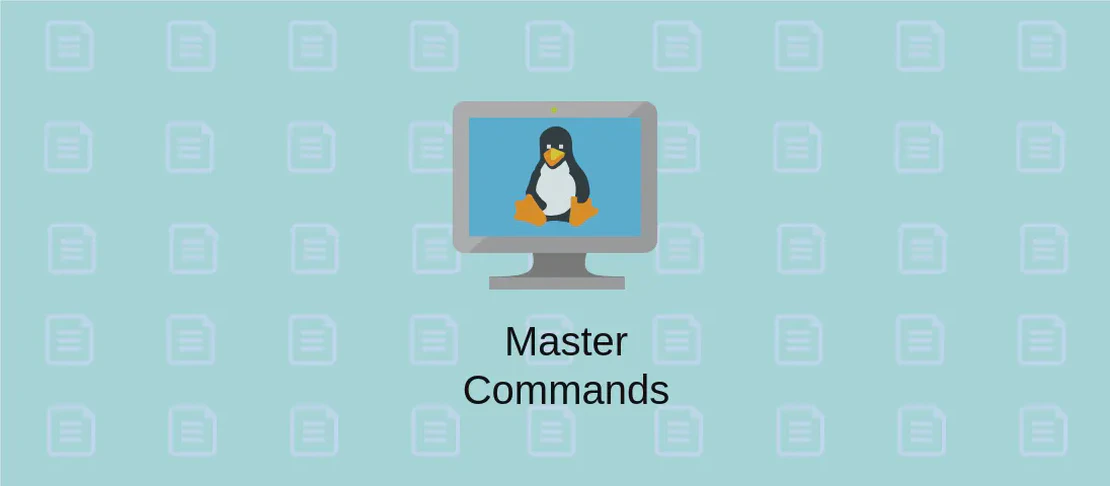
How to Use the Bash 'coproc' Command (with Examples)
- Linux
- December 17, 2024
The coproc
command in Bash is a powerful feature that allows the creation of asynchronous subshells. It enables users to initiate coprocesses, which are essentially background processes, and interact with them in a bidirectional manner. This makes coproc
very useful for scenarios where input and output need to be managed asynchronously without blocking the main shell execution.
Run a Subshell Asynchronously
Code:
coproc { command1; command2; ...; }
Motivation:
In scenarios where different tasks need to be executed simultaneously without holding up the main thread of execution, running a subshell asynchronously can be invaluable. It allows different processes to be executed independently, ensuring that they do not block others that may depend on different resources.
Explanation:
coproc
: Indicates the initiation of a coprocess.{ command1; command2; ...; }
: This is the command group that will run inside the coprocess. It allows for multiple commands to execute asynchronously in the background.
Example Output:
When coproc
is used with commands like sleep 5; echo "Finished sleeping"
, the main shell continues executing other tasks while the coprocess does its work, eventually outputting “Finished sleeping” after the delay.
Create a Coprocess with a Specific Name
Code:
coproc name { command1; command2; ...; }
Motivation:
Naming a coprocess can be particularly useful when managing multiple asynchronous tasks, as it allows you to easily identify and interact with each coprocess individually. This is important in complex scripts where clarity and organization are crucial.
Explanation:
coproc name
: Initiates a coprocess and assigns a specific name to it.name
: The chosen identifier for the coprocess, helping to reference its file descriptors later.{ command1; command2; ...; }
: The set of commands to be executed by the coprocess.
Example Output:
This might output labels like [1] 54321
indicating the background job and process ID, allowing you to channel input/output streams efficiently as per the coprocess named name
.
Write to a Specific Coprocess stdin
Code:
echo "input" >&"${{{name}}[1]}"
Motivation:
Sending input to a coprocess is a fundamental operation when you want to provide specific commands or data for processing. It allows the coprocess to function with dynamic input based on real-time user actions or other automated processes.
Explanation:
echo "input"
: Sends the string “input” to standard output.>&
: Redirects this output to another file descriptor."${{{name}}[1]}"
: The file descriptor for the coprocess’stdin
, specified by its name.
Example Output:
If the coprocess is running a mathematical calculator program, echoing “2+2” will input it into the program, expecting to see a computation output as a result.
Read from a Specific Coprocess stdout
Code:
read variable <&"${{{name}}[0]}"
Motivation:
Retrieving output from a coprocess is necessary when the execution results need to be used in further decision-making processes or output formatting. This capability is critical in scripting where output needs to be captured and potentially transformed or stored.
Explanation:
read variable
: Reads input from the standard input.<&
: Redirects input from a specified file descriptor."${{{name}}[0]}"
: The file descriptor forstdout
of the named coprocess.
Example Output:
When the coprocess outputs calculation results, such as “4”, this value can be stored in a variable for subsequent usage or logging.
Create a Coprocess which Repeatedly Reads stdin
and Runs Some Commands on the Input
Code:
coproc name { while read line; do command1; command2; ...; done }
Motivation:
Having a coprocess that actively listens for input to process allows for persistent interactive sessions or repeating tasks. This usage is crucial for servers, listeners, or anytime daemon-like behavior in scripts is desired.
Explanation:
coproc name
: Initializes a named coprocess.while read line
: Continuously reads lines from input.do command1; command2;
: Executes a series of commands on the read input.
Example Output:
If this coprocess is set up to convert input text to uppercase, inputs such as “hello” would output “HELLO”.
Create a Coprocess which Repeatedly Reads stdin
, Runs a Pipeline on the Input, and Writes the Output to stdout
Code:
coproc name { while read line; do echo "$line" | command1 | command2 | cat /dev/fd/0; done }
Motivation:
Pipelining processes together with coprocess functionality allows complex transformations and multi-step computations on inputs. This is useful in data processing or real-time data transformations where multiple operations need to be performed sequentially.
Explanation:
coproc name
: Initiates the coprocess with a name.while read line
: The coprocess continually accepts input.echo "$line"
: Converts input to a command-friendly format.|
: Passes output from one command to the next in the pipeline.
Example Output:
Inputs passing through filters/commands might experience transformations, such as text conversion and sorting, showing all processed outputs back through the pipeline to stdout
.
Create and Use a Coprocess Running bc
Code:
coproc BC { bc --mathlib; }; echo "1/3" >&"${BC[1]}"; read output <&"${BC[0]}"; echo "$output"
Motivation:
Using a coprocess to interface with bc
(an arbitrary precision calculator) offers a programmatic way to perform complex mathematical operations within scripts, facilitating calculated dynamic configurations or decision-making processes.
Explanation:
coproc BC { bc --mathlib; }
: Initiates a coprocess runningbc
with its math library.echo "1/3" >&"${BC[1]}"
: Sends the expression “1/3” tobc
.read output <&"${BC[0]}"
: Captures the output frombc
.echo "$output"
: Displays the result.
Example Output:
This script outputs a precise result such as “0.3333333333” demonstrating bc
’s capabilities in doing mathematical calculations.
Conclusion:
Bash’s coproc
command is versatile and allows powerful management of asynchronous processes. With examples covering various scenarios from simple command execution to complex input/output operations and data processing, understanding the use of coproc
rules can significantly enhance script efficiency and flexibility.