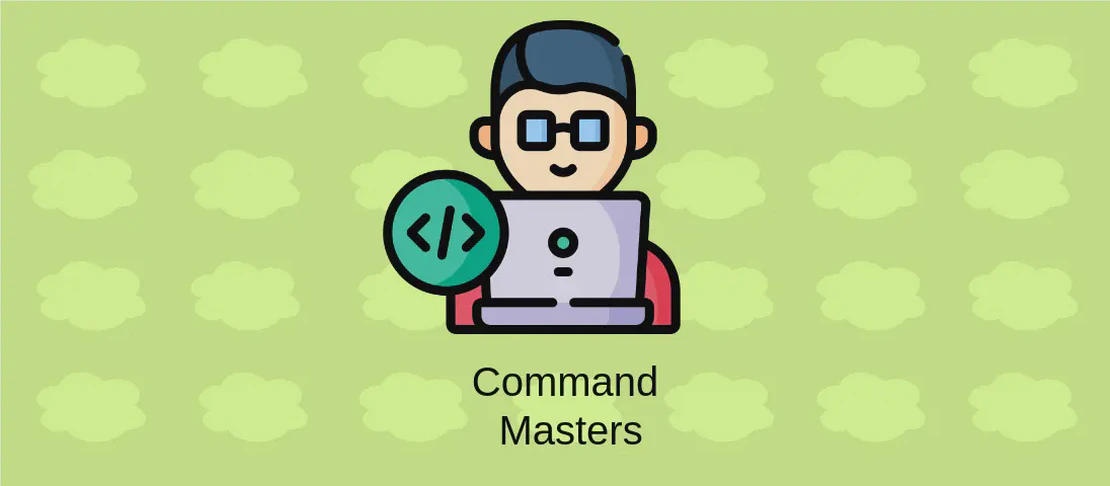
How to Use the Command `cp` (with Examples)
The cp
command in Linux is a versatile utility used to copy files and directories from one location to another. It is part of the GNU Core Utilities and is often employed in shell scripts and general command-line operations for managing files. With various options and parameters, cp
can handle everything from simple file copies to more complex directory operations while preserving file attributes and structure as needed.
Use Case 1: Copy a File to Another Location
Code:
cp path/to/source_file.ext path/to/target_file.ext
Motivation: Sometimes you need to create a direct copy of a file and place it in a different directory, perhaps for backup, configuration purposes, or simply to have the same file available in another location for accessibility. This simple command helps in directly duplicating a file from its original directory to the specified target, making it easier to manage versions or backups quickly.
Explanation:
cp
: Invokes the copy command.path/to/source_file.ext
: Specifies the path and name of the file you want to copy.path/to/target_file.ext
: Defines the destination path where the new copy of the file will be placed, inclusive of the new file name.
Example Output: Assuming source_file.ext
is a text file containing “Hello World”, after execution, target_file.ext
in the target directory will have the same content.
Use Case 2: Copy a File into Another Directory, Keeping the Filename
Code:
cp path/to/source_file.ext path/to/target_parent_directory
Motivation: This use case is helpful when you want to keep the original filename intact but move the file to a different directory. For instance, moving configuration files from a development folder to a production environment without any renaming.
Explanation:
cp
: The copy command.path/to/source_file.ext
: Locates the original file that needs to be copied.path/to/target_parent_directory
: The directory where the source file will be copied, maintaining its original filename.
Example Output: If the source file named source_file.ext
contains “Configuration Settings”, it will appear in the target_parent_directory
with the same name and content.
Use Case 3: Recursively Copy a Directory’s Contents to Another Location
Code:
cp -R path/to/source_directory path/to/target_directory
Motivation: In scenarios where you need to duplicate an entire directory with its contents, such as in backing up project files or migrating directory structures to different servers, a recursive copy ensures all files within the directory, including subdirectories, are copied over.
