of the echo command to the stdin of the coprocess identified byname
.
Example output:
Sending “list” command to a coprocess handling a network service might cause the coprocess to list connected clients.
Use case 4: Read from a specific coprocess stdout
Code:
read variable <&"${{name}}[0]}"
Motivation:
Reading output from a coprocess allows a script to capture and process the results of asynchronously running commands.
Explanation:
read variable
: Reads data into the specified variable.<&"${{name}}[0]}"
: Redirects the stdout of the coprocess identified byname
to theread
command.
Example output:
When integrated with a coprocess running bc
, the script reads calculated results into a variable for further use or display.
Use case 5: Create a coprocess which repeatedly reads stdin
and runs some commands on the input
Code:
coproc name { while read line; do command1; command2; ...; done }
Motivation:
This use case is advantageous for handling streaming input data. The coprocess reads lines continuously and processes each one with the specified commands, ideal for log parsing or data transformation in real time.
Explanation:
coproc name
: Starts a coprocess with the identifiername
.{ while read line; do command1; command2; ...; done }
: A loop that reads each line fromstdin
and executes a set of commands.
Example output:
If command1
prints the length of a line, inputting “Hello World” results in 11
, showing how the data is processed per line received.
Use case 6: Create and use a coprocess running bc
Code:
coproc BC { bc --mathlib; }; echo "1/3" >&"${BC[1]}"; read output <&"${BC[0]}"; echo "$output"
Motivation:
This usage demonstrates integrating coproc
with bc
, the basic calculator, to evaluate expressions asynchronously.
Explanation:
coproc BC { bc --mathlib; }
: Starts a coprocess runningbc
with its math library.echo "1/3" >&"${BC[1]}"
: Sends the expression to be evaluated tobc
’s stdin.read output <&"${BC[0]}"
: Reads the result frombc
’s stdout into the variableoutput
.echo "$output"
: Displays the result.
Example output:
Executing this script calculates 1/3
using bc
, with the expected output of
.3333333333
Conclusion:
The coproc
command in Bash provides a powerful mechanism for handling asynchronous tasks within scripts. Leveraging named coprocesses, and efficient input and output handling, it can significantly enhance the performance and complexity of shell-based workflows. Whether running calculations, transforming data, or managing network interactions, coproc
offers a versatile toolset for advanced shell scripting.