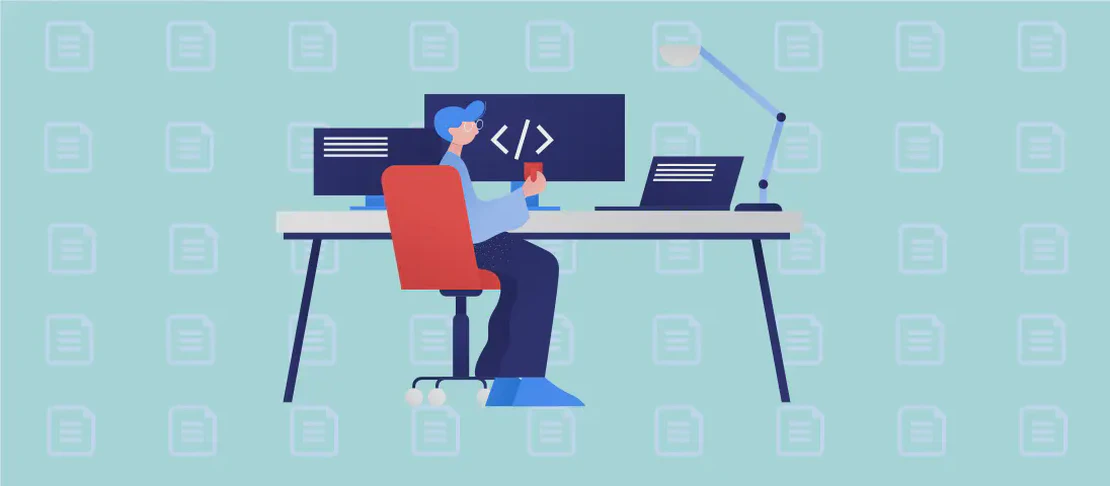
Mastering Apache Cordova for Mobile App Development (with examples)
Apache Cordova is a popular open-source framework that enables developers to create mobile applications using HTML, CSS, and JavaScript. By wrapping these web technologies into native applications for different mobile platforms, developers can write their application code once and deploy it across various platforms such as iOS, Android, and Windows. This versatility not only accelerates development but also reduces the costs associated with maintaining separate codebases for each platform. With Cordova, you can leverage existing web development skills to enter the mobile app market effectively.
Use case 1: Create a Cordova project
Code:
cordova create path/to/directory package project_name
Motivation:
Creating a new Cordova project is the first step in building a cross-platform mobile application. This command sets up the basic project structure and necessary files needed for you to start adding functionality and aesthetics to your app. Initiating a project through Cordova enables you to efficiently manage the unique requirements of each mobile platform while also maintaining shared resources.
Explanation:
path/to/directory
: This specifies the directory path where the project will be created. Be sure to choose a path that is easy to access and well-organized within your development environment.package
: This represents the reverse domain-style identifier for your app, such ascom.example.myapp
. It ensures that your app has a unique identifier within its namespace.project_name
: This is the display name for your app, which will appear on device home screens.
Example Output:
Creating a new cordova project.
Use case 2: Display the current workspace status
Code:
cordova info
Motivation:
Understanding the current state of your Cordova project is crucial, especially as projects grow in complexity. The cordova info
command provides a comprehensive summary of project details, including installed plugins, platforms, and other configuration settings. This informs you of the project’s current setup and any necessary changes or updates that may be required.
Explanation:
No additional arguments are needed with this command. Simply running cordova info
will gather and display information pertinent to the project’s current configuration.
Example Output:
{
"platforms": ["android", "ios"],
"plugins": ["cordova-plugin-camera"],
"projectName": "MyCordovaApp"
}
Use case 3: Add a Cordova platform
Code:
cordova platform add platform
Motivation:
To extend your app’s reach, you’ll want to deploy to multiple mobile platforms. The cordova platform add
command enables you to add support for a specific platform such as Android or iOS. This command is essential for starting the process of adapting your web application code to run on different mobile operating systems.
Explanation:
platform
: This argument specifies the type of platform you want to add. Typical examples areandroid
,ios
, orwindows
. This informs Cordova to set up the necessary infrastructure related to the chosen platform within your project.
Example Output:
Adding android project...
Use case 4: Remove a Cordova platform
Code:
cordova platform remove platform
Motivation:
In some scenarios, you may decide to discontinue support for a particular platform based on strategic or technical considerations. Using cordova platform remove
helps maintain a cleaner project structure by removing files and configurations associated with the platform you no longer wish to support.
Explanation:
platform
: This is the platform you intend to remove, such asandroid
,ios
, etc. Removing a platform deletes its files and settings from your Cordova project, which helps declutter unnecessary components.
Example Output:
Removing platform android from project...
Use case 5: Add a Cordova plugin
Code:
cordova plugin add pluginid
Motivation:
Plugins are a core feature of Cordova, allowing you to access native device features such as the camera, geolocation, and more. Adding a plugin expands your app’s functionality, connecting your web-based app with hardware-specific resources in a seamless manner. The cordova plugin add
command simplifies integrating these plugins into your project.
Explanation:
pluginid
: This is the unique identifier of the plugin you want to add, likecordova-plugin-camera
. It tells Cordova which specific plugin to download and integrate into the project.
Example Output:
Installing "cordova-plugin-camera" for android
Use case 6: Remove a Cordova plugin
Code:
cordova plugin remove pluginid
Motivation:
Over time, you might need to remove a plugin from your project because it is no longer required, makes your app overly complex, or has been replaced by another solution. The cordova plugin remove
command ensures that the plugin and its dependencies are cleaned from your project, preventing any unnecessary consumption of resources.
Explanation:
pluginid
: This specifies the plugin you wish to remove, such ascordova-plugin-camera
. Removing a plugin helps maintain optimal project performance and management by eliminating unused code.
Example Output:
Removing "cordova-plugin-camera"
Conclusion:
Apache Cordova offers a tremendous boost in developing cross-platform mobile applications by allowing you to repurpose your web development skills. Through the examples above, you gained insights into starting a Cordova project, manipulating platforms and plugins, and understanding your project’s current state—all essential for maintaining performant and versatile mobile applications. With Cordova, you are well-equipped to deliver applications with broad reach and consistent user experiences.