Explanation:
cp
: The command to copy files.-R
: Stands for recursive, instructingcp
to copy directories and their contents recursively.path/to/source_directory
: The directory whose contents and structure you want to replicate.path/to/target_directory
: The destination directory where the source directory’s contents will be placed.
Example Output: If source_directory
contains files and subfolders, after execution, target_directory
will reflect the same structure and files.
Use Case 4: Copy a Directory Recursively, in Verbose Mode
Code:
cp -vR path/to/source_directory path/to/target_directory
Motivation:
When you need clarity on which files and directories are being copied, perhaps for debugging or logging purposes, the verbose option provides real-time feedback on what is happening as the cp
command executes.
Explanation:
cp
: Copy command.-v
: Verbose option, which helps debug by printing the names of files/directories as they are processed.-R
: Enables recursive copying of directories and their inner contents.path/to/source_directory
: The source directory to be duplicated.path/to/target_directory
: The destination where the copy will be placed.
Example Output: As files are copied, the terminal window will display messages indicating each file and directory during the copy process, such as “source_directory/file.txt -> target_directory/file.txt”.
Use Case 5: Copy Multiple Files at Once to a Directory
Code:
cp -t path/to/destination_directory path/to/file1 path/to/file2 ...
Motivation: When handling multiple specific files that need to be collected and moved to a single location, such as compiling a set of documents for project submission, this command streamlines the task by moving them all in one go.
Explanation:
cp
: The command to initialize copy.-t path/to/destination_directory
: Specifies target directory upfront where files will be copied.path/to/file1 path/to/file2 ...
: Lists multiple files to be copied, helping streamline the command input when dealing with several files.
Example Output: If running for file1
, file2
, etc., after command execution, all listed files will appear in the destination_directory
.
Use Case 6: Copy Text Files to Another Location, in Interactive Mode
Code:
cp -i *.txt path/to/target_directory
Motivation: Interactive copying is essential when there’s a risk of overwriting files in the target directory. This option prompts for confirmation, ensuring you don’t unintentionally overwrite files, which is particularly useful in sensitive environments like production systems.
Explanation:
cp
: Copies files.-i
: Interactive mode, which asks for user confirmation if a copy will overwrite an existing file.*.txt
: Indicates all text files in the current directory.path/to/target_directory
: The location to copy the files into.
Example Output: Each .txt
file being copied will trigger a prompt like “overwrite ‘file.txt’? y/n”, allowing for safe copying control.
Use Case 7: Follow Symbolic Links Before Copying
Code:
cp -L link path/to/target_directory
Motivation: Copying symbolic links without resolving them can lead to broken links in the destination. This option ensures that the files or directories the links point to are copied, avoiding potential issues in environments where actual file content is necessary.
Explanation:
cp
: Command to initiate copying.-L
: Follows symbolic links to the actual files or directories during copying.link
: The symbolic link or location of the linked file/directory.path/to/target_directory
: Destination directory for the copied content.
Example Output: If link
points to a file example.txt
, after this command, example.txt
rather than the link itself is copied to the target_directory
.
Use Case 8: Use the First Argument as the Destination Directory
Code:
cp -t path/to/target_directory path/to/file_or_directory1 path/to/file_or_directory2 ...
Motivation:
This setup is favorable for command pipelines, such as an output from xargs
, that include cp
to dynamically copy generated file paths, ensuring streamlined and precise directory targeting.
Explanation:
cp
: The core command to copy.-t path/to/target_directory
: Sets the target directory for all specified files/directories.path/to/file_or_directory1 path/to/file_or_directory2 ...
: A list of multiple paths, including files or directories, that need copying to the target location.
Example Output: After execution, all specified files and directories will reside in the target_directory
.
Conclusion
The cp
command, with its various options and flexibility, provides an essential tool for file management in Linux environments. Whether managing single files, directories, or symbolic links, understanding and utilizing the appropriate cp
command use cases can enhance efficiency and safety in file operations. From ensuring backup precision to managing project configurations, these use cases demonstrate cp
’s significant and versatile role in daily command-line operations